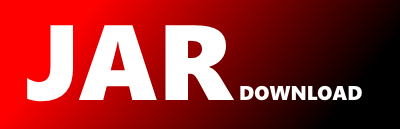
io.relayr.java.RelayrJavaSdk Maven / Gradle / Ivy
package io.relayr.java;
import javax.inject.Inject;
import io.relayr.java.api.AccountsApi;
import io.relayr.java.api.DeviceApi;
import io.relayr.java.api.DeviceModelsApi;
import io.relayr.java.api.GroupsApi;
import io.relayr.java.api.services.AggregatedDataService;
import io.relayr.java.api.services.NotificationService;
import io.relayr.java.api.ProjectsApi;
import io.relayr.java.api.PublishersApi;
import io.relayr.java.api.RelayrApi;
import io.relayr.java.api.RuleTemplateApi;
import io.relayr.java.api.UserApi;
import io.relayr.java.api.services.RawDataService;
import io.relayr.java.api.helpers.AggregatedDataHelper;
import io.relayr.java.api.helpers.RawDataHelper;
import io.relayr.java.model.Device;
import io.relayr.java.model.Transmitter;
import io.relayr.java.model.User;
import io.relayr.java.model.account.Account;
import io.relayr.java.model.groups.Group;
import io.relayr.java.model.models.DeviceModel;
import io.relayr.java.storage.DeviceModelCache;
import io.relayr.java.websocket.WebSocketClient;
import retrofit.RestAdapter;
import rx.Observable;
/**
* The RelayrJavaSdk Class serves as the access point to all endpoints in the Android SDK.
* It includes basic calls such as user login validation and can also call the handlers of the other classes.
* For easy start, after logging in, obtain {@link User} by calling {@link #getUser()}.
* This is main object in Relayr SDK that can be used to fetch users {@link Device},
* {@link Transmitter}, {@link Group} and {@link Account} objects.
* Every mentioned object has it's own interaction methods so direct usage of APIs is not necessary.
* However it's still possible to obtain any of the desired API handlers through appropriate method:
*
* - {@link #getUserApi()}
* - {@link #getRelayrApi()}
* - {@link #getDeviceApi()}
* - {@link #getGroupsApi()}
* - {@link #getAccountsApi()}
* - {@link #getPublishersApi()}
* - {@link #getProjectsApi()}
* - {@link #getRuleTemplateApi()}
* - {@link #getAggregatedDataService()} ()} or using {@link AggregatedDataHelper}
* - {@link #getRawDataService()} ()} ()} or using {@link RawDataHelper}
* - {@link #getDeviceModelsApi()} or using cache {@link #getDeviceModelsCache()}
*
* For other details check methods JavaDoc
*/
public class RelayrJavaSdk {
@Inject static UserApi userApi;
@Inject static DeviceApi deviceApi;
@Inject static RelayrApi relayrApi;
@Inject static GroupsApi groupsApi;
@Inject static AccountsApi accountsApi;
@Inject static DeviceModelsApi deviceModelsApi;
@Inject static DeviceModelCache deviceModelsCache;
@Inject static WebSocketClient webSocketClient;
@Inject static PublishersApi publishersApi;
@Inject static ProjectsApi projectsApi;
@Inject static RuleTemplateApi ruleTemplateApi;
@Inject static RawDataService rawDataService;
@Inject static AggregatedDataService aggregatedDataService;
@Inject static NotificationService notificationService;
/** Initializes the SDK. */
public static class Builder {
private boolean inMock = false;
private boolean inProduction = true;
private boolean cacheModels = false;
private String token;
private RestAdapter.LogLevel level;
private String userAgent;
private String mainApi;
private String mqttApi;
public Builder() {}
/**
* LEGACY
* Initializes the SDK in Mock Mode. False by default.
* In this mode, mock reading values are generated
* Used for testing purposes, without the need of a WunderBar or an internet connection.
*/
public Builder inMockMode(boolean mockMode) {
this.inMock = mockMode;
return this;
}
/**
* Initializes the SDK in production or development mode.
* Use each environment with corresponding API key. True by default.
*/
public Builder useProduction(boolean production) {
this.inProduction = production;
return this;
}
/**
* Sets Log level for all API calls.
* Defaults: in production {@link retrofit.RestAdapter.LogLevel#NONE}
* in development {@link retrofit.RestAdapter.LogLevel#BASIC}
*/
public Builder setLogLevel(RestAdapter.LogLevel level) {
if (level == null) throw new NullPointerException("Log level can not be NULL!");
this.level = level;
return this;
}
/**
* Sets token retrieved from @see link
* Token is necessary to be able to make any of the API calls.
*/
public Builder setToken(String token) {
if (token == null) throw new NullPointerException("Token can not be NULL!");
this.token = token;
return this;
}
/** If true it will cache all device models in the background. By default it's false. */
public Builder cacheModels(boolean cache) {
this.cacheModels = cache;
return this;
}
/** Define specific user agent for your app */
public Builder setUserAgent(String userAgent) {
this.userAgent = userAgent;
return this;
}
/** EXPERIMENTAL - Set specific API urls. If not used, default relayr APIs are used. */
public Builder setApiUrls(String mainApi, String mqttApi) {
if (mainApi == null || mqttApi == null)
throw new NullPointerException("Api endpoint can not be NULL!");
this.mainApi = mainApi;
this.mqttApi = mqttApi;
return this;
}
/** Initializes SDK with inserted parameters */
public void build() {
if (mainApi == null)
RelayrJavaApp.init(token, inMock, inProduction, cacheModels, level, userAgent);
else
RelayrJavaApp.init(token, inMock, inProduction, cacheModels, level, userAgent, mainApi, mqttApi);
}
}
/**
* Returns log level set when initializing the SDK.
* Possible levels:
* {@link retrofit.RestAdapter.LogLevel#NONE},
* {@link retrofit.RestAdapter.LogLevel#BASIC},
* {@link retrofit.RestAdapter.LogLevel#HEADERS},
* {@link retrofit.RestAdapter.LogLevel#HEADERS_AND_ARGS},
* {@link retrofit.RestAdapter.LogLevel#FULL},
* @return current Log level
*/
public static RestAdapter.LogLevel getLogLevel() {
return RelayrJavaApp.getLogLevel();
}
/** Returns true if production environment servers are used. False if development environment is selected. */
public static boolean isProductionEnvironment() {
return RelayrJavaApp.isProduction();
}
/**
* @return user object.
* This is main object in Relayr SDK that can be used to fetch user {@link Device},
* {@link Transmitter}, {@link Group} and {@link Account}.
* Any of the mentioned objects has it's own special methods so direct usage of APIs is not necessary but it's still possible
*/
public static Observable getUser() {
return userApi.getUserInfo();
}
/**
* @return the version String
*/
public static String getVersion() {
return "1.4.0";
}
/**
* @return the handler of the Relayr API.
* Used as an access point to class {@link RelayrApi}
*/
public static RelayrApi getRelayrApi() {
return relayrApi;
}
/**
* @return the handler of the Accounts API. Use to add third party accounts to the relayr user.
* Used as an access point to class {@link AccountsApi}
*/
public static AccountsApi getAccountsApi() {
return accountsApi;
}
/**
* @return the handler of the Device API.
* Used as an access point to class {@link DeviceApi}
*/
public static DeviceApi getDeviceApi() {
return deviceApi;
}
/**
* @return the handler of the Groups API.
* Used as an access point to class {@link GroupsApi}
*/
public static GroupsApi getGroupsApi() {
return groupsApi;
}
/**
* @return the handler of the relayr User API.
* Use to get user data, user transmitters, devices, accounts and groups
* Used as an access point to class {@link UserApi}
*/
public static UserApi getUserApi() {
return userApi;
}
/**
* @return the handler of the relayr AggregatedDataService API.
* Used as an access point to class {@link AggregatedDataService}
* {@link Device#getAggregatedDataHelper()} can be used to fetch aggregated history data for each device.
*/
public static AggregatedDataService getAggregatedDataService() {
return aggregatedDataService;
}
/**
* @return the handler of the relayr RawDataService API.
* Used as an access point to class {@link RawDataService}
* {@link Device#getRawDataHelper()} can be used to fetch raw history data for each device.
*/
public static RawDataService getRawDataService() {
return rawDataService;
}
/**
* @return the handler of the relayr NotificationService API.
* Used as an access point to class {@link NotificationService}
*/
public static NotificationService getNotificationService() {
return notificationService;
}
/**
* @return the handler of the relayr RuleTemplate API.
* Used as an access point to class {@link RuleTemplateApi}
* Provides an API for the creation of Rule Engine Rules on the base of pre made templates.
*/
public static RuleTemplateApi getRuleTemplateApi() {
return ruleTemplateApi;
}
/**
* Use this class to subscribe to live data from device, namely {@link io.relayr.java.model.action.Reading},
* {@link io.relayr.java.model.action.Command} and
* {@link io.relayr.java.model.action.Configuration}
* @return the handler of the WebSocketClient
*/
public static WebSocketClient getWebSocketClient() {
return webSocketClient;
}
/**
* @return the handler of the relayr DeviceModels API. Returns new device models.
* Used as an access point to class {@link DeviceModelsApi}
*/
public static DeviceModelsApi getDeviceModelsApi() {
return deviceModelsApi;
}
/**
* @return cached {@link DeviceModel} objects.
* Cache will be populated with models from {@link Device#modelId} when device is fetched.
* Use instead of {@link #getDeviceModelsApi()}
*/
public static DeviceModelCache getDeviceModelsCache() {
return deviceModelsCache;
}
/** @return the handler of the relayr {@link PublishersApi}. */
public static PublishersApi getPublishersApi() {
return publishersApi;
}
/** @return the handler of the relayr {@link ProjectsApi}. */
public static ProjectsApi getProjectsApi() {
return projectsApi;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy