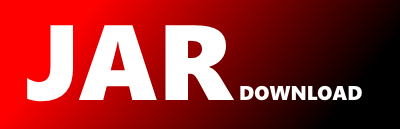
io.relayr.java.api.ApiModule$$ModuleAdapter Maven / Gradle / Ivy
// Code generated by dagger-compiler. Do not edit.
package io.relayr.java.api;
import com.squareup.okhttp.OkHttpClient;
import dagger.internal.Binding;
import dagger.internal.BindingsGroup;
import dagger.internal.Linker;
import dagger.internal.ModuleAdapter;
import dagger.internal.ProvidesBinding;
import io.relayr.java.api.services.AggregatedDataService;
import io.relayr.java.api.services.NotificationService;
import io.relayr.java.api.services.RawDataService;
import java.lang.Class;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.util.Set;
import retrofit.Endpoint;
import retrofit.RestAdapter;
import retrofit.client.Client;
/**
* A manager of modules and provides adapters allowing for proper linking and
* instance provision of types served by {@code @dagger.Provides} methods.
*/
public final class ApiModule$$ModuleAdapter extends ModuleAdapter {
private static final String[] INJECTS = { };
private static final Class>[] STATIC_INJECTIONS = { };
private static final Class>[] INCLUDES = { };
public ApiModule$$ModuleAdapter() {
super(ApiModule.class, INJECTS, STATIC_INJECTIONS, false /*overrides*/, INCLUDES, false /*complete*/, true /*library*/);
}
@Override
public ApiModule newModule() {
return new ApiModule();
}
/**
* Used internally obtain dependency information, such as for cyclical
* graph detection.
*/
@Override
public void getBindings(BindingsGroup bindings, ApiModule module) {
bindings.contributeProvidesBinding("@javax.inject.Named(value=api)/retrofit.Endpoint", new ApiEndpointProvidesAdapter(module));
bindings.contributeProvidesBinding("com.squareup.okhttp.OkHttpClient", new ProvideOkHttpClientProvidesAdapter(module));
bindings.contributeProvidesBinding("retrofit.client.Client", new ProvideClientProvidesAdapter(module));
bindings.contributeProvidesBinding("@javax.inject.Named(value=api)/retrofit.RestAdapter", new ProvideApiRestAdapterProvidesAdapter(module));
bindings.contributeProvidesBinding("@javax.inject.Named(value=oauth)/retrofit.RestAdapter", new ProvideOauthRestAdapterProvidesAdapter(module));
bindings.contributeProvidesBinding("@javax.inject.Named(value=models)/retrofit.RestAdapter", new ProvideModelsRestAdapterProvidesAdapter(module));
bindings.contributeProvidesBinding("@javax.inject.Named(value=history)/retrofit.RestAdapter", new ProvideHistoryRestAdapterProvidesAdapter(module));
bindings.contributeProvidesBinding("io.relayr.java.api.OauthApi", new ProvideOauthApiProvidesAdapter(module));
bindings.contributeProvidesBinding("io.relayr.java.api.ChannelApi", new ProvideChannelApiProvidesAdapter(module));
bindings.contributeProvidesBinding("io.relayr.java.api.CloudApi", new ProvideCloudApiProvidesAdapter(module));
bindings.contributeProvidesBinding("io.relayr.java.api.RelayrApi", new ProvideRelayrApiProvidesAdapter(module));
bindings.contributeProvidesBinding("io.relayr.java.api.DeviceApi", new ProvideDeviceApiProvidesAdapter(module));
bindings.contributeProvidesBinding("io.relayr.java.api.AccountsApi", new ProvideAccountsApiProvidesAdapter(module));
bindings.contributeProvidesBinding("io.relayr.java.api.GroupsApi", new ProvideGroupsApiProvidesAdapter(module));
bindings.contributeProvidesBinding("io.relayr.java.api.UserApi", new ProvideUserApiProvidesAdapter(module));
bindings.contributeProvidesBinding("io.relayr.java.api.DeviceModelsApi", new ProvideDeviceModelsApiProvidesAdapter(module));
bindings.contributeProvidesBinding("io.relayr.java.api.ProjectsApi", new ProvideProjectsApiProvidesAdapter(module));
bindings.contributeProvidesBinding("io.relayr.java.api.PublishersApi", new ProvidePublishersApiProvidesAdapter(module));
bindings.contributeProvidesBinding("io.relayr.java.api.RuleTemplateApi", new ProvideRulesApiProvidesAdapter(module));
bindings.contributeProvidesBinding("io.relayr.java.api.services.RawDataService", new ProvideRawDataApiProvidesAdapter(module));
bindings.contributeProvidesBinding("io.relayr.java.api.services.AggregatedDataService", new ProvideAggDataApiProvidesAdapter(module));
bindings.contributeProvidesBinding("io.relayr.java.api.services.NotificationService", new ProvideNotificationsApiProvidesAdapter(module));
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ApiEndpointProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
public ApiEndpointProvidesAdapter(ApiModule module) {
super("@javax.inject.Named(value=api)/retrofit.Endpoint", IS_SINGLETON, "io.relayr.java.api.ApiModule", "apiEndpoint");
this.module = module;
setLibrary(true);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public Endpoint get() {
return module.apiEndpoint();
}
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ProvideOkHttpClientProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
public ProvideOkHttpClientProvidesAdapter(ApiModule module) {
super("com.squareup.okhttp.OkHttpClient", IS_SINGLETON, "io.relayr.java.api.ApiModule", "provideOkHttpClient");
this.module = module;
setLibrary(true);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public OkHttpClient get() {
return module.provideOkHttpClient();
}
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Owning the dependency links between {@code Client} and its
* dependencies.
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ProvideClientProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
private Binding client;
public ProvideClientProvidesAdapter(ApiModule module) {
super("retrofit.client.Client", IS_SINGLETON, "io.relayr.java.api.ApiModule", "provideClient");
this.module = module;
setLibrary(true);
}
/**
* Used internally to link bindings/providers together at run time
* according to their dependency graph.
*/
@Override
@SuppressWarnings("unchecked")
public void attach(Linker linker) {
client = (Binding) linker.requestBinding("com.squareup.okhttp.OkHttpClient", ApiModule.class, getClass().getClassLoader());
}
/**
* Used internally obtain dependency information, such as for cyclical
* graph detection.
*/
@Override
public void getDependencies(Set> getBindings, Set> injectMembersBindings) {
getBindings.add(client);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public Client get() {
return module.provideClient(client.get());
}
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Owning the dependency links between {@code RestAdapter} and its
* dependencies.
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ProvideApiRestAdapterProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
private Binding endpoint;
private Binding client;
public ProvideApiRestAdapterProvidesAdapter(ApiModule module) {
super("@javax.inject.Named(value=api)/retrofit.RestAdapter", IS_SINGLETON, "io.relayr.java.api.ApiModule", "provideApiRestAdapter");
this.module = module;
setLibrary(true);
}
/**
* Used internally to link bindings/providers together at run time
* according to their dependency graph.
*/
@Override
@SuppressWarnings("unchecked")
public void attach(Linker linker) {
endpoint = (Binding) linker.requestBinding("@javax.inject.Named(value=api)/retrofit.Endpoint", ApiModule.class, getClass().getClassLoader());
client = (Binding) linker.requestBinding("retrofit.client.Client", ApiModule.class, getClass().getClassLoader());
}
/**
* Used internally obtain dependency information, such as for cyclical
* graph detection.
*/
@Override
public void getDependencies(Set> getBindings, Set> injectMembersBindings) {
getBindings.add(endpoint);
getBindings.add(client);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public RestAdapter get() {
return module.provideApiRestAdapter(endpoint.get(), client.get());
}
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Owning the dependency links between {@code RestAdapter} and its
* dependencies.
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ProvideOauthRestAdapterProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
private Binding endpoint;
private Binding client;
public ProvideOauthRestAdapterProvidesAdapter(ApiModule module) {
super("@javax.inject.Named(value=oauth)/retrofit.RestAdapter", IS_SINGLETON, "io.relayr.java.api.ApiModule", "provideOauthRestAdapter");
this.module = module;
setLibrary(true);
}
/**
* Used internally to link bindings/providers together at run time
* according to their dependency graph.
*/
@Override
@SuppressWarnings("unchecked")
public void attach(Linker linker) {
endpoint = (Binding) linker.requestBinding("@javax.inject.Named(value=api)/retrofit.Endpoint", ApiModule.class, getClass().getClassLoader());
client = (Binding) linker.requestBinding("retrofit.client.Client", ApiModule.class, getClass().getClassLoader());
}
/**
* Used internally obtain dependency information, such as for cyclical
* graph detection.
*/
@Override
public void getDependencies(Set> getBindings, Set> injectMembersBindings) {
getBindings.add(endpoint);
getBindings.add(client);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public RestAdapter get() {
return module.provideOauthRestAdapter(endpoint.get(), client.get());
}
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Owning the dependency links between {@code RestAdapter} and its
* dependencies.
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ProvideModelsRestAdapterProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
private Binding endpoint;
private Binding client;
public ProvideModelsRestAdapterProvidesAdapter(ApiModule module) {
super("@javax.inject.Named(value=models)/retrofit.RestAdapter", IS_SINGLETON, "io.relayr.java.api.ApiModule", "provideModelsRestAdapter");
this.module = module;
setLibrary(true);
}
/**
* Used internally to link bindings/providers together at run time
* according to their dependency graph.
*/
@Override
@SuppressWarnings("unchecked")
public void attach(Linker linker) {
endpoint = (Binding) linker.requestBinding("@javax.inject.Named(value=api)/retrofit.Endpoint", ApiModule.class, getClass().getClassLoader());
client = (Binding) linker.requestBinding("retrofit.client.Client", ApiModule.class, getClass().getClassLoader());
}
/**
* Used internally obtain dependency information, such as for cyclical
* graph detection.
*/
@Override
public void getDependencies(Set> getBindings, Set> injectMembersBindings) {
getBindings.add(endpoint);
getBindings.add(client);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public RestAdapter get() {
return module.provideModelsRestAdapter(endpoint.get(), client.get());
}
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Owning the dependency links between {@code RestAdapter} and its
* dependencies.
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ProvideHistoryRestAdapterProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
private Binding endpoint;
private Binding client;
public ProvideHistoryRestAdapterProvidesAdapter(ApiModule module) {
super("@javax.inject.Named(value=history)/retrofit.RestAdapter", IS_SINGLETON, "io.relayr.java.api.ApiModule", "provideHistoryRestAdapter");
this.module = module;
setLibrary(true);
}
/**
* Used internally to link bindings/providers together at run time
* according to their dependency graph.
*/
@Override
@SuppressWarnings("unchecked")
public void attach(Linker linker) {
endpoint = (Binding) linker.requestBinding("@javax.inject.Named(value=history)/retrofit.Endpoint", ApiModule.class, getClass().getClassLoader());
client = (Binding) linker.requestBinding("retrofit.client.Client", ApiModule.class, getClass().getClassLoader());
}
/**
* Used internally obtain dependency information, such as for cyclical
* graph detection.
*/
@Override
public void getDependencies(Set> getBindings, Set> injectMembersBindings) {
getBindings.add(endpoint);
getBindings.add(client);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public RestAdapter get() {
return module.provideHistoryRestAdapter(endpoint.get(), client.get());
}
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Owning the dependency links between {@code OauthApi} and its
* dependencies.
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ProvideOauthApiProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
private Binding adapter;
public ProvideOauthApiProvidesAdapter(ApiModule module) {
super("io.relayr.java.api.OauthApi", IS_SINGLETON, "io.relayr.java.api.ApiModule", "provideOauthApi");
this.module = module;
setLibrary(true);
}
/**
* Used internally to link bindings/providers together at run time
* according to their dependency graph.
*/
@Override
@SuppressWarnings("unchecked")
public void attach(Linker linker) {
adapter = (Binding) linker.requestBinding("@javax.inject.Named(value=oauth)/retrofit.RestAdapter", ApiModule.class, getClass().getClassLoader());
}
/**
* Used internally obtain dependency information, such as for cyclical
* graph detection.
*/
@Override
public void getDependencies(Set> getBindings, Set> injectMembersBindings) {
getBindings.add(adapter);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public OauthApi get() {
return module.provideOauthApi(adapter.get());
}
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Owning the dependency links between {@code ChannelApi} and its
* dependencies.
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ProvideChannelApiProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
private Binding adapter;
public ProvideChannelApiProvidesAdapter(ApiModule module) {
super("io.relayr.java.api.ChannelApi", IS_SINGLETON, "io.relayr.java.api.ApiModule", "provideChannelApi");
this.module = module;
setLibrary(true);
}
/**
* Used internally to link bindings/providers together at run time
* according to their dependency graph.
*/
@Override
@SuppressWarnings("unchecked")
public void attach(Linker linker) {
adapter = (Binding) linker.requestBinding("@javax.inject.Named(value=api)/retrofit.RestAdapter", ApiModule.class, getClass().getClassLoader());
}
/**
* Used internally obtain dependency information, such as for cyclical
* graph detection.
*/
@Override
public void getDependencies(Set> getBindings, Set> injectMembersBindings) {
getBindings.add(adapter);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public ChannelApi get() {
return module.provideChannelApi(adapter.get());
}
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Owning the dependency links between {@code CloudApi} and its
* dependencies.
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ProvideCloudApiProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
private Binding adapter;
public ProvideCloudApiProvidesAdapter(ApiModule module) {
super("io.relayr.java.api.CloudApi", IS_SINGLETON, "io.relayr.java.api.ApiModule", "provideCloudApi");
this.module = module;
setLibrary(true);
}
/**
* Used internally to link bindings/providers together at run time
* according to their dependency graph.
*/
@Override
@SuppressWarnings("unchecked")
public void attach(Linker linker) {
adapter = (Binding) linker.requestBinding("@javax.inject.Named(value=api)/retrofit.RestAdapter", ApiModule.class, getClass().getClassLoader());
}
/**
* Used internally obtain dependency information, such as for cyclical
* graph detection.
*/
@Override
public void getDependencies(Set> getBindings, Set> injectMembersBindings) {
getBindings.add(adapter);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public CloudApi get() {
return module.provideCloudApi(adapter.get());
}
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Owning the dependency links between {@code RelayrApi} and its
* dependencies.
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ProvideRelayrApiProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
private Binding adapter;
public ProvideRelayrApiProvidesAdapter(ApiModule module) {
super("io.relayr.java.api.RelayrApi", IS_SINGLETON, "io.relayr.java.api.ApiModule", "provideRelayrApi");
this.module = module;
setLibrary(true);
}
/**
* Used internally to link bindings/providers together at run time
* according to their dependency graph.
*/
@Override
@SuppressWarnings("unchecked")
public void attach(Linker linker) {
adapter = (Binding) linker.requestBinding("@javax.inject.Named(value=api)/retrofit.RestAdapter", ApiModule.class, getClass().getClassLoader());
}
/**
* Used internally obtain dependency information, such as for cyclical
* graph detection.
*/
@Override
public void getDependencies(Set> getBindings, Set> injectMembersBindings) {
getBindings.add(adapter);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public RelayrApi get() {
return module.provideRelayrApi(adapter.get());
}
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Owning the dependency links between {@code DeviceApi} and its
* dependencies.
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ProvideDeviceApiProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
private Binding adapter;
public ProvideDeviceApiProvidesAdapter(ApiModule module) {
super("io.relayr.java.api.DeviceApi", IS_SINGLETON, "io.relayr.java.api.ApiModule", "provideDeviceApi");
this.module = module;
setLibrary(true);
}
/**
* Used internally to link bindings/providers together at run time
* according to their dependency graph.
*/
@Override
@SuppressWarnings("unchecked")
public void attach(Linker linker) {
adapter = (Binding) linker.requestBinding("@javax.inject.Named(value=api)/retrofit.RestAdapter", ApiModule.class, getClass().getClassLoader());
}
/**
* Used internally obtain dependency information, such as for cyclical
* graph detection.
*/
@Override
public void getDependencies(Set> getBindings, Set> injectMembersBindings) {
getBindings.add(adapter);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public DeviceApi get() {
return module.provideDeviceApi(adapter.get());
}
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Owning the dependency links between {@code AccountsApi} and its
* dependencies.
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ProvideAccountsApiProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
private Binding adapter;
public ProvideAccountsApiProvidesAdapter(ApiModule module) {
super("io.relayr.java.api.AccountsApi", IS_SINGLETON, "io.relayr.java.api.ApiModule", "provideAccountsApi");
this.module = module;
setLibrary(true);
}
/**
* Used internally to link bindings/providers together at run time
* according to their dependency graph.
*/
@Override
@SuppressWarnings("unchecked")
public void attach(Linker linker) {
adapter = (Binding) linker.requestBinding("@javax.inject.Named(value=api)/retrofit.RestAdapter", ApiModule.class, getClass().getClassLoader());
}
/**
* Used internally obtain dependency information, such as for cyclical
* graph detection.
*/
@Override
public void getDependencies(Set> getBindings, Set> injectMembersBindings) {
getBindings.add(adapter);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public AccountsApi get() {
return module.provideAccountsApi(adapter.get());
}
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Owning the dependency links between {@code GroupsApi} and its
* dependencies.
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ProvideGroupsApiProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
private Binding adapter;
public ProvideGroupsApiProvidesAdapter(ApiModule module) {
super("io.relayr.java.api.GroupsApi", IS_SINGLETON, "io.relayr.java.api.ApiModule", "provideGroupsApi");
this.module = module;
setLibrary(true);
}
/**
* Used internally to link bindings/providers together at run time
* according to their dependency graph.
*/
@Override
@SuppressWarnings("unchecked")
public void attach(Linker linker) {
adapter = (Binding) linker.requestBinding("@javax.inject.Named(value=api)/retrofit.RestAdapter", ApiModule.class, getClass().getClassLoader());
}
/**
* Used internally obtain dependency information, such as for cyclical
* graph detection.
*/
@Override
public void getDependencies(Set> getBindings, Set> injectMembersBindings) {
getBindings.add(adapter);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public GroupsApi get() {
return module.provideGroupsApi(adapter.get());
}
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Owning the dependency links between {@code UserApi} and its
* dependencies.
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ProvideUserApiProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
private Binding adapter;
public ProvideUserApiProvidesAdapter(ApiModule module) {
super("io.relayr.java.api.UserApi", IS_SINGLETON, "io.relayr.java.api.ApiModule", "provideUserApi");
this.module = module;
setLibrary(true);
}
/**
* Used internally to link bindings/providers together at run time
* according to their dependency graph.
*/
@Override
@SuppressWarnings("unchecked")
public void attach(Linker linker) {
adapter = (Binding) linker.requestBinding("@javax.inject.Named(value=api)/retrofit.RestAdapter", ApiModule.class, getClass().getClassLoader());
}
/**
* Used internally obtain dependency information, such as for cyclical
* graph detection.
*/
@Override
public void getDependencies(Set> getBindings, Set> injectMembersBindings) {
getBindings.add(adapter);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public UserApi get() {
return module.provideUserApi(adapter.get());
}
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Owning the dependency links between {@code DeviceModelsApi} and its
* dependencies.
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ProvideDeviceModelsApiProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
private Binding adapter;
public ProvideDeviceModelsApiProvidesAdapter(ApiModule module) {
super("io.relayr.java.api.DeviceModelsApi", IS_SINGLETON, "io.relayr.java.api.ApiModule", "provideDeviceModelsApi");
this.module = module;
setLibrary(true);
}
/**
* Used internally to link bindings/providers together at run time
* according to their dependency graph.
*/
@Override
@SuppressWarnings("unchecked")
public void attach(Linker linker) {
adapter = (Binding) linker.requestBinding("@javax.inject.Named(value=models)/retrofit.RestAdapter", ApiModule.class, getClass().getClassLoader());
}
/**
* Used internally obtain dependency information, such as for cyclical
* graph detection.
*/
@Override
public void getDependencies(Set> getBindings, Set> injectMembersBindings) {
getBindings.add(adapter);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public DeviceModelsApi get() {
return module.provideDeviceModelsApi(adapter.get());
}
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Owning the dependency links between {@code ProjectsApi} and its
* dependencies.
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ProvideProjectsApiProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
private Binding adapter;
public ProvideProjectsApiProvidesAdapter(ApiModule module) {
super("io.relayr.java.api.ProjectsApi", IS_SINGLETON, "io.relayr.java.api.ApiModule", "provideProjectsApi");
this.module = module;
setLibrary(true);
}
/**
* Used internally to link bindings/providers together at run time
* according to their dependency graph.
*/
@Override
@SuppressWarnings("unchecked")
public void attach(Linker linker) {
adapter = (Binding) linker.requestBinding("@javax.inject.Named(value=api)/retrofit.RestAdapter", ApiModule.class, getClass().getClassLoader());
}
/**
* Used internally obtain dependency information, such as for cyclical
* graph detection.
*/
@Override
public void getDependencies(Set> getBindings, Set> injectMembersBindings) {
getBindings.add(adapter);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public ProjectsApi get() {
return module.provideProjectsApi(adapter.get());
}
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Owning the dependency links between {@code PublishersApi} and its
* dependencies.
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ProvidePublishersApiProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
private Binding adapter;
public ProvidePublishersApiProvidesAdapter(ApiModule module) {
super("io.relayr.java.api.PublishersApi", IS_SINGLETON, "io.relayr.java.api.ApiModule", "providePublishersApi");
this.module = module;
setLibrary(true);
}
/**
* Used internally to link bindings/providers together at run time
* according to their dependency graph.
*/
@Override
@SuppressWarnings("unchecked")
public void attach(Linker linker) {
adapter = (Binding) linker.requestBinding("@javax.inject.Named(value=api)/retrofit.RestAdapter", ApiModule.class, getClass().getClassLoader());
}
/**
* Used internally obtain dependency information, such as for cyclical
* graph detection.
*/
@Override
public void getDependencies(Set> getBindings, Set> injectMembersBindings) {
getBindings.add(adapter);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public PublishersApi get() {
return module.providePublishersApi(adapter.get());
}
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Owning the dependency links between {@code RuleTemplateApi} and its
* dependencies.
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ProvideRulesApiProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
private Binding adapter;
public ProvideRulesApiProvidesAdapter(ApiModule module) {
super("io.relayr.java.api.RuleTemplateApi", IS_SINGLETON, "io.relayr.java.api.ApiModule", "provideRulesApi");
this.module = module;
setLibrary(true);
}
/**
* Used internally to link bindings/providers together at run time
* according to their dependency graph.
*/
@Override
@SuppressWarnings("unchecked")
public void attach(Linker linker) {
adapter = (Binding) linker.requestBinding("@javax.inject.Named(value=api)/retrofit.RestAdapter", ApiModule.class, getClass().getClassLoader());
}
/**
* Used internally obtain dependency information, such as for cyclical
* graph detection.
*/
@Override
public void getDependencies(Set> getBindings, Set> injectMembersBindings) {
getBindings.add(adapter);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public RuleTemplateApi get() {
return module.provideRulesApi(adapter.get());
}
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Owning the dependency links between {@code RawDataService} and its
* dependencies.
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ProvideRawDataApiProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
private Binding adapter;
public ProvideRawDataApiProvidesAdapter(ApiModule module) {
super("io.relayr.java.api.services.RawDataService", IS_SINGLETON, "io.relayr.java.api.ApiModule", "provideRawDataApi");
this.module = module;
setLibrary(true);
}
/**
* Used internally to link bindings/providers together at run time
* according to their dependency graph.
*/
@Override
@SuppressWarnings("unchecked")
public void attach(Linker linker) {
adapter = (Binding) linker.requestBinding("@javax.inject.Named(value=api)/retrofit.RestAdapter", ApiModule.class, getClass().getClassLoader());
}
/**
* Used internally obtain dependency information, such as for cyclical
* graph detection.
*/
@Override
public void getDependencies(Set> getBindings, Set> injectMembersBindings) {
getBindings.add(adapter);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public RawDataService get() {
return module.provideRawDataApi(adapter.get());
}
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Owning the dependency links between {@code AggregatedDataService} and its
* dependencies.
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ProvideAggDataApiProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
private Binding adapter;
public ProvideAggDataApiProvidesAdapter(ApiModule module) {
super("io.relayr.java.api.services.AggregatedDataService", IS_SINGLETON, "io.relayr.java.api.ApiModule", "provideAggDataApi");
this.module = module;
setLibrary(true);
}
/**
* Used internally to link bindings/providers together at run time
* according to their dependency graph.
*/
@Override
@SuppressWarnings("unchecked")
public void attach(Linker linker) {
adapter = (Binding) linker.requestBinding("@javax.inject.Named(value=api)/retrofit.RestAdapter", ApiModule.class, getClass().getClassLoader());
}
/**
* Used internally obtain dependency information, such as for cyclical
* graph detection.
*/
@Override
public void getDependencies(Set> getBindings, Set> injectMembersBindings) {
getBindings.add(adapter);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public AggregatedDataService get() {
return module.provideAggDataApi(adapter.get());
}
}
/**
* A {@code Binding} implementation which satisfies
* Dagger's infrastructure requirements including:
*
* Owning the dependency links between {@code NotificationService} and its
* dependencies.
*
* Being a {@code Provider} and handling creation and
* preparation of object instances.
*/
public static final class ProvideNotificationsApiProvidesAdapter extends ProvidesBinding {
private final ApiModule module;
private Binding adapter;
public ProvideNotificationsApiProvidesAdapter(ApiModule module) {
super("io.relayr.java.api.services.NotificationService", IS_SINGLETON, "io.relayr.java.api.ApiModule", "provideNotificationsApi");
this.module = module;
setLibrary(true);
}
/**
* Used internally to link bindings/providers together at run time
* according to their dependency graph.
*/
@Override
@SuppressWarnings("unchecked")
public void attach(Linker linker) {
adapter = (Binding) linker.requestBinding("@javax.inject.Named(value=api)/retrofit.RestAdapter", ApiModule.class, getClass().getClassLoader());
}
/**
* Used internally obtain dependency information, such as for cyclical
* graph detection.
*/
@Override
public void getDependencies(Set> getBindings, Set> injectMembersBindings) {
getBindings.add(adapter);
}
/**
* Returns the fully provisioned instance satisfying the contract for
* {@code Provider}.
*/
@Override
public NotificationService get() {
return module.provideNotificationsApi(adapter.get());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy