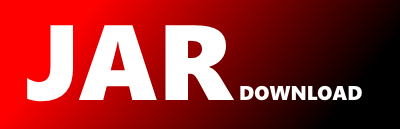
io.relayr.java.api.ChannelApi Maven / Gradle / Ivy
package io.relayr.java.api;
import io.relayr.java.RelayrJavaSdk;
import io.relayr.java.model.channel.ChannelDefinition;
import io.relayr.java.model.channel.ChannelTransport;
import io.relayr.java.model.channel.DataChannel;
import io.relayr.java.model.channel.ExistingChannel;
import io.relayr.java.model.channel.PublishChannel;
import retrofit.http.Body;
import retrofit.http.DELETE;
import retrofit.http.GET;
import retrofit.http.POST;
import retrofit.http.Path;
import rx.Observable;
public interface ChannelApi {
/**
* Creates an MQTT channel for a device specified with {@link ChannelDefinition#deviceId}
* Used in {@link RelayrJavaSdk#getWebSocketClient()} to create channels and subscribe to device readings.
* @param mqttDefinition - requires {@link ChannelDefinition} with {@link ChannelTransport#MQTT}
* @return an {@link DataChannel} with created MQTT channel credentials, {@link rx.Subscriber#onError(Throwable)} otherwise
*/
@POST("/channels") Observable create(@Body ChannelDefinition mqttDefinition);
/**
* Deletes an MQTT channel
* @param channelId existing channel ID
* @return an empty {@link Observable} if channel is deleted, {@link rx.Subscriber#onError(Throwable)} otherwise
*/
@DELETE("/channels/{channelId}") Observable delete(@Path("channelId") String channelId);
/**
* Returns existing MQTT channels for specified device.
* @param deviceId - device identificator
* @return an {@link ExistingChannel}, {@link rx.Subscriber#onError(Throwable)} otherwise
*/
@GET("/devices/{deviceId}/channels")
Observable getChannels(@Path("deviceId") String deviceId);
/**
* Create channel to publish device data to.
* @param mqttDefinition defines deviceId and type of transport {@link ChannelTransport#MQTT}
* @param deviceId device identificator
* @return an {@link PublishChannel}, {@link rx.Subscriber#onError(Throwable)} otherwise
*/
@POST("/devices/{deviceId}/transmitter")
Observable createForDevice(@Body ChannelDefinition mqttDefinition,
@Path("deviceId") String deviceId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy