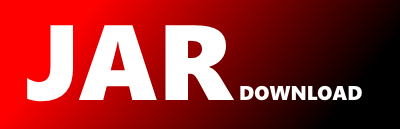
io.relayr.java.api.GroupsApi Maven / Gradle / Ivy
package io.relayr.java.api;
import io.relayr.java.model.groups.Group;
import io.relayr.java.model.groups.GroupCreate;
import io.relayr.java.model.groups.GroupDeviceAdd;
import io.relayr.java.model.groups.PositionUpdate;
import retrofit.http.Body;
import retrofit.http.DELETE;
import retrofit.http.GET;
import retrofit.http.PATCH;
import retrofit.http.POST;
import retrofit.http.Path;
import rx.Observable;
public interface GroupsApi {
/**
* Creates new group and returns 200 OK if group is successfully created, error otherwise.
* @param groupCreate {@link GroupCreate}
* @return an empty {@link Observable}
*/
@POST("/groups") Observable createGroup(@Body GroupCreate groupCreate);
/**
* Returns group defined with groupId
* @param groupId {@link Group#id}
* @return an {@link Observable}
*/
@GET("/groups/{groupId}") Observable getGroup(
@Path("groupId") String groupId);
/**
* Updates group and returns 200 OK if group is successfully updated, error otherwise.
* @param group group object with updated fields
* @param groupId {@link Group#id}
* @return an empty {@link Observable}
*/
@PATCH("/groups/{groupId}") Observable updateGroup(
@Body GroupCreate group, @Path("groupId") String groupId);
/**
* Adds device to a group and returns 200 OK if successful, error otherwise.
* @param groupId {@link Group#id}
* @param deviceIds {@link io.relayr.java.model.Device#id}
* @return an empty {@link Observable}
*/
@POST("/groups/{groupId}")
Observable addDevice(@Path("groupId") String groupId, @Body GroupDeviceAdd deviceIds);
/**
* Deletes device from a group and returns 200 OK if successful, error otherwise.
* @param groupId {@link Group#id}
* @param deviceId {@link io.relayr.java.model.Device#id}
* @return an empty {@link Observable}
*/
@DELETE("/groups/{groupId}/devices/{deviceId}")
Observable deleteDevice(@Path("groupId") String groupId,
@Path("deviceId") String deviceId);
/**
* Updates device's position in a group and returns 200 OK if successful, error otherwise.
* @param groupId {@link Group#id}
* @param deviceId {@link io.relayr.java.model.Device#id}
* @return an empty {@link Observable}
*/
@PATCH("/groups/{groupId}/devices/{deviceId}")
Observable updateDevicePosition(@Path("groupId") String groupId,
@Path("deviceId") String deviceId,
@Body PositionUpdate update);
/**
* Deletes group and returns 200 OK if group is successfully updated, error otherwise.
* @param groupId {@link Group#id}
* @return an empty {@link Observable}
*/
@DELETE("/groups/{groupId}") Observable deleteGroup(
@Path("groupId") String groupId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy