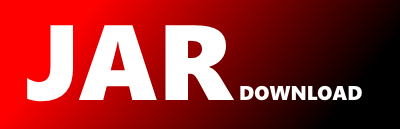
io.relayr.java.api.ProjectsApi Maven / Gradle / Ivy
package io.relayr.java.api;
import java.util.List;
import io.relayr.java.model.Publisher;
import io.relayr.java.model.User;
import io.relayr.java.model.projects.App;
import io.relayr.java.model.projects.ExtendedApp;
import retrofit.http.Body;
import retrofit.http.DELETE;
import retrofit.http.GET;
import retrofit.http.PATCH;
import retrofit.http.POST;
import retrofit.http.Path;
import rx.Observable;
public interface ProjectsApi {
/** @return information about the {@link App} initiating the request. */
@GET("/oauth2/app-info") Observable getAppInfo();
/** @return list of all {@link App} registered on relayr's platform */
@GET("/apps") Observable> getAllApps();
/** @return created {@link ExtendedApp} */
@POST("/apps") Observable createApp(@Body ExtendedApp app);
/** @return updated {@link ExtendedApp} */
@PATCH("/apps/{id}") Observable updateApp(@Path("id") String appId);
/** @return empty {@link Observable} */
@DELETE("/apps/{id}") Observable deleteApp(@Path("id") String appId);
/**
* @param appId - owned by user
* @return {@link ExtendedApp} defined by appId
*/
@GET("/apps/{id}") Observable getApp(@Path("id") String appId);
/**
* For more information about {@link Publisher} check {@link PublishersApi}
* @param publisherId - check {@link PublishersApi#getUserPublishers(String)}
* @return list of all {@link App} owned by {@link Publisher}.
*/
@GET("/publishers/{id}/apps")
Observable> getPublisherApps(@Path("id") String publisherId);
/**
* For more information about {@link Publisher} check {@link PublishersApi}
* @param publisherId - check {@link PublishersApi#getUserPublishers(String)}
* @return list of all {@link ExtendedApp} owned by {@link Publisher}.
*/
@GET("/publishers/{id}/apps/extended")
Observable> getPublisherExtendedApps(@Path("id") String publisherId);
/**
* @param userId
* @return list of all {@link ExtendedApp} owned by {@link User} defined with userId.
*/
@GET("/users/{id}/apps") Observable> getUserApps(@Path("id") String userId);
/**
* @param userId
* @param appId
* @param app
* @return updated {@link ExtendedApp} defined by appId owned by {@link User} defined by userId
*/
@POST("/users/{id}/apps/{appId}")
Observable updateUserApp(@Path("id") String userId, @Path("appId") String appId, @Body ExtendedApp app);
/**
* @param userId
* @param appId
* @return {@link ExtendedApp} defined by appId owned by {@link User} defined by userId
*/
@GET("/users/{id}/apps/{appId}")
Observable getUserApp(@Path("id") String userId, @Path("appId") String appId);
/**
* Deletes {@link ExtendedApp} defined by appId owned by {@link User} defined by userId
* @param userId
* @param appId
* @return empty {@link Observable}
*/
@DELETE("/users/{id}/apps/{appId}")
Observable deleteUserApp(@Path("id") String userId, @Path("appId") String appId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy