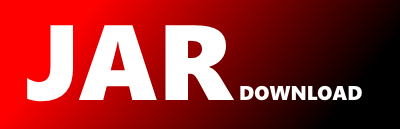
io.relayr.java.api.RuleTemplateApi Maven / Gradle / Ivy
package io.relayr.java.api;
import java.util.List;
import io.relayr.java.helper.observer.ResponseObserver;
import io.relayr.java.model.rules.AppliedTemplate;
import io.relayr.java.model.rules.Template;
import io.relayr.java.model.rules.TemplateInfo;
import io.relayr.java.model.rules.TemplateParameters;
import retrofit.client.Response;
import retrofit.http.Body;
import retrofit.http.DELETE;
import retrofit.http.GET;
import retrofit.http.PATCH;
import retrofit.http.POST;
import retrofit.http.Path;
import rx.Observable;
import rx.Observer;
/**
* Provides an API for the create of Rule Engine Rules on the base of pre made templates
*/
public interface RuleTemplateApi {
/**
* @param projectId
* @return a list of templates which are associated with this project
*/
@GET("/projects/{id}/templates")
Observable> getTemplates(@Path("id") String projectId);
/**
* @param projectId
* @param templateId
* @return detailed view on a template in a project. Also provides the latest version of this template (if it exists)
*/
@GET("/projects/{id}/templates/{templateId}")
Observable getTemplate(@Path("id") String projectId,
@Path("templateId") String templateId);
/**
* @param projectId
* @param templateId
* @return a list of all the versions which exist for one template. This list can be empty if no version has been created yet.
*/
@GET("/projects/{id}/templates/{templateId}/versions")
Observable> getTemplateVersions(@Path("id") String projectId,
@Path("templateId") String templateId);
/**
* @param projectId
* @param templateId
* @param templateVersionId
* @return the details about a template version
*/
@GET("/projects/{id}/templates/{templateId}/versions/{templateVersionId}")
Observable getTemplateVersion(@Path("id") String projectId,
@Path("templateId") String templateId,
@Path("templateVersionId") String templateVersionId);
/**
* Applies a given template. This means that the template is filled with the provided values and
* therefore a rule is created in the system.
* @param projectId
* @param templateId
* @param templateVersionId
* @return the created user installation.
*/
@POST("/projects/{id}/templates/{templateId}/versions/{templateVersionId}/apply")
Observable applyTemplate(@Body TemplateParameters parameters,
@Path("id") String projectId,
@Path("templateId") String templateId,
@Path("templateVersionId") String templateVersionId);
/**
* @param userId
* @return list of all the installations of templates of a user. If the user applied a template three times, you will get three installations here.
*/
@GET("/users/{userId}/ruletemplateinstallations")
Observable> getAppliedTemplates(@Path("userId") String userId);
@GET("/users/{userId}/ruletemplateinstallations/{installationId}")
Observable getAppliedTemplate(@Path("userId") String userId,
@Path("installationId") String installationId);
/**
* Removes a template installation. This means that this instance of the template is removed.
* @param userId
* @param installationId
* @return
* - 204 - If the deletion was successful
*
- 401 - provided Authentication credentials are invalid or the user doesn't own the installation
*
- 404 - the provided installation doesn't exist
*
*/
@DELETE("/users/{userId}/ruletemplateinstallations/{installationId}")
Observable deleteAppliedTemplate(@Path("userId") String userId,
@Path("installationId") String installationId);
/**
* Changes the properties of a rule template installation, i.e. the name or the configuration parameters
* @param userId
* @param installationId
*/
@PATCH("/users/{userId}/ruletemplateinstallations/{installationId}")
Observable updateAppliedTemplate(@Body Object ruleInstallation,
@Path("userId") String userId,
@Path("installationId") String installationId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy