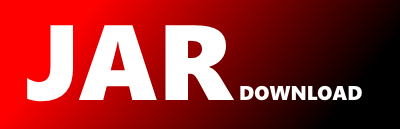
io.relayr.java.api.UserApi Maven / Gradle / Ivy
package io.relayr.java.api;
import java.util.List;
import io.relayr.java.model.Bookmark;
import io.relayr.java.model.device.BookmarkDevice;
import io.relayr.java.model.Device;
import io.relayr.java.model.Transmitter;
import io.relayr.java.model.User;
import io.relayr.java.model.account.Account;
import io.relayr.java.model.groups.Group;
import retrofit.http.Body;
import retrofit.http.DELETE;
import retrofit.http.GET;
import retrofit.http.PATCH;
import retrofit.http.POST;
import retrofit.http.Path;
import rx.Observable;
public interface UserApi {
/** @return an {@link Observable} information about the user initiating the request. */
@GET("/oauth2/user-info") Observable getUserInfo();
/**
* Returns user devices. Use {@link User#getDevices()} on fetched {@link User} object.
* @param userId
* @return an {@link Observable} of a list of devices registered under a user.
*/
@GET("/users/{userId}/devices")
Observable> getDevices(@Path("userId") String userId);
/**
* Returns list of groups created by user.
* @return an {@link Observable} with a list of all user groups {@link Group}.
*/
@GET("/users/{userId}/groups") Observable> getGroups(@Path("userId") String userId);
/**
* Deletes all groups and returns 200 OK if successful, error otherwise.
* @return an empty {@link Observable}
*/
@DELETE("/users/{userId}/groups")
Observable deleteAllGroups(@Path("userId") String userId);
/**
* Updates user details.
* @param userId
* @return Updated user object
*/
@PATCH("/users/{userId}")
Observable updateUserDetails(@Body User user, @Path("userId") String userId);
/**
* Returns user transmitters. Use {@link User#getTransmitters()} on fetched {@link User} object.
* @param userId
* @return an {@link Observable} with a list all Transmitters listed under a user.
*/
@GET("/users/{userId}/transmitters")
Observable> getTransmitters(@Path("userId") String userId);
/**
* Bookmarks a specific public device. By Bookmarking a device you are indicating that you have
* a particular interest in this device. In order to receive data from a bookmarked device,
* the subscription call must first be initiated.
* @param userId id of the user that is bookmarking the device
* @param deviceId id of bookmarked device - the Id must be one of a public device
* @return an {@link Observable} to the bookmarked device
*/
@POST("/users/{userId}/devices/{deviceId}/bookmarks")
Observable bookmarkPublicDevice(@Path("userId") String userId,
@Path("deviceId") String deviceId);
/**
* Deletes a bookmarked device.
* @param userId id of the user that bookmarked the device
* @param deviceId id of bookmarked device - the Id must be one of a public device
* @return an empty {@link Observable}
*/
@DELETE("/users/{userId}/devices/{deviceId}/bookmarks")
Observable deleteBookmark(@Path("userId") String userId,
@Path("deviceId") String deviceId);
/**
* Returns a list of devices bookmarked by the user.
* @param userId id of the user that bookmarked devices
* @return an {@link Observable} with a list of the users bookmarked devices
*/
@GET("/users/{userId}/devices/bookmarks")
Observable> getBookmarkedDevices(@Path("userId") String userId);
/**
* Returns a list of accounts that user connected to the relayr account.
* Use {@link User#getAccounts()} on fetched {@link User} object.
* @param userId id of the user
* @return an {@link Observable} with a list of accounts {@link Account}
*/
@GET("/users/{userId}/accounts")
Observable> getAccounts(@Path("userId") String userId);
/**
* Returns 200 OK (onNext()) if named account is connected to the users relayr account
* Use {@link User#isAccountConnected(String)} on fetched {@link User} object.
* @param userId id of the user
* @param accountName name of the connected account {@link Account#name}
* @return an empty {@link Observable}
*/
@GET("/users/{userId}/accounts/{accountName}/isconnected")
Observable isAccountConnected(@Path("userId") String userId,
@Path("accountName") String accountName);
/**
* Returns 200 OK (onNext()) if account is disconnected
* Use {@link User#disconnectAccount(String)} on fetched {@link User} object.
* @param userId id of the user
* @param accountName name of the connected account {@link Account#name}
* @return an empty {@link Observable}
*/
@POST("/users/{userId}/accounts/{accountName}/disconnect")
Observable disconnectAccount(@Path("userId") String userId,
@Path("accountName") String accountName);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy