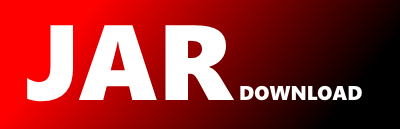
io.relayr.java.api.helpers.Aggregates Maven / Gradle / Ivy
package io.relayr.java.api.helpers;
import io.relayr.java.helper.Validator;
public class Aggregates {
public static String toList(Aggregate... aggregates) throws IllegalArgumentException {
Validator.requireNotNullNotEmpty(aggregates, "Aggregate list can't be null or empty");
String query = "";
for (Aggregate aggregate : aggregates) query += aggregate.getName() + ",";
return query.substring(0, query.length() - 1);
}
public enum Interval {
i10s("10s", 10), i1m("1m", 60), i5m("5m", 300), i30m("30m", 1800), i1h("1h", 3600);
private final String interval;
private final int numSeconds;
Interval(String interval, int seconds) {
this.interval = interval;
this.numSeconds = seconds;
}
public String getInterval() {
return interval;
}
public int inSeconds() {
return numSeconds;
}
@Override public String toString() {
return interval;
}
}
public enum Aggregate {
COUNT, NUM_COUNT, AVG, MIN, MAX, BOOL_COUNT, BOOL_VALUES, STRING_COUNT, STRING_VALUES,
LAT_LON_COUNT, LAT_LON_AVG, XYZ_COUNT, XYZ_AVG, LENGTH_AVG;
public String getName() {
String name = "";
final char[] chars = this.name().toLowerCase().toCharArray();
boolean underscore = false;
for (char aChar : chars) {
if (aChar != '_') {
if (underscore) {
name += Character.toUpperCase(aChar);
underscore = false;
} else name += aChar;
} else {
underscore = true;
}
}
return name;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy