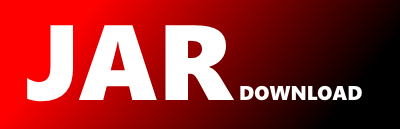
io.relayr.java.model.Transmitter Maven / Gradle / Ivy
package io.relayr.java.model;
import com.google.gson.annotations.SerializedName;
import java.io.Serializable;
import java.util.List;
import io.relayr.java.RelayrJavaSdk;
import io.relayr.java.model.account.AccountType;
import io.relayr.java.model.channel.DataChannel;
import rx.Observable;
/**
* The Transmitter class is a representation of the Transmitter entity.
* A Transmitter is another basic entity on the relayr platform.
* A transmitter contrary to a device does not gather data but is only used to relay the data
* from the devices to the relayr platform.
* The transmitter is also used to authenticate the different devices that transmit data via it.
*/
public class Transmitter implements Serializable {
private String id;
private String secret;
private String owner;
private String topic;
private String name;
private String clientId;
private DataChannel.ChannelCredentials credentials;
@SerializedName("integrationType") private String accountType;
public Transmitter(String owner, String name, AccountType type) {
this.owner = owner;
this.name = name;
this.accountType = type.getName();
}
/** Specific for WUNDERBAR v1 */
public Transmitter(String id, String secret, String owner, String name) {
this.id = id;
this.secret = secret;
this.owner = owner;
this.name = name;
accountType = AccountType.WUNDERBAR_1.getName();
}
/**
* @return an {@link Observable} with a list of devices that belong to the specific
* transmitter.
*/
public Observable> getDevices() {
return RelayrJavaSdk.getRelayrApi().getTransmitterDevices(id);
}
/**
* Updates a transmitter.
* @return an {@link Observable} to the updated Transmitter
*/
public Observable updateTransmitter() {
return RelayrJavaSdk.getRelayrApi().updateTransmitter(id, this);
}
public String getId() {
return id;
}
public String getSecret() {
return secret;
}
public String getOwner() {
return owner;
}
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
public String getTopic() {
return topic;
}
public AccountType getAccountType() {
return AccountType.getByName(accountType);
}
public String getClientId() {
return clientId;
}
public DataChannel.ChannelCredentials getCredentials() {
return credentials;
}
@Override public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Transmitter that = (Transmitter) o;
return !(id != null ? !id.equals(that.id) : that.id != null);
}
@Override public int hashCode() {
return id != null ? id.hashCode() : 0;
}
@Override public String toString() {
return "Transmitter{" +
"id='" + id + '\'' +
", secret='" + secret + '\'' +
", owner='" + owner + '\'' +
", topic='" + topic + '\'' +
", accountType='" + accountType + '\'' +
", name='" + name + '\'' +
", clientId='" + clientId + '\'' +
", credentials=" + credentials +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy