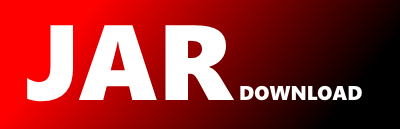
io.relayr.java.model.action.Action Maven / Gradle / Ivy
package io.relayr.java.model.action;
import java.io.Serializable;
import io.relayr.java.model.models.schema.ValueSchema;
import io.relayr.java.model.models.transport.DeviceConfiguration;
import rx.Observable;
import rx.Subscriber;
public abstract class Action implements Serializable {
protected String path;
protected String name;
protected Object value;
protected long ts;
protected transient boolean mValidated;
public boolean validate(ValueSchema schema) {
mValidated = schema.validate(value);
return mValidated;
}
protected Observable throwIfNull(final String what) {
return Observable.create(new Observable.OnSubscribe() {
@Override public void call(Subscriber super Void> subscriber) {
subscriber.onError(new NullPointerException(what + " can not be null!"));
}
});
}
protected Observable throwNotValidated() {
return Observable.create(new Observable.OnSubscribe() {
@Override public void call(Subscriber super Void> subscriber) {
subscriber.onError(new NullPointerException("Please validate before sending."));
}
});
}
/** Identifies the name of the action. */
public String getName() {
return name;
}
/** Identifies the component to which the configuration should be sent. */
public String getPath() {
return path;
}
/** Get current configuration value. Type of value object is defined with {@link DeviceConfiguration#getValueSchema()} */
public Object getValue() {
return value;
}
public long getTs() {
return ts;
}
public abstract Observable send(String deviceId);
@Override public String toString() {
return "path='" + path + '\'' +
", name='" + name + '\'' +
", value=" + value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy