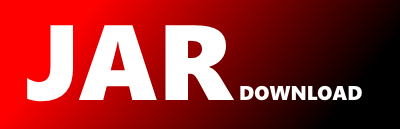
io.relayr.java.model.groups.Group Maven / Gradle / Ivy
package io.relayr.java.model.groups;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
import io.relayr.java.RelayrJavaSdk;
import io.relayr.java.model.Device;
import rx.Observable;
/** Group entity. Can contain one or more devices ordered by position. */
public class Group implements Serializable, Comparable {
private String id;
private String name;
private String owner;
private int position;
private List devices = new ArrayList<>();
private transient List realDevices = null;
public String getId() {
return id;
}
public String getName() {
return name;
}
/** @return list of {@link Device} in this group */
public List getDevices() {
Collections.sort(devices, new Comparator() {
@Override public int compare(GroupDevice lhs, GroupDevice rhs) {
return lhs.getPosition() - rhs.getPosition();
}
});
if (realDevices == null || realDevices.size() != devices.size()) {
realDevices = new ArrayList<>();
for (GroupDevice basic : devices)
realDevices.add(basic.toDevice());
}
return realDevices;
}
/**
* Calls {@link rx.Subscriber#onNext(Object)} if group is updated
* {@link rx.Subscriber#onError(Throwable)} otherwise.
* Subscription is necessary to run the method.
* @return {@link Observable}
*/
public Observable update(String name) {
//TODO Check with server guys about this. Currently it updates only one field
final GroupCreate groupUpdate = new GroupCreate(name, this.owner, position);
return update(groupUpdate);
}
/**
* Calls {@link rx.Subscriber#onNext(Object)} if group is updated
* {@link rx.Subscriber#onError(Throwable)} otherwise
* Subscription is necessary to run the method.
* @return {@link Observable}
*/
public Observable update(int position) {
final GroupCreate groupUpdate = new GroupCreate(name, this.owner, position);
return update(groupUpdate);
}
/**
* Updates object locally and calls {@link rx.Subscriber#onNext(Object)} if group is updated
* {@link rx.Subscriber#onError(Throwable)} otherwise.
* Subscription is necessary to run the method.
* @return {@link Observable}
*/
private Observable update(GroupCreate groupUpdate) {
this.name = groupUpdate.name;
return RelayrJavaSdk.getGroupsApi()
.updateGroup(groupUpdate, this.id);
}
/**
* Calls {@link rx.Subscriber#onNext(Object)} if device is added to the group.
* {@link rx.Subscriber#onError(Throwable)} otherwise
* Subscription is necessary to run the method.
* @return {@link Observable}
*/
public Observable addDevice(String... deviceIds) {
return RelayrJavaSdk.getGroupsApi().addDevice(id, new GroupDeviceAdd(deviceIds));
}
/**
* Calls {@link rx.Subscriber#onNext(Object)} if device is removed
* {@link rx.Subscriber#onError(Throwable)} otherwise
* Subscription is necessary to run the method.
* @return {@link Observable}
*/
public Observable removeDevice(String deviceId) {
return RelayrJavaSdk.getGroupsApi().deleteDevice(this.id, deviceId);
}
/**
* Calls {@link rx.Subscriber#onNext(Object)} if device is moved
* {@link rx.Subscriber#onError(Throwable)} otherwise
* Subscription is necessary to run the method.
* @return {@link Observable}
*/
public Observable moveDeviceTo(String deviceId, int newPosition) {
return RelayrJavaSdk.getGroupsApi()
.updateDevicePosition(id, deviceId, new PositionUpdate(newPosition));
}
@Override public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof Group)) return false;
Group group = (Group) o;
return id.equals(group.id);
}
@Override public int hashCode() {
return id.hashCode();
}
@Override public int compareTo(Group another) {
return this.position - another.position;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy