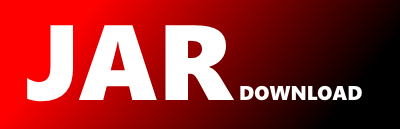
io.relayr.java.model.models.DeviceModels Maven / Gradle / Ivy
package io.relayr.java.model.models;
import java.io.Serializable;
import java.util.List;
import io.relayr.java.model.models.error.DeviceModelsException;
/**
* Object that represents all device models supported on relayr platform
* and a way of navigation through them.
*/
public class DeviceModels implements Serializable {
private ModelLinks _links;
private List models;
private List prototypes;
private int count;
private int limit;
private int offset;
/**
* Returns {@link ModelLinks} object with hyperlinks to other {@link DeviceModel} objects
* @return {@link ModelLinks}
*/
public ModelLinks getLinks() {
return _links;
}
/**
* Returns all device io.relayr.java.model supported on relayr platform.
*/
public List getModels() {
return models;
}
/**
* Returns all device io.relayr.java.model supported on relayr platform.
*/
public List getPrototypes() {
return prototypes;
}
/**
* Returns {@link DeviceModel} specified with modelId or {@link DeviceModelsException}
* if io.relayr.java.model with specified id doesn't exist.
* @return {@link DeviceModel} or {@link DeviceModelsException}
*/
public DeviceModel getModel(String modelId) throws DeviceModelsException {
for (DeviceModel model : models)
if (model.getId().equals(modelId))
return model;
throw DeviceModelsException.deviceModelNotFound();
}
/**
* Returns total number of models.
*/
public int getCount() {
return count;
}
/**
* Returns number of models that can be fetched when paging.
*/
public int getLimit() {
return limit;
}
/**
* Return offset from the first io.relayr.java.model.
*/
public int getOffset() {
return offset;
}
@Override public String toString() {
return "DeviceModels{" +
"_links=" + _links +
", models=" + models +
", prototypes=" + prototypes +
", count=" + count +
", limit=" + limit +
", offset=" + offset +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy