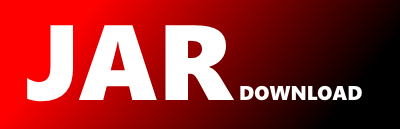
io.relayr.java.model.models.schema.ArraySchema Maven / Gradle / Ivy
package io.relayr.java.model.models.schema;
import java.util.List;
public class ArraySchema extends ValueSchema {
public ArraySchema(ValueSchema schema) {
super(schema);
items = schema.items;
maxItems = schema.maxItems;
minItems = schema.minItems;
uniqueItems = schema.uniqueItems;
additionalItems = schema.additionalItems;
}
/** Items of this array MUST be objects, and each of these objects MUST be a valid JSON Schema. */
public Object getItems() {
return items;
}
/** Items of this array MUST be objects, and each of these objects MUST be a valid JSON Schema. */
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy