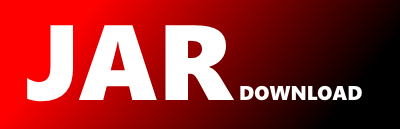
io.relayr.java.model.models.schema.NumberSchema Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-sdk Show documentation
Show all versions of java-sdk Show documentation
Java SDK for connecting to the Relayr Cloud
The newest version!
package io.relayr.java.model.models.schema;
public class NumberSchema extends ValueSchema {
public NumberSchema(ValueSchema schema) {
super(schema);
minimum = schema.minimum;
maximum = schema.maximum;
exclusiveMinimum = schema.exclusiveMinimum;
exclusiveMaximum = schema.exclusiveMaximum;
multipleOf = schema.multipleOf;
}
/** @return minimum value. */
public Number getMin() {
return minimum == null ? null : minimum.doubleValue();
}
/** @return maximum value. */
public Number getMax() {
return maximum == null ? null : maximum.doubleValue();
}
/**
* If "exclusiveMinimum" is present, "minimum" MUST also be present.
* if "exclusiveMinimum" is not present, or has boolean value false, then the instance is valid
* if it is greater than, or equal to, the value of "minimum";
* if "exclusiveMinimum" is present and has boolean value true, the instance is valid if
* it is strictly greater than the value of "minimum".
*/
public Boolean getExclusiveMin() {
return exclusiveMinimum;
}
/**
* If "exclusiveMaximum" is present, "maximum" MUST also be present.
* if "exclusiveMaximum" is not present, or has boolean value false, then the instance is valid
* if it is lower than, or equal to, the value of "maximum".
* if "exclusiveMaximum" has boolean value true, the instance is valid if it is strictly lower
* than the value of "maximum".
*/
public Boolean getExclusiveMax() {
return exclusiveMaximum;
}
/** If defined this number MUST be strictly greater than 0. */
public Number getMultipleOf() {
return multipleOf;
}
@Override public boolean validate(Object value) {
if (!validateNull(value)) return false;
if (!(value instanceof Number)) return false;
Double numValue;
try {
numValue = (Double) value;
} catch (ClassCastException e) {
numValue = ((Integer) value).doubleValue();
}
if (multipleOf != null)
if (numValue.intValue() % multipleOf.intValue() != 0) return false;
if (exclusiveMaximum != null && exclusiveMaximum) {
if (maximum == null) return false;
if (!(numValue < maximum.doubleValue())) return false;
} else {
if (maximum != null && !(numValue <= maximum.doubleValue())) return false;
}
if (exclusiveMinimum != null && exclusiveMinimum) {
if (minimum == null) return false;
if (!(numValue > minimum.doubleValue())) return false;
} else {
if (minimum != null && !(numValue >= minimum.doubleValue())) return false;
}
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy