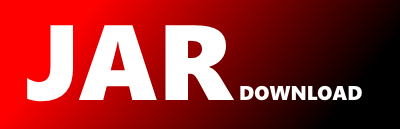
io.relayr.java.model.models.schema.StringSchema Maven / Gradle / Ivy
package io.relayr.java.model.models.schema;
public class StringSchema extends ValueSchema {
public StringSchema(ValueSchema schema) {
super(schema);
maxLength = schema.maxLength;
minLength = schema.minLength;
pattern = schema.pattern;
}
/** If defined this integer MUST be greater than, or equal to, 0. */
public Integer getMaxLength() {
return maxLength;
}
/** If defined this integer MUST be greater than, or equal to, 0. */
public Integer getMinLength() {
return minLength;
}
/** @return valid regular expression, according to the ECMA 262 regular expression dialect. */
public String getPattern() {
return pattern;
}
/** @return true if there are any possible values for the field. */
public boolean hasValues() {
return enums != null && !enums.isEmpty();
}
@Override public boolean validate(Object value) {
if (value == null && hasValues() && !getEnums().contains(null)) return false;
if (!validateNull(value)) return false;
if (value instanceof String) {
String stringValue = (String) value;
if (minLength != null)
if (stringValue.length() < minLength) return false;
if (maxLength != null)
if (stringValue.length() > maxLength) return false;
if (pattern != null)
if (!stringValue.matches(pattern)) return false;
if (hasValues()) {
boolean found = false;
for (Object item : getEnums())
if (item instanceof String)
if (stringValue.equals(item)) {
found = true;
break;
}
if (!found) return false;
}
return true;
} else {
if (hasValues()) return getEnums().contains(null);
else return true;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy