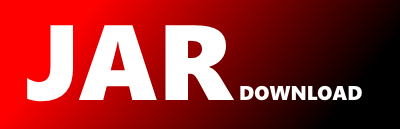
io.relayr.java.model.models.transport.Transport Maven / Gradle / Ivy
package io.relayr.java.model.models.transport;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import io.relayr.java.model.models.error.DeviceModelsException;
/**
* Defines all possible ways for user to interact with the device.
* Use for sending commands, setting configurations and formatting the readings.
*/
public class Transport implements Serializable {
private List commands;
private List readings;
private List configurations;
/** Returns all possible readings. See {@link DeviceReading} */
public List getReadings() {
return readings;
}
/** Returns all possible reading meanings as a list. */
public List getReadingMeanings() {
List meanings = new ArrayList<>();
for (DeviceReading reading : readings)
meanings.add(reading.getMeaning());
return meanings;
}
/** Returns reading depending on specified meaning. */
public DeviceReading getReadingByMeaning(String meaning, String path) throws DeviceModelsException {
if (meaning == null) throw DeviceModelsException.nullModelId();
for (DeviceReading reading : readings)
if (reading.getMeaning().equals(meaning) &&
((path != null && path.equals(reading.getPath())) ||
(path == null && reading.getPath() == null)))
return reading;
throw DeviceModelsException.deviceReadingNotFound();
}
/** Returns all possible commands. See {@link DeviceCommand} */
public List getCommands() {
return commands;
}
/** Returns all possible configurations. See {@link DeviceConfiguration} */
public List getConfigurations() {
return configurations;
}
@Override public String toString() {
return "Transport{" +
"commands=" + commands.toString() +
", readings=" + readings.toString() +
", configurations=" + configurations.toString() +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy