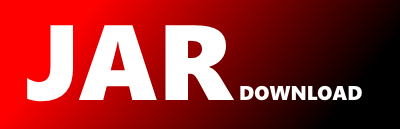
io.relayr.java.model.projects.ExtendedApp Maven / Gradle / Ivy
package io.relayr.java.model.projects;
import java.io.Serializable;
/**
* @see App
*/
public class ExtendedApp extends App implements Serializable {
private String publisher;
private String clientId;
private String clientSecret;
private String redirectUri;
/**
* Construction Method for an app
* @param id the relayr assigned id for the app instance
* @param name The name of this app
* @param description a brief description of the app
*/
public ExtendedApp(String id, String name, String description) {
super(id, name, description);
}
public ExtendedApp(String id, String name, String description, String publisher, String redirectUri) {
super(id, name, description);
this.publisher = publisher;
this.redirectUri = redirectUri;
}
/** The ID of the app's Publisher. */
public String getPublisherId() {
return publisher;
}
public String getClientId() {
return clientId;
}
/**
* Represents the OAuth client secret. Generated when creating an app on the relayr developer dashboard.
*/
public String getClientSecret() {
return clientSecret;
}
/** The URI of the page where the user is redirected upon successful login. The URI must include the protocol used e.g. 'http'. */
public String getRedirectUri() {
return redirectUri;
}
@Override public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
ExtendedApp that = (ExtendedApp) o;
return publisher != null ? publisher.equals(that.publisher) : that.publisher == null;
}
@Override public int hashCode() {
int result = super.hashCode();
result = 31 * result + (publisher != null ? publisher.hashCode() : 0);
return result;
}
@Override public String toString() {
return "ExtendedApp{" +
"publisher='" + publisher + '\'' +
", clientId='" + clientId + '\'' +
", clientSecret='" + clientSecret + '\'' +
", redirectUri='" + redirectUri + '\'' +
"} " + super.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy