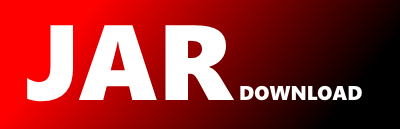
io.relayr.java.model.state.State Maven / Gradle / Ivy
package io.relayr.java.model.state;
import java.io.Serializable;
import java.util.List;
import io.relayr.java.model.action.Command;
import io.relayr.java.model.action.Configuration;
import io.relayr.java.model.action.Reading;
/***
* Device state is a device specific storage.
* It shows latest state of devices' readings, configuration, commands and metadata.
* Latest state refers to a list of latest changes in device actions.
* If device, for example, is sending a reading every number of seconds only latest reading will
* be saved and persisted in the state
*/
public class State implements Serializable {
private List readings;
private List commands;
private List configurations;
private Object metadata;
private Version version;
/***
* Returns list of devices' latest send readings and version.
* Device readings are defined in {@link io.relayr.java.model.models.transport.Transport}
* that can be find in {@link io.relayr.java.model.models.DeviceModel} for each device.
* @return {@link StateReadings}
*/
public StateReadings getReadings() {
return new StateReadings(version, readings);
}
/***
* Returns list of devices' latest used commands and version.
* Device commands are defined in {@link io.relayr.java.model.models.transport.Transport}
* that can be find in {@link io.relayr.java.model.models.DeviceModel} for each device.
* @return {@link StateCommands}
*/
public StateCommands getCommands() {
return new StateCommands(version, commands);
}
/***
* Returns list of devices' latest used configurations and version.
* Device configurations are defined in {@link io.relayr.java.model.models.transport.Transport}
* that can be find in {@link io.relayr.java.model.models.DeviceModel} for each device.
* @return {@link StateCommands}
*/
public StateConfigurations getConfigurations() {
return new StateConfigurations(version, configurations);
}
/***
* Metadata is an dictionary object defined by user. It can be used to save device specific data
* that's not defined by device model.
* @return {@link Object}
*/
public Object getMetadata() {
return metadata;
}
/***
* Returns current device state version. Version is defined with incremental number and
* timestamp of last update.
*/
public Version getVersion() {
return version;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy