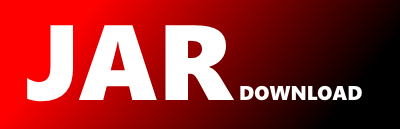
io.relayr.java.websocket.WebSocket Maven / Gradle / Ivy
package io.relayr.java.websocket;
import org.eclipse.paho.client.mqttv3.IMqttDeliveryToken;
import org.eclipse.paho.client.mqttv3.MqttAsyncClient;
import org.eclipse.paho.client.mqttv3.MqttException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import io.relayr.java.model.channel.DataChannel;
import rx.Observable;
abstract class WebSocket {
protected static final Object mLock = new Object();
protected static final int CONNECT_TIMEOUT = 15000;
protected static final int SUBSCRIBE_TIMEOUT = 5000;
protected static final int UNSUBSCRIBE_TIMEOUT = 2000;
protected MqttAsyncClient mClient = null;
protected Map mPublishClients = new HashMap<>();
protected Map> mTopicCallbacks = new HashMap<>();
public WebSocket() {
SslUtil.init();
}
public boolean isConnected() {
return mClient != null && mClient.isConnected();
}
protected boolean publish(String deviceId, String topic, String payload) throws MqttException {
final byte[] data = payload == null ? new byte[]{} : payload.getBytes();
if (mPublishClients.get(deviceId) == null) return false;
if (!mPublishClients.get(deviceId).isConnected()) return false;
try {
final IMqttDeliveryToken publishToken = mPublishClients.get(deviceId).publish(topic, data, 0, false);
publishToken.waitForCompletion();
return true;
} catch (Exception e) {
System.err.println("Publish error " + e.getMessage());
return false;
}
}
abstract Observable createClient(T object);
abstract Observable createPublishClient(T object);
abstract boolean subscribe(String topic, String channelId, final WebSocketCallback callback);
abstract boolean subscribeAction(String topic, String deviceId, String channelId, WebSocketCallback webSocketCallback);
abstract boolean unSubscribe(String topic);
public synchronized void reset() {
killClient(mClient);
if (mPublishClients != null && !mPublishClients.isEmpty()) {
for (MqttAsyncClient client : mPublishClients.values())
killClient(client);
mPublishClients.clear();
mTopicCallbacks.clear();
}
}
private synchronized void killClient(MqttAsyncClient client) {
disconnectClient(client);
closeClient(client);
}
private synchronized void disconnectClient(MqttAsyncClient client) {
if (client == null) return;
try {
System.out.println("Disconnecting client " + client.getClientId());
client.disconnect();
} catch (MqttException e) {
System.err.println("Failed to disconnect MQTT client");
}
}
private synchronized void closeClient(MqttAsyncClient client) {
if (client == null) return;
System.out.println("Closing client " + client.getClientId());
try {
client.close();
} catch (MqttException e) {
System.err.println("Failed to close MQTT client");
}
}
public void removePublishClient(String deviceId) {
final MqttAsyncClient client = mPublishClients.get(deviceId);
killClient(client);
mPublishClients.remove(deviceId);
mTopicCallbacks.remove(deviceId);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy