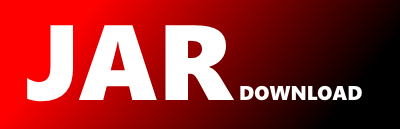
invirt.data.mongodb.MongoEntity.kt Maven / Gradle / Ivy
package invirt.data.mongodb
import com.mongodb.client.model.ReplaceOptions
import com.mongodb.kotlin.client.MongoCollection
import com.mongodb.kotlin.client.MongoDatabase
import java.time.Instant
import java.time.temporal.ChronoUnit
import kotlin.reflect.full.findAnnotation
interface MongoEntity {
val id: String
}
interface TimestampedEntity : MongoEntity {
@Indexed
val createdAt: Instant
@Indexed
var updatedAt: Instant
}
@Target(AnnotationTarget.CLASS)
@Retention(AnnotationRetention.RUNTIME)
@MustBeDocumented
annotation class CollectionName(val name: String)
inline fun collectionName(): String {
val annotation = T::class.findAnnotation()
?: throw IllegalStateException("Class ${T::class} doesn't have an @CollectionName annotation")
return annotation.name
}
fun MongoCollection.save(entity: T): T {
if (entity is TimestampedEntity) {
entity.updatedAt = mongoNow()
}
replaceOne(byId(entity.id), entity, ReplaceOptions().upsert(true))
return entity
}
inline fun MongoDatabase.collection(): MongoCollection {
return getCollection(collectionName())
}
/**
* MongoDB only support millisecond precision
*/
fun mongoNow(): Instant = Instant.now().truncatedTo(ChronoUnit.MILLIS)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy