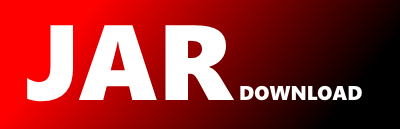
invirt.data.mongodb.query.kt Maven / Gradle / Ivy
package invirt.data.mongodb
import com.mongodb.kotlin.client.FindIterable
import com.mongodb.kotlin.client.MongoCollection
import invirt.data.Page
import invirt.data.RecordsPage
import invirt.data.Sort
import org.bson.conversions.Bson
interface MongoQuery {
val page: Page
val sort: Sort?
val filter: Bson?
}
inline fun MongoCollection.query(searchQuery: MongoQuery): RecordsPage {
return this.query(searchQuery.filter, searchQuery.page, searchQuery.sort)
}
inline fun MongoCollection.query(
filter: Bson?,
page: Page = Page(0, 10),
sort: Sort? = null
): RecordsPage {
// This isn't optimal as it performs two Mongo queries, but the alternative is using
// aggregations (see commented code below) and things get very complex, particularly with text search
var (count: Long, iterable: FindIterable) = if (filter != null) {
Pair(this.countDocuments(filter), this.find(filter).page(page))
} else {
Pair(this.countDocuments(), this.find().page(page))
}
if (sort != null) {
iterable = iterable.sort(sort.mongoSort())
}
return RecordsPage(
records = iterable.toList(),
count = count,
page = page,
sort = sort
)
}
//data class CountObject(val count: Long)
//data class SearchResult(
// val results: List,
// val count: List
//) {
// inline fun typesResults(collection: MongoCollection): List {
// val codec = collection.codecRegistry[T::class.java]
// return results.map {
// codec.decode(BsonDocumentReader(it), defaultDecoderContext)
// }
// }
//}
//
//inline fun MongoCollection.query(
// filter: Bson?,
// page: Page = Page(0, 10),
// sort: Sort? = null
//): PagedResults {
// val queryPipeline = mutableListOf()
// if (filter != null) {
// queryPipeline.add(Aggregates.match(filter))
// }
// if (sort != null) {
// queryPipeline.add(Aggregates.sort(sort.mongoSort()))
// }
// queryPipeline.add(Aggregates.skip(page.from))
// queryPipeline.add(Aggregates.limit(page.size))
//
// val countPipeline = mutableListOf()
// if (filter != null) {
// countPipeline.add(Aggregates.match(filter))
// }
// countPipeline.add(Aggregates.count())
//
// val facets = Aggregates.facet(
// Facet(SearchResult::results.name, queryPipeline),
// Facet(SearchResult::count.name, countPipeline)
// )
// val searchResult = this.withDocumentClass().aggregate(listOf(facets)).first()
// val count = searchResult.count.firstOrNull()?.count ?: 0
// return PagedResults(
// results = searchResult.typesResults(this),
// count = count,
// page = Page(page, count),
// sort = sort
// )
//}
//
© 2015 - 2025 Weber Informatics LLC | Privacy Policy