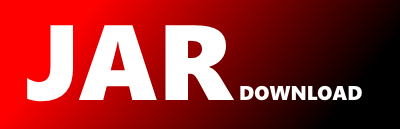
io.resys.hdes.ast.HdesParserVisitor Maven / Gradle / Ivy
The newest version!
// Generated from HdesParser.g4 by ANTLR 4.8
package io.resys.hdes.ast;
import org.antlr.v4.runtime.tree.ParseTreeVisitor;
/**
* This interface defines a complete generic visitor for a parse tree produced
* by {@link HdesParser}.
*
* @param The return type of the visit operation. Use {@link Void} for
* operations with no return type.
*/
public interface HdesParserVisitor extends ParseTreeVisitor {
/**
* Visit a parse tree produced by {@link HdesParser#hdesContent}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitHdesContent(HdesParser.HdesContentContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#hdesBody}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitHdesBody(HdesParser.HdesBodyContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#decisionTableUnit}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDecisionTableUnit(HdesParser.DecisionTableUnitContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#hitPolicy}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitHitPolicy(HdesParser.HitPolicyContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#matchingPolicy}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMatchingPolicy(HdesParser.MatchingPolicyContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#whenThenRules}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWhenThenRules(HdesParser.WhenThenRulesContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#mappingPolicy}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMappingPolicy(HdesParser.MappingPolicyContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#mappingFrom}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMappingFrom(HdesParser.MappingFromContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#mappingTo}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMappingTo(HdesParser.MappingToContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#mappingRows}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMappingRows(HdesParser.MappingRowsContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#mappingRow}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMappingRow(HdesParser.MappingRowContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#thenRules}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitThenRules(HdesParser.ThenRulesContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#whenRules}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWhenRules(HdesParser.WhenRulesContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#ruleLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRuleLiteral(HdesParser.RuleLiteralContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#ruleExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRuleExpression(HdesParser.RuleExpressionContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#ruleUndefinedValue}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRuleUndefinedValue(HdesParser.RuleUndefinedValueContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#scalarType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitScalarType(HdesParser.ScalarTypeContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#headers}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitHeaders(HdesParser.HeadersContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#headersAccepts}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitHeadersAccepts(HdesParser.HeadersAcceptsContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#headersReturns}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitHeadersReturns(HdesParser.HeadersReturnsContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#typeDefs}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTypeDefs(HdesParser.TypeDefsContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#typeDef}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTypeDef(HdesParser.TypeDefContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#typeDefNames}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTypeDefNames(HdesParser.TypeDefNamesContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#typeDefName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTypeDefName(HdesParser.TypeDefNameContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#simpleType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSimpleType(HdesParser.SimpleTypeContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#objectType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitObjectType(HdesParser.ObjectTypeContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#arrayType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArrayType(HdesParser.ArrayTypeContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#optional}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOptional(HdesParser.OptionalContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#debugValue}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDebugValue(HdesParser.DebugValueContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#formula}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFormula(HdesParser.FormulaContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#formulaOverAll}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFormulaOverAll(HdesParser.FormulaOverAllContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#mapping}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMapping(HdesParser.MappingContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#mappingArg}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMappingArg(HdesParser.MappingArgContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#fieldMapping}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFieldMapping(HdesParser.FieldMappingContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#fastMapping}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFastMapping(HdesParser.FastMappingContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#mappingArray}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMappingArray(HdesParser.MappingArrayContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#mappingValue}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMappingValue(HdesParser.MappingValueContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLiteral(HdesParser.LiteralContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#placeholderRule}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPlaceholderRule(HdesParser.PlaceholderRuleContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#placeholderTypeName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPlaceholderTypeName(HdesParser.PlaceholderTypeNameContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#simpleTypeName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSimpleTypeName(HdesParser.SimpleTypeNameContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#typeName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTypeName(HdesParser.TypeNameContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#methodName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMethodName(HdesParser.MethodNameContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#methodInvocation}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMethodInvocation(HdesParser.MethodInvocationContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#staticMethod}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStaticMethod(HdesParser.StaticMethodContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#instanceMethod}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInstanceMethod(HdesParser.InstanceMethodContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#instanceMethodChild}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInstanceMethodChild(HdesParser.InstanceMethodChildContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#mappingMethod}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMappingMethod(HdesParser.MappingMethodContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#mappingMethodChild}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMappingMethodChild(HdesParser.MappingMethodChildContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#mapMethod}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMapMethod(HdesParser.MapMethodContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#filterMethod}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFilterMethod(HdesParser.FilterMethodContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#sortMethod}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSortMethod(HdesParser.SortMethodContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#findFirstMethod}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFindFirstMethod(HdesParser.FindFirstMethodContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#methodArgs}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMethodArgs(HdesParser.MethodArgsContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#methodArg}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMethodArg(HdesParser.MethodArgContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#primary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPrimary(HdesParser.PrimaryContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#expressionUnit}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExpressionUnit(HdesParser.ExpressionUnitContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExpression(HdesParser.ExpressionContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#lambdaExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLambdaExpression(HdesParser.LambdaExpressionContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#lambdaParameters}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLambdaParameters(HdesParser.LambdaParametersContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#lambdaBody}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLambdaBody(HdesParser.LambdaBodyContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#conditionalExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitConditionalExpression(HdesParser.ConditionalExpressionContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#conditionalOrExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitConditionalOrExpression(HdesParser.ConditionalOrExpressionContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#conditionalAndExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitConditionalAndExpression(HdesParser.ConditionalAndExpressionContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#andExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAndExpression(HdesParser.AndExpressionContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#equalityExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEqualityExpression(HdesParser.EqualityExpressionContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#relationalExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRelationalExpression(HdesParser.RelationalExpressionContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#additiveExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAdditiveExpression(HdesParser.AdditiveExpressionContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#multiplicativeExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMultiplicativeExpression(HdesParser.MultiplicativeExpressionContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#unaryExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUnaryExpression(HdesParser.UnaryExpressionContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#unaryExpressionNotPlusMinus}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUnaryExpressionNotPlusMinus(HdesParser.UnaryExpressionNotPlusMinusContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#serviceTaskUnit}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitServiceTaskUnit(HdesParser.ServiceTaskUnitContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#promise}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPromise(HdesParser.PromiseContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#promiseTimeout}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPromiseTimeout(HdesParser.PromiseTimeoutContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#externalService}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExternalService(HdesParser.ExternalServiceContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#flowUnit}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFlowUnit(HdesParser.FlowUnitContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#steps}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSteps(HdesParser.StepsContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#step}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStep(HdesParser.StepContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#stepAs}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStepAs(HdesParser.StepAsContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#callAction}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCallAction(HdesParser.CallActionContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#callDef}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCallDef(HdesParser.CallDefContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#callAwait}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCallAwait(HdesParser.CallAwaitContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#iterateAction}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIterateAction(HdesParser.IterateActionContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#iterateBody}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIterateBody(HdesParser.IterateBodyContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#pointer}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPointer(HdesParser.PointerContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#whenThenPointerArgs}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWhenThenPointerArgs(HdesParser.WhenThenPointerArgsContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#whenThenPointer}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWhenThenPointer(HdesParser.WhenThenPointerContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#elsePointer}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitElsePointer(HdesParser.ElsePointerContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#thenPointer}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitThenPointer(HdesParser.ThenPointerContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#continuePointer}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitContinuePointer(HdesParser.ContinuePointerContext ctx);
/**
* Visit a parse tree produced by {@link HdesParser#endAsPointer}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEndAsPointer(HdesParser.EndAsPointerContext ctx);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy