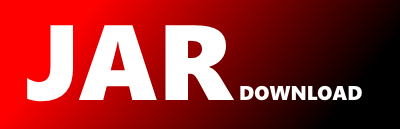
io.resys.hdes.ast.api.nodes.ImmutableDecisionTableBody Maven / Gradle / Ivy
package io.resys.hdes.ast.api.nodes;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link DecisionTableNode.DecisionTableBody}.
*
* Use the builder to create immutable instances:
* {@code ImmutableDecisionTableBody.builder()}.
*/
@Generated(from = "DecisionTableNode.DecisionTableBody", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class ImmutableDecisionTableBody
implements DecisionTableNode.DecisionTableBody {
private final HdesNode.Token token;
private final BodyNode.BodyId id;
private final BodyNode.Headers headers;
private final DecisionTableNode.HitPolicy hitPolicy;
private final BodyNode.ObjectDef constants;
private final BodyNode.ObjectDef matched;
private ImmutableDecisionTableBody(
HdesNode.Token token,
BodyNode.BodyId id,
BodyNode.Headers headers,
DecisionTableNode.HitPolicy hitPolicy,
BodyNode.ObjectDef constants,
BodyNode.ObjectDef matched) {
this.token = token;
this.id = id;
this.headers = headers;
this.hitPolicy = hitPolicy;
this.constants = constants;
this.matched = matched;
}
/**
* @return The value of the {@code token} attribute
*/
@Override
public HdesNode.Token getToken() {
return token;
}
/**
* @return The value of the {@code id} attribute
*/
@Override
public BodyNode.BodyId getId() {
return id;
}
/**
* @return The value of the {@code headers} attribute
*/
@Override
public BodyNode.Headers getHeaders() {
return headers;
}
/**
* @return The value of the {@code hitPolicy} attribute
*/
@Override
public DecisionTableNode.HitPolicy getHitPolicy() {
return hitPolicy;
}
/**
* @return The value of the {@code constants} attribute
*/
@Override
public BodyNode.ObjectDef getConstants() {
return constants;
}
/**
* @return The value of the {@code matched} attribute
*/
@Override
public BodyNode.ObjectDef getMatched() {
return matched;
}
/**
* Copy the current immutable object by setting a value for the {@link DecisionTableNode.DecisionTableBody#getToken() token} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for token
* @return A modified copy of the {@code this} object
*/
public final ImmutableDecisionTableBody withToken(HdesNode.Token value) {
if (this.token == value) return this;
HdesNode.Token newValue = Objects.requireNonNull(value, "token");
return new ImmutableDecisionTableBody(newValue, this.id, this.headers, this.hitPolicy, this.constants, this.matched);
}
/**
* Copy the current immutable object by setting a value for the {@link DecisionTableNode.DecisionTableBody#getId() id} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for id
* @return A modified copy of the {@code this} object
*/
public final ImmutableDecisionTableBody withId(BodyNode.BodyId value) {
if (this.id == value) return this;
BodyNode.BodyId newValue = Objects.requireNonNull(value, "id");
return new ImmutableDecisionTableBody(this.token, newValue, this.headers, this.hitPolicy, this.constants, this.matched);
}
/**
* Copy the current immutable object by setting a value for the {@link DecisionTableNode.DecisionTableBody#getHeaders() headers} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for headers
* @return A modified copy of the {@code this} object
*/
public final ImmutableDecisionTableBody withHeaders(BodyNode.Headers value) {
if (this.headers == value) return this;
BodyNode.Headers newValue = Objects.requireNonNull(value, "headers");
return new ImmutableDecisionTableBody(this.token, this.id, newValue, this.hitPolicy, this.constants, this.matched);
}
/**
* Copy the current immutable object by setting a value for the {@link DecisionTableNode.DecisionTableBody#getHitPolicy() hitPolicy} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for hitPolicy
* @return A modified copy of the {@code this} object
*/
public final ImmutableDecisionTableBody withHitPolicy(DecisionTableNode.HitPolicy value) {
if (this.hitPolicy == value) return this;
DecisionTableNode.HitPolicy newValue = Objects.requireNonNull(value, "hitPolicy");
return new ImmutableDecisionTableBody(this.token, this.id, this.headers, newValue, this.constants, this.matched);
}
/**
* Copy the current immutable object by setting a value for the {@link DecisionTableNode.DecisionTableBody#getConstants() constants} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for constants
* @return A modified copy of the {@code this} object
*/
public final ImmutableDecisionTableBody withConstants(BodyNode.ObjectDef value) {
if (this.constants == value) return this;
BodyNode.ObjectDef newValue = Objects.requireNonNull(value, "constants");
return new ImmutableDecisionTableBody(this.token, this.id, this.headers, this.hitPolicy, newValue, this.matched);
}
/**
* Copy the current immutable object by setting a value for the {@link DecisionTableNode.DecisionTableBody#getMatched() matched} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for matched
* @return A modified copy of the {@code this} object
*/
public final ImmutableDecisionTableBody withMatched(BodyNode.ObjectDef value) {
if (this.matched == value) return this;
BodyNode.ObjectDef newValue = Objects.requireNonNull(value, "matched");
return new ImmutableDecisionTableBody(this.token, this.id, this.headers, this.hitPolicy, this.constants, newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableDecisionTableBody} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableDecisionTableBody
&& equalTo((ImmutableDecisionTableBody) another);
}
private boolean equalTo(ImmutableDecisionTableBody another) {
return token.equals(another.token)
&& id.equals(another.id)
&& headers.equals(another.headers)
&& hitPolicy.equals(another.hitPolicy)
&& constants.equals(another.constants)
&& matched.equals(another.matched);
}
/**
* Computes a hash code from attributes: {@code token}, {@code id}, {@code headers}, {@code hitPolicy}, {@code constants}, {@code matched}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + token.hashCode();
h += (h << 5) + id.hashCode();
h += (h << 5) + headers.hashCode();
h += (h << 5) + hitPolicy.hashCode();
h += (h << 5) + constants.hashCode();
h += (h << 5) + matched.hashCode();
return h;
}
/**
* Prints the immutable value {@code DecisionTableBody} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "DecisionTableBody{"
+ "token=" + token
+ ", id=" + id
+ ", headers=" + headers
+ ", hitPolicy=" + hitPolicy
+ ", constants=" + constants
+ ", matched=" + matched
+ "}";
}
/**
* Creates an immutable copy of a {@link DecisionTableNode.DecisionTableBody} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable DecisionTableBody instance
*/
public static ImmutableDecisionTableBody copyOf(DecisionTableNode.DecisionTableBody instance) {
if (instance instanceof ImmutableDecisionTableBody) {
return (ImmutableDecisionTableBody) instance;
}
return ImmutableDecisionTableBody.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableDecisionTableBody ImmutableDecisionTableBody}.
*
* ImmutableDecisionTableBody.builder()
* .token(io.resys.hdes.ast.api.nodes.HdesNode.Token) // required {@link DecisionTableNode.DecisionTableBody#getToken() token}
* .id(io.resys.hdes.ast.api.nodes.BodyNode.BodyId) // required {@link DecisionTableNode.DecisionTableBody#getId() id}
* .headers(io.resys.hdes.ast.api.nodes.BodyNode.Headers) // required {@link DecisionTableNode.DecisionTableBody#getHeaders() headers}
* .hitPolicy(io.resys.hdes.ast.api.nodes.DecisionTableNode.HitPolicy) // required {@link DecisionTableNode.DecisionTableBody#getHitPolicy() hitPolicy}
* .constants(io.resys.hdes.ast.api.nodes.BodyNode.ObjectDef) // required {@link DecisionTableNode.DecisionTableBody#getConstants() constants}
* .matched(io.resys.hdes.ast.api.nodes.BodyNode.ObjectDef) // required {@link DecisionTableNode.DecisionTableBody#getMatched() matched}
* .build();
*
* @return A new ImmutableDecisionTableBody builder
*/
public static ImmutableDecisionTableBody.Builder builder() {
return new ImmutableDecisionTableBody.Builder();
}
/**
* Builds instances of type {@link ImmutableDecisionTableBody ImmutableDecisionTableBody}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "DecisionTableNode.DecisionTableBody", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_TOKEN = 0x1L;
private static final long INIT_BIT_ID = 0x2L;
private static final long INIT_BIT_HEADERS = 0x4L;
private static final long INIT_BIT_HIT_POLICY = 0x8L;
private static final long INIT_BIT_CONSTANTS = 0x10L;
private static final long INIT_BIT_MATCHED = 0x20L;
private long initBits = 0x3fL;
private @Nullable HdesNode.Token token;
private @Nullable BodyNode.BodyId id;
private @Nullable BodyNode.Headers headers;
private @Nullable DecisionTableNode.HitPolicy hitPolicy;
private @Nullable BodyNode.ObjectDef constants;
private @Nullable BodyNode.ObjectDef matched;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code io.resys.hdes.ast.api.nodes.HdesNode} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(HdesNode instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code io.resys.hdes.ast.api.nodes.BodyNode} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(BodyNode instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code io.resys.hdes.ast.api.nodes.DecisionTableNode.DecisionTableBody} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(DecisionTableNode.DecisionTableBody instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
if (object instanceof HdesNode) {
HdesNode instance = (HdesNode) object;
token(instance.getToken());
}
if (object instanceof BodyNode) {
BodyNode instance = (BodyNode) object;
headers(instance.getHeaders());
id(instance.getId());
}
if (object instanceof DecisionTableNode.DecisionTableBody) {
DecisionTableNode.DecisionTableBody instance = (DecisionTableNode.DecisionTableBody) object;
hitPolicy(instance.getHitPolicy());
matched(instance.getMatched());
constants(instance.getConstants());
}
}
/**
* Initializes the value for the {@link DecisionTableNode.DecisionTableBody#getToken() token} attribute.
* @param token The value for token
* @return {@code this} builder for use in a chained invocation
*/
public final Builder token(HdesNode.Token token) {
this.token = Objects.requireNonNull(token, "token");
initBits &= ~INIT_BIT_TOKEN;
return this;
}
/**
* Initializes the value for the {@link DecisionTableNode.DecisionTableBody#getId() id} attribute.
* @param id The value for id
* @return {@code this} builder for use in a chained invocation
*/
public final Builder id(BodyNode.BodyId id) {
this.id = Objects.requireNonNull(id, "id");
initBits &= ~INIT_BIT_ID;
return this;
}
/**
* Initializes the value for the {@link DecisionTableNode.DecisionTableBody#getHeaders() headers} attribute.
* @param headers The value for headers
* @return {@code this} builder for use in a chained invocation
*/
public final Builder headers(BodyNode.Headers headers) {
this.headers = Objects.requireNonNull(headers, "headers");
initBits &= ~INIT_BIT_HEADERS;
return this;
}
/**
* Initializes the value for the {@link DecisionTableNode.DecisionTableBody#getHitPolicy() hitPolicy} attribute.
* @param hitPolicy The value for hitPolicy
* @return {@code this} builder for use in a chained invocation
*/
public final Builder hitPolicy(DecisionTableNode.HitPolicy hitPolicy) {
this.hitPolicy = Objects.requireNonNull(hitPolicy, "hitPolicy");
initBits &= ~INIT_BIT_HIT_POLICY;
return this;
}
/**
* Initializes the value for the {@link DecisionTableNode.DecisionTableBody#getConstants() constants} attribute.
* @param constants The value for constants
* @return {@code this} builder for use in a chained invocation
*/
public final Builder constants(BodyNode.ObjectDef constants) {
this.constants = Objects.requireNonNull(constants, "constants");
initBits &= ~INIT_BIT_CONSTANTS;
return this;
}
/**
* Initializes the value for the {@link DecisionTableNode.DecisionTableBody#getMatched() matched} attribute.
* @param matched The value for matched
* @return {@code this} builder for use in a chained invocation
*/
public final Builder matched(BodyNode.ObjectDef matched) {
this.matched = Objects.requireNonNull(matched, "matched");
initBits &= ~INIT_BIT_MATCHED;
return this;
}
/**
* Builds a new {@link ImmutableDecisionTableBody ImmutableDecisionTableBody}.
* @return An immutable instance of DecisionTableBody
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableDecisionTableBody build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableDecisionTableBody(token, id, headers, hitPolicy, constants, matched);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_TOKEN) != 0) attributes.add("token");
if ((initBits & INIT_BIT_ID) != 0) attributes.add("id");
if ((initBits & INIT_BIT_HEADERS) != 0) attributes.add("headers");
if ((initBits & INIT_BIT_HIT_POLICY) != 0) attributes.add("hitPolicy");
if ((initBits & INIT_BIT_CONSTANTS) != 0) attributes.add("constants");
if ((initBits & INIT_BIT_MATCHED) != 0) attributes.add("matched");
return "Cannot build DecisionTableBody, some of required attributes are not set " + attributes;
}
}
}