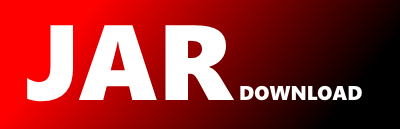
io.resys.hdes.ast.api.nodes.ImmutableLambdaExpression Maven / Gradle / Ivy
package io.resys.hdes.ast.api.nodes;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link ExpressionNode.LambdaExpression}.
*
* Use the builder to create immutable instances:
* {@code ImmutableLambdaExpression.builder()}.
*/
@Generated(from = "ExpressionNode.LambdaExpression", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class ImmutableLambdaExpression
implements ExpressionNode.LambdaExpression {
private final HdesNode.Token token;
private final InvocationNode type;
private final InvocationNode.SimpleInvocation param;
private final HdesNode body;
private final Boolean findFirst;
private final List sort;
private final List filter;
private final @Nullable HdesNode next;
private ImmutableLambdaExpression(
HdesNode.Token token,
InvocationNode type,
InvocationNode.SimpleInvocation param,
HdesNode body,
Boolean findFirst,
List sort,
List filter,
@Nullable HdesNode next) {
this.token = token;
this.type = type;
this.param = param;
this.body = body;
this.findFirst = findFirst;
this.sort = sort;
this.filter = filter;
this.next = next;
}
/**
* @return The value of the {@code token} attribute
*/
@Override
public HdesNode.Token getToken() {
return token;
}
/**
* @return The value of the {@code type} attribute
*/
@Override
public InvocationNode getType() {
return type;
}
/**
* @return The value of the {@code param} attribute
*/
@Override
public InvocationNode.SimpleInvocation getParam() {
return param;
}
/**
* @return The value of the {@code body} attribute
*/
@Override
public HdesNode getBody() {
return body;
}
/**
* @return The value of the {@code findFirst} attribute
*/
@Override
public Boolean getFindFirst() {
return findFirst;
}
/**
* @return The value of the {@code sort} attribute
*/
@Override
public List getSort() {
return sort;
}
/**
* @return The value of the {@code filter} attribute
*/
@Override
public List getFilter() {
return filter;
}
/**
* @return The value of the {@code next} attribute
*/
@Override
public Optional getNext() {
return Optional.ofNullable(next);
}
/**
* Copy the current immutable object by setting a value for the {@link ExpressionNode.LambdaExpression#getToken() token} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for token
* @return A modified copy of the {@code this} object
*/
public final ImmutableLambdaExpression withToken(HdesNode.Token value) {
if (this.token == value) return this;
HdesNode.Token newValue = Objects.requireNonNull(value, "token");
return new ImmutableLambdaExpression(newValue, this.type, this.param, this.body, this.findFirst, this.sort, this.filter, this.next);
}
/**
* Copy the current immutable object by setting a value for the {@link ExpressionNode.LambdaExpression#getType() type} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for type
* @return A modified copy of the {@code this} object
*/
public final ImmutableLambdaExpression withType(InvocationNode value) {
if (this.type == value) return this;
InvocationNode newValue = Objects.requireNonNull(value, "type");
return new ImmutableLambdaExpression(this.token, newValue, this.param, this.body, this.findFirst, this.sort, this.filter, this.next);
}
/**
* Copy the current immutable object by setting a value for the {@link ExpressionNode.LambdaExpression#getParam() param} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for param
* @return A modified copy of the {@code this} object
*/
public final ImmutableLambdaExpression withParam(InvocationNode.SimpleInvocation value) {
if (this.param == value) return this;
InvocationNode.SimpleInvocation newValue = Objects.requireNonNull(value, "param");
return new ImmutableLambdaExpression(this.token, this.type, newValue, this.body, this.findFirst, this.sort, this.filter, this.next);
}
/**
* Copy the current immutable object by setting a value for the {@link ExpressionNode.LambdaExpression#getBody() body} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for body
* @return A modified copy of the {@code this} object
*/
public final ImmutableLambdaExpression withBody(HdesNode value) {
if (this.body == value) return this;
HdesNode newValue = Objects.requireNonNull(value, "body");
return new ImmutableLambdaExpression(this.token, this.type, this.param, newValue, this.findFirst, this.sort, this.filter, this.next);
}
/**
* Copy the current immutable object by setting a value for the {@link ExpressionNode.LambdaExpression#getFindFirst() findFirst} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for findFirst
* @return A modified copy of the {@code this} object
*/
public final ImmutableLambdaExpression withFindFirst(Boolean value) {
Boolean newValue = Objects.requireNonNull(value, "findFirst");
if (this.findFirst.equals(newValue)) return this;
return new ImmutableLambdaExpression(this.token, this.type, this.param, this.body, newValue, this.sort, this.filter, this.next);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ExpressionNode.LambdaExpression#getSort() sort}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableLambdaExpression withSort(ExpressionNode.LambdaSortExpression... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableLambdaExpression(this.token, this.type, this.param, this.body, this.findFirst, newValue, this.filter, this.next);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ExpressionNode.LambdaExpression#getSort() sort}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of sort elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableLambdaExpression withSort(Iterable extends ExpressionNode.LambdaSortExpression> elements) {
if (this.sort == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableLambdaExpression(this.token, this.type, this.param, this.body, this.findFirst, newValue, this.filter, this.next);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ExpressionNode.LambdaExpression#getFilter() filter}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableLambdaExpression withFilter(ExpressionNode.LambdaFilterExpression... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableLambdaExpression(this.token, this.type, this.param, this.body, this.findFirst, this.sort, newValue, this.next);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ExpressionNode.LambdaExpression#getFilter() filter}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of filter elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableLambdaExpression withFilter(Iterable extends ExpressionNode.LambdaFilterExpression> elements) {
if (this.filter == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableLambdaExpression(this.token, this.type, this.param, this.body, this.findFirst, this.sort, newValue, this.next);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link ExpressionNode.LambdaExpression#getNext() next} attribute.
* @param value The value for next
* @return A modified copy of {@code this} object
*/
public final ImmutableLambdaExpression withNext(HdesNode value) {
@Nullable HdesNode newValue = Objects.requireNonNull(value, "next");
if (this.next == newValue) return this;
return new ImmutableLambdaExpression(this.token, this.type, this.param, this.body, this.findFirst, this.sort, this.filter, newValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link ExpressionNode.LambdaExpression#getNext() next} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for next
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final ImmutableLambdaExpression withNext(Optional extends HdesNode> optional) {
@Nullable HdesNode value = optional.orElse(null);
if (this.next == value) return this;
return new ImmutableLambdaExpression(this.token, this.type, this.param, this.body, this.findFirst, this.sort, this.filter, value);
}
/**
* This instance is equal to all instances of {@code ImmutableLambdaExpression} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableLambdaExpression
&& equalTo((ImmutableLambdaExpression) another);
}
private boolean equalTo(ImmutableLambdaExpression another) {
return token.equals(another.token)
&& type.equals(another.type)
&& param.equals(another.param)
&& body.equals(another.body)
&& findFirst.equals(another.findFirst)
&& sort.equals(another.sort)
&& filter.equals(another.filter)
&& Objects.equals(next, another.next);
}
/**
* Computes a hash code from attributes: {@code token}, {@code type}, {@code param}, {@code body}, {@code findFirst}, {@code sort}, {@code filter}, {@code next}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + token.hashCode();
h += (h << 5) + type.hashCode();
h += (h << 5) + param.hashCode();
h += (h << 5) + body.hashCode();
h += (h << 5) + findFirst.hashCode();
h += (h << 5) + sort.hashCode();
h += (h << 5) + filter.hashCode();
h += (h << 5) + Objects.hashCode(next);
return h;
}
/**
* Prints the immutable value {@code LambdaExpression} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("LambdaExpression{");
builder.append("token=").append(token);
builder.append(", ");
builder.append("type=").append(type);
builder.append(", ");
builder.append("param=").append(param);
builder.append(", ");
builder.append("body=").append(body);
builder.append(", ");
builder.append("findFirst=").append(findFirst);
builder.append(", ");
builder.append("sort=").append(sort);
builder.append(", ");
builder.append("filter=").append(filter);
if (next != null) {
builder.append(", ");
builder.append("next=").append(next);
}
return builder.append("}").toString();
}
/**
* Creates an immutable copy of a {@link ExpressionNode.LambdaExpression} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable LambdaExpression instance
*/
public static ImmutableLambdaExpression copyOf(ExpressionNode.LambdaExpression instance) {
if (instance instanceof ImmutableLambdaExpression) {
return (ImmutableLambdaExpression) instance;
}
return ImmutableLambdaExpression.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableLambdaExpression ImmutableLambdaExpression}.
*
* ImmutableLambdaExpression.builder()
* .token(io.resys.hdes.ast.api.nodes.HdesNode.Token) // required {@link ExpressionNode.LambdaExpression#getToken() token}
* .type(io.resys.hdes.ast.api.nodes.InvocationNode) // required {@link ExpressionNode.LambdaExpression#getType() type}
* .param(io.resys.hdes.ast.api.nodes.InvocationNode.SimpleInvocation) // required {@link ExpressionNode.LambdaExpression#getParam() param}
* .body(io.resys.hdes.ast.api.nodes.HdesNode) // required {@link ExpressionNode.LambdaExpression#getBody() body}
* .findFirst(Boolean) // required {@link ExpressionNode.LambdaExpression#getFindFirst() findFirst}
* .addSort|addAllSort(io.resys.hdes.ast.api.nodes.ExpressionNode.LambdaSortExpression) // {@link ExpressionNode.LambdaExpression#getSort() sort} elements
* .addFilter|addAllFilter(io.resys.hdes.ast.api.nodes.ExpressionNode.LambdaFilterExpression) // {@link ExpressionNode.LambdaExpression#getFilter() filter} elements
* .next(io.resys.hdes.ast.api.nodes.HdesNode) // optional {@link ExpressionNode.LambdaExpression#getNext() next}
* .build();
*
* @return A new ImmutableLambdaExpression builder
*/
public static ImmutableLambdaExpression.Builder builder() {
return new ImmutableLambdaExpression.Builder();
}
/**
* Builds instances of type {@link ImmutableLambdaExpression ImmutableLambdaExpression}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "ExpressionNode.LambdaExpression", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_TOKEN = 0x1L;
private static final long INIT_BIT_TYPE = 0x2L;
private static final long INIT_BIT_PARAM = 0x4L;
private static final long INIT_BIT_BODY = 0x8L;
private static final long INIT_BIT_FIND_FIRST = 0x10L;
private long initBits = 0x1fL;
private @Nullable HdesNode.Token token;
private @Nullable InvocationNode type;
private @Nullable InvocationNode.SimpleInvocation param;
private @Nullable HdesNode body;
private @Nullable Boolean findFirst;
private List sort = new ArrayList();
private List filter = new ArrayList();
private @Nullable HdesNode next;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code io.resys.hdes.ast.api.nodes.HdesNode} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(HdesNode instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code io.resys.hdes.ast.api.nodes.ExpressionNode.LambdaExpression} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(ExpressionNode.LambdaExpression instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
if (object instanceof HdesNode) {
HdesNode instance = (HdesNode) object;
token(instance.getToken());
}
if (object instanceof ExpressionNode.LambdaExpression) {
ExpressionNode.LambdaExpression instance = (ExpressionNode.LambdaExpression) object;
addAllFilter(instance.getFilter());
Optional nextOptional = instance.getNext();
if (nextOptional.isPresent()) {
next(nextOptional);
}
param(instance.getParam());
findFirst(instance.getFindFirst());
addAllSort(instance.getSort());
type(instance.getType());
body(instance.getBody());
}
}
/**
* Initializes the value for the {@link ExpressionNode.LambdaExpression#getToken() token} attribute.
* @param token The value for token
* @return {@code this} builder for use in a chained invocation
*/
public final Builder token(HdesNode.Token token) {
this.token = Objects.requireNonNull(token, "token");
initBits &= ~INIT_BIT_TOKEN;
return this;
}
/**
* Initializes the value for the {@link ExpressionNode.LambdaExpression#getType() type} attribute.
* @param type The value for type
* @return {@code this} builder for use in a chained invocation
*/
public final Builder type(InvocationNode type) {
this.type = Objects.requireNonNull(type, "type");
initBits &= ~INIT_BIT_TYPE;
return this;
}
/**
* Initializes the value for the {@link ExpressionNode.LambdaExpression#getParam() param} attribute.
* @param param The value for param
* @return {@code this} builder for use in a chained invocation
*/
public final Builder param(InvocationNode.SimpleInvocation param) {
this.param = Objects.requireNonNull(param, "param");
initBits &= ~INIT_BIT_PARAM;
return this;
}
/**
* Initializes the value for the {@link ExpressionNode.LambdaExpression#getBody() body} attribute.
* @param body The value for body
* @return {@code this} builder for use in a chained invocation
*/
public final Builder body(HdesNode body) {
this.body = Objects.requireNonNull(body, "body");
initBits &= ~INIT_BIT_BODY;
return this;
}
/**
* Initializes the value for the {@link ExpressionNode.LambdaExpression#getFindFirst() findFirst} attribute.
* @param findFirst The value for findFirst
* @return {@code this} builder for use in a chained invocation
*/
public final Builder findFirst(Boolean findFirst) {
this.findFirst = Objects.requireNonNull(findFirst, "findFirst");
initBits &= ~INIT_BIT_FIND_FIRST;
return this;
}
/**
* Adds one element to {@link ExpressionNode.LambdaExpression#getSort() sort} list.
* @param element A sort element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addSort(ExpressionNode.LambdaSortExpression element) {
this.sort.add(Objects.requireNonNull(element, "sort element"));
return this;
}
/**
* Adds elements to {@link ExpressionNode.LambdaExpression#getSort() sort} list.
* @param elements An array of sort elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addSort(ExpressionNode.LambdaSortExpression... elements) {
for (ExpressionNode.LambdaSortExpression element : elements) {
this.sort.add(Objects.requireNonNull(element, "sort element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link ExpressionNode.LambdaExpression#getSort() sort} list.
* @param elements An iterable of sort elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder sort(Iterable extends ExpressionNode.LambdaSortExpression> elements) {
this.sort.clear();
return addAllSort(elements);
}
/**
* Adds elements to {@link ExpressionNode.LambdaExpression#getSort() sort} list.
* @param elements An iterable of sort elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllSort(Iterable extends ExpressionNode.LambdaSortExpression> elements) {
for (ExpressionNode.LambdaSortExpression element : elements) {
this.sort.add(Objects.requireNonNull(element, "sort element"));
}
return this;
}
/**
* Adds one element to {@link ExpressionNode.LambdaExpression#getFilter() filter} list.
* @param element A filter element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addFilter(ExpressionNode.LambdaFilterExpression element) {
this.filter.add(Objects.requireNonNull(element, "filter element"));
return this;
}
/**
* Adds elements to {@link ExpressionNode.LambdaExpression#getFilter() filter} list.
* @param elements An array of filter elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addFilter(ExpressionNode.LambdaFilterExpression... elements) {
for (ExpressionNode.LambdaFilterExpression element : elements) {
this.filter.add(Objects.requireNonNull(element, "filter element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link ExpressionNode.LambdaExpression#getFilter() filter} list.
* @param elements An iterable of filter elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder filter(Iterable extends ExpressionNode.LambdaFilterExpression> elements) {
this.filter.clear();
return addAllFilter(elements);
}
/**
* Adds elements to {@link ExpressionNode.LambdaExpression#getFilter() filter} list.
* @param elements An iterable of filter elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllFilter(Iterable extends ExpressionNode.LambdaFilterExpression> elements) {
for (ExpressionNode.LambdaFilterExpression element : elements) {
this.filter.add(Objects.requireNonNull(element, "filter element"));
}
return this;
}
/**
* Initializes the optional value {@link ExpressionNode.LambdaExpression#getNext() next} to next.
* @param next The value for next
* @return {@code this} builder for chained invocation
*/
public final Builder next(HdesNode next) {
this.next = Objects.requireNonNull(next, "next");
return this;
}
/**
* Initializes the optional value {@link ExpressionNode.LambdaExpression#getNext() next} to next.
* @param next The value for next
* @return {@code this} builder for use in a chained invocation
*/
public final Builder next(Optional extends HdesNode> next) {
this.next = next.orElse(null);
return this;
}
/**
* Builds a new {@link ImmutableLambdaExpression ImmutableLambdaExpression}.
* @return An immutable instance of LambdaExpression
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableLambdaExpression build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableLambdaExpression(
token,
type,
param,
body,
findFirst,
createUnmodifiableList(true, sort),
createUnmodifiableList(true, filter),
next);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_TOKEN) != 0) attributes.add("token");
if ((initBits & INIT_BIT_TYPE) != 0) attributes.add("type");
if ((initBits & INIT_BIT_PARAM) != 0) attributes.add("param");
if ((initBits & INIT_BIT_BODY) != 0) attributes.add("body");
if ((initBits & INIT_BIT_FIND_FIRST) != 0) attributes.add("findFirst");
return "Cannot build LambdaExpression, some of required attributes are not set " + attributes;
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>();
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}