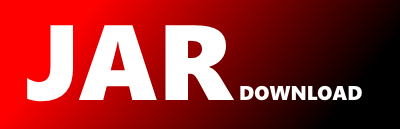
io.resys.hdes.ast.api.nodes.ImmutableObjectDef Maven / Gradle / Ivy
package io.resys.hdes.ast.api.nodes;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link BodyNode.ObjectDef}.
*
* Use the builder to create immutable instances:
* {@code ImmutableObjectDef.builder()}.
*/
@Generated(from = "BodyNode.ObjectDef", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class ImmutableObjectDef implements BodyNode.ObjectDef {
private final HdesNode.Token token;
private final Boolean required;
private final String name;
private final Boolean array;
private final BodyNode.ContextTypeDef context;
private final List values;
private ImmutableObjectDef(
HdesNode.Token token,
Boolean required,
String name,
Boolean array,
BodyNode.ContextTypeDef context,
List values) {
this.token = token;
this.required = required;
this.name = name;
this.array = array;
this.context = context;
this.values = values;
}
/**
* @return The value of the {@code token} attribute
*/
@Override
public HdesNode.Token getToken() {
return token;
}
/**
* @return The value of the {@code required} attribute
*/
@Override
public Boolean getRequired() {
return required;
}
/**
* @return The value of the {@code name} attribute
*/
@Override
public String getName() {
return name;
}
/**
* @return The value of the {@code array} attribute
*/
@Override
public Boolean getArray() {
return array;
}
/**
* @return The value of the {@code context} attribute
*/
@Override
public BodyNode.ContextTypeDef getContext() {
return context;
}
/**
* @return The value of the {@code values} attribute
*/
@Override
public List getValues() {
return values;
}
/**
* Copy the current immutable object by setting a value for the {@link BodyNode.ObjectDef#getToken() token} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for token
* @return A modified copy of the {@code this} object
*/
public final ImmutableObjectDef withToken(HdesNode.Token value) {
if (this.token == value) return this;
HdesNode.Token newValue = Objects.requireNonNull(value, "token");
return new ImmutableObjectDef(newValue, this.required, this.name, this.array, this.context, this.values);
}
/**
* Copy the current immutable object by setting a value for the {@link BodyNode.ObjectDef#getRequired() required} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for required
* @return A modified copy of the {@code this} object
*/
public final ImmutableObjectDef withRequired(Boolean value) {
Boolean newValue = Objects.requireNonNull(value, "required");
if (this.required.equals(newValue)) return this;
return new ImmutableObjectDef(this.token, newValue, this.name, this.array, this.context, this.values);
}
/**
* Copy the current immutable object by setting a value for the {@link BodyNode.ObjectDef#getName() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name
* @return A modified copy of the {@code this} object
*/
public final ImmutableObjectDef withName(String value) {
String newValue = Objects.requireNonNull(value, "name");
if (this.name.equals(newValue)) return this;
return new ImmutableObjectDef(this.token, this.required, newValue, this.array, this.context, this.values);
}
/**
* Copy the current immutable object by setting a value for the {@link BodyNode.ObjectDef#getArray() array} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for array
* @return A modified copy of the {@code this} object
*/
public final ImmutableObjectDef withArray(Boolean value) {
Boolean newValue = Objects.requireNonNull(value, "array");
if (this.array.equals(newValue)) return this;
return new ImmutableObjectDef(this.token, this.required, this.name, newValue, this.context, this.values);
}
/**
* Copy the current immutable object by setting a value for the {@link BodyNode.ObjectDef#getContext() context} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for context
* @return A modified copy of the {@code this} object
*/
public final ImmutableObjectDef withContext(BodyNode.ContextTypeDef value) {
if (this.context == value) return this;
BodyNode.ContextTypeDef newValue = Objects.requireNonNull(value, "context");
if (this.context.equals(newValue)) return this;
return new ImmutableObjectDef(this.token, this.required, this.name, this.array, newValue, this.values);
}
/**
* Copy the current immutable object with elements that replace the content of {@link BodyNode.ObjectDef#getValues() values}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableObjectDef withValues(BodyNode.TypeDef... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableObjectDef(this.token, this.required, this.name, this.array, this.context, newValue);
}
/**
* Copy the current immutable object with elements that replace the content of {@link BodyNode.ObjectDef#getValues() values}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of values elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableObjectDef withValues(Iterable extends BodyNode.TypeDef> elements) {
if (this.values == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableObjectDef(this.token, this.required, this.name, this.array, this.context, newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableObjectDef} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableObjectDef
&& equalTo((ImmutableObjectDef) another);
}
private boolean equalTo(ImmutableObjectDef another) {
return token.equals(another.token)
&& required.equals(another.required)
&& name.equals(another.name)
&& array.equals(another.array)
&& context.equals(another.context)
&& values.equals(another.values);
}
/**
* Computes a hash code from attributes: {@code token}, {@code required}, {@code name}, {@code array}, {@code context}, {@code values}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + token.hashCode();
h += (h << 5) + required.hashCode();
h += (h << 5) + name.hashCode();
h += (h << 5) + array.hashCode();
h += (h << 5) + context.hashCode();
h += (h << 5) + values.hashCode();
return h;
}
/**
* Prints the immutable value {@code ObjectDef} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "ObjectDef{"
+ "token=" + token
+ ", required=" + required
+ ", name=" + name
+ ", array=" + array
+ ", context=" + context
+ ", values=" + values
+ "}";
}
/**
* Creates an immutable copy of a {@link BodyNode.ObjectDef} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable ObjectDef instance
*/
public static ImmutableObjectDef copyOf(BodyNode.ObjectDef instance) {
if (instance instanceof ImmutableObjectDef) {
return (ImmutableObjectDef) instance;
}
return ImmutableObjectDef.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableObjectDef ImmutableObjectDef}.
*
* ImmutableObjectDef.builder()
* .token(io.resys.hdes.ast.api.nodes.HdesNode.Token) // required {@link BodyNode.ObjectDef#getToken() token}
* .required(Boolean) // required {@link BodyNode.ObjectDef#getRequired() required}
* .name(String) // required {@link BodyNode.ObjectDef#getName() name}
* .array(Boolean) // required {@link BodyNode.ObjectDef#getArray() array}
* .context(io.resys.hdes.ast.api.nodes.BodyNode.ContextTypeDef) // required {@link BodyNode.ObjectDef#getContext() context}
* .addValues|addAllValues(io.resys.hdes.ast.api.nodes.BodyNode.TypeDef) // {@link BodyNode.ObjectDef#getValues() values} elements
* .build();
*
* @return A new ImmutableObjectDef builder
*/
public static ImmutableObjectDef.Builder builder() {
return new ImmutableObjectDef.Builder();
}
/**
* Builds instances of type {@link ImmutableObjectDef ImmutableObjectDef}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "BodyNode.ObjectDef", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_TOKEN = 0x1L;
private static final long INIT_BIT_REQUIRED = 0x2L;
private static final long INIT_BIT_NAME = 0x4L;
private static final long INIT_BIT_ARRAY = 0x8L;
private static final long INIT_BIT_CONTEXT = 0x10L;
private long initBits = 0x1fL;
private @Nullable HdesNode.Token token;
private @Nullable Boolean required;
private @Nullable String name;
private @Nullable Boolean array;
private @Nullable BodyNode.ContextTypeDef context;
private List values = new ArrayList();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code io.resys.hdes.ast.api.nodes.HdesNode} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(HdesNode instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code io.resys.hdes.ast.api.nodes.BodyNode.TypeDef} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(BodyNode.TypeDef instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code io.resys.hdes.ast.api.nodes.BodyNode.ObjectDef} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(BodyNode.ObjectDef instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
if (object instanceof HdesNode) {
HdesNode instance = (HdesNode) object;
token(instance.getToken());
}
if (object instanceof BodyNode.TypeDef) {
BodyNode.TypeDef instance = (BodyNode.TypeDef) object;
name(instance.getName());
context(instance.getContext());
array(instance.getArray());
required(instance.getRequired());
}
if (object instanceof BodyNode.ObjectDef) {
BodyNode.ObjectDef instance = (BodyNode.ObjectDef) object;
addAllValues(instance.getValues());
}
}
/**
* Initializes the value for the {@link BodyNode.ObjectDef#getToken() token} attribute.
* @param token The value for token
* @return {@code this} builder for use in a chained invocation
*/
public final Builder token(HdesNode.Token token) {
this.token = Objects.requireNonNull(token, "token");
initBits &= ~INIT_BIT_TOKEN;
return this;
}
/**
* Initializes the value for the {@link BodyNode.ObjectDef#getRequired() required} attribute.
* @param required The value for required
* @return {@code this} builder for use in a chained invocation
*/
public final Builder required(Boolean required) {
this.required = Objects.requireNonNull(required, "required");
initBits &= ~INIT_BIT_REQUIRED;
return this;
}
/**
* Initializes the value for the {@link BodyNode.ObjectDef#getName() name} attribute.
* @param name The value for name
* @return {@code this} builder for use in a chained invocation
*/
public final Builder name(String name) {
this.name = Objects.requireNonNull(name, "name");
initBits &= ~INIT_BIT_NAME;
return this;
}
/**
* Initializes the value for the {@link BodyNode.ObjectDef#getArray() array} attribute.
* @param array The value for array
* @return {@code this} builder for use in a chained invocation
*/
public final Builder array(Boolean array) {
this.array = Objects.requireNonNull(array, "array");
initBits &= ~INIT_BIT_ARRAY;
return this;
}
/**
* Initializes the value for the {@link BodyNode.ObjectDef#getContext() context} attribute.
* @param context The value for context
* @return {@code this} builder for use in a chained invocation
*/
public final Builder context(BodyNode.ContextTypeDef context) {
this.context = Objects.requireNonNull(context, "context");
initBits &= ~INIT_BIT_CONTEXT;
return this;
}
/**
* Adds one element to {@link BodyNode.ObjectDef#getValues() values} list.
* @param element A values element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addValues(BodyNode.TypeDef element) {
this.values.add(Objects.requireNonNull(element, "values element"));
return this;
}
/**
* Adds elements to {@link BodyNode.ObjectDef#getValues() values} list.
* @param elements An array of values elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addValues(BodyNode.TypeDef... elements) {
for (BodyNode.TypeDef element : elements) {
this.values.add(Objects.requireNonNull(element, "values element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link BodyNode.ObjectDef#getValues() values} list.
* @param elements An iterable of values elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder values(Iterable extends BodyNode.TypeDef> elements) {
this.values.clear();
return addAllValues(elements);
}
/**
* Adds elements to {@link BodyNode.ObjectDef#getValues() values} list.
* @param elements An iterable of values elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllValues(Iterable extends BodyNode.TypeDef> elements) {
for (BodyNode.TypeDef element : elements) {
this.values.add(Objects.requireNonNull(element, "values element"));
}
return this;
}
/**
* Builds a new {@link ImmutableObjectDef ImmutableObjectDef}.
* @return An immutable instance of ObjectDef
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableObjectDef build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableObjectDef(token, required, name, array, context, createUnmodifiableList(true, values));
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_TOKEN) != 0) attributes.add("token");
if ((initBits & INIT_BIT_REQUIRED) != 0) attributes.add("required");
if ((initBits & INIT_BIT_NAME) != 0) attributes.add("name");
if ((initBits & INIT_BIT_ARRAY) != 0) attributes.add("array");
if ((initBits & INIT_BIT_CONTEXT) != 0) attributes.add("context");
return "Cannot build ObjectDef, some of required attributes are not set " + attributes;
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>();
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}