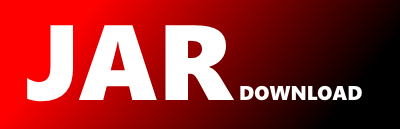
io.resys.hdes.ast.api.nodes.ImmutableScalarDef Maven / Gradle / Ivy
package io.resys.hdes.ast.api.nodes;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link BodyNode.ScalarDef}.
*
* Use the builder to create immutable instances:
* {@code ImmutableScalarDef.builder()}.
*/
@Generated(from = "BodyNode.ScalarDef", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class ImmutableScalarDef implements BodyNode.ScalarDef {
private final HdesNode.Token token;
private final Boolean required;
private final String name;
private final Boolean array;
private final BodyNode.ContextTypeDef context;
private final @Nullable String debugValue;
private final @Nullable ExpressionNode.ExpressionBody formula;
private final @Nullable Boolean formulaOverAll;
private final BodyNode.ScalarType type;
private ImmutableScalarDef(
HdesNode.Token token,
Boolean required,
String name,
Boolean array,
BodyNode.ContextTypeDef context,
@Nullable String debugValue,
@Nullable ExpressionNode.ExpressionBody formula,
@Nullable Boolean formulaOverAll,
BodyNode.ScalarType type) {
this.token = token;
this.required = required;
this.name = name;
this.array = array;
this.context = context;
this.debugValue = debugValue;
this.formula = formula;
this.formulaOverAll = formulaOverAll;
this.type = type;
}
/**
* @return The value of the {@code token} attribute
*/
@Override
public HdesNode.Token getToken() {
return token;
}
/**
* @return The value of the {@code required} attribute
*/
@Override
public Boolean getRequired() {
return required;
}
/**
* @return The value of the {@code name} attribute
*/
@Override
public String getName() {
return name;
}
/**
* @return The value of the {@code array} attribute
*/
@Override
public Boolean getArray() {
return array;
}
/**
* @return The value of the {@code context} attribute
*/
@Override
public BodyNode.ContextTypeDef getContext() {
return context;
}
/**
* @return The value of the {@code debugValue} attribute
*/
@Override
public Optional getDebugValue() {
return Optional.ofNullable(debugValue);
}
/**
* @return The value of the {@code formula} attribute
*/
@Override
public Optional getFormula() {
return Optional.ofNullable(formula);
}
/**
* @return The value of the {@code formulaOverAll} attribute
*/
@Override
public Optional getFormulaOverAll() {
return Optional.ofNullable(formulaOverAll);
}
/**
* @return The value of the {@code type} attribute
*/
@Override
public BodyNode.ScalarType getType() {
return type;
}
/**
* Copy the current immutable object by setting a value for the {@link BodyNode.ScalarDef#getToken() token} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for token
* @return A modified copy of the {@code this} object
*/
public final ImmutableScalarDef withToken(HdesNode.Token value) {
if (this.token == value) return this;
HdesNode.Token newValue = Objects.requireNonNull(value, "token");
return new ImmutableScalarDef(
newValue,
this.required,
this.name,
this.array,
this.context,
this.debugValue,
this.formula,
this.formulaOverAll,
this.type);
}
/**
* Copy the current immutable object by setting a value for the {@link BodyNode.ScalarDef#getRequired() required} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for required
* @return A modified copy of the {@code this} object
*/
public final ImmutableScalarDef withRequired(Boolean value) {
Boolean newValue = Objects.requireNonNull(value, "required");
if (this.required.equals(newValue)) return this;
return new ImmutableScalarDef(
this.token,
newValue,
this.name,
this.array,
this.context,
this.debugValue,
this.formula,
this.formulaOverAll,
this.type);
}
/**
* Copy the current immutable object by setting a value for the {@link BodyNode.ScalarDef#getName() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name
* @return A modified copy of the {@code this} object
*/
public final ImmutableScalarDef withName(String value) {
String newValue = Objects.requireNonNull(value, "name");
if (this.name.equals(newValue)) return this;
return new ImmutableScalarDef(
this.token,
this.required,
newValue,
this.array,
this.context,
this.debugValue,
this.formula,
this.formulaOverAll,
this.type);
}
/**
* Copy the current immutable object by setting a value for the {@link BodyNode.ScalarDef#getArray() array} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for array
* @return A modified copy of the {@code this} object
*/
public final ImmutableScalarDef withArray(Boolean value) {
Boolean newValue = Objects.requireNonNull(value, "array");
if (this.array.equals(newValue)) return this;
return new ImmutableScalarDef(
this.token,
this.required,
this.name,
newValue,
this.context,
this.debugValue,
this.formula,
this.formulaOverAll,
this.type);
}
/**
* Copy the current immutable object by setting a value for the {@link BodyNode.ScalarDef#getContext() context} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for context
* @return A modified copy of the {@code this} object
*/
public final ImmutableScalarDef withContext(BodyNode.ContextTypeDef value) {
if (this.context == value) return this;
BodyNode.ContextTypeDef newValue = Objects.requireNonNull(value, "context");
if (this.context.equals(newValue)) return this;
return new ImmutableScalarDef(
this.token,
this.required,
this.name,
this.array,
newValue,
this.debugValue,
this.formula,
this.formulaOverAll,
this.type);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link BodyNode.ScalarDef#getDebugValue() debugValue} attribute.
* @param value The value for debugValue
* @return A modified copy of {@code this} object
*/
public final ImmutableScalarDef withDebugValue(String value) {
@Nullable String newValue = Objects.requireNonNull(value, "debugValue");
if (Objects.equals(this.debugValue, newValue)) return this;
return new ImmutableScalarDef(
this.token,
this.required,
this.name,
this.array,
this.context,
newValue,
this.formula,
this.formulaOverAll,
this.type);
}
/**
* Copy the current immutable object by setting an optional value for the {@link BodyNode.ScalarDef#getDebugValue() debugValue} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for debugValue
* @return A modified copy of {@code this} object
*/
public final ImmutableScalarDef withDebugValue(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.debugValue, value)) return this;
return new ImmutableScalarDef(
this.token,
this.required,
this.name,
this.array,
this.context,
value,
this.formula,
this.formulaOverAll,
this.type);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link BodyNode.ScalarDef#getFormula() formula} attribute.
* @param value The value for formula
* @return A modified copy of {@code this} object
*/
public final ImmutableScalarDef withFormula(ExpressionNode.ExpressionBody value) {
@Nullable ExpressionNode.ExpressionBody newValue = Objects.requireNonNull(value, "formula");
if (this.formula == newValue) return this;
return new ImmutableScalarDef(
this.token,
this.required,
this.name,
this.array,
this.context,
this.debugValue,
newValue,
this.formulaOverAll,
this.type);
}
/**
* Copy the current immutable object by setting an optional value for the {@link BodyNode.ScalarDef#getFormula() formula} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for formula
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final ImmutableScalarDef withFormula(Optional extends ExpressionNode.ExpressionBody> optional) {
@Nullable ExpressionNode.ExpressionBody value = optional.orElse(null);
if (this.formula == value) return this;
return new ImmutableScalarDef(
this.token,
this.required,
this.name,
this.array,
this.context,
this.debugValue,
value,
this.formulaOverAll,
this.type);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link BodyNode.ScalarDef#getFormulaOverAll() formulaOverAll} attribute.
* @param value The value for formulaOverAll
* @return A modified copy of {@code this} object
*/
public final ImmutableScalarDef withFormulaOverAll(boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.formulaOverAll, newValue)) return this;
return new ImmutableScalarDef(
this.token,
this.required,
this.name,
this.array,
this.context,
this.debugValue,
this.formula,
newValue,
this.type);
}
/**
* Copy the current immutable object by setting an optional value for the {@link BodyNode.ScalarDef#getFormulaOverAll() formulaOverAll} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for formulaOverAll
* @return A modified copy of {@code this} object
*/
public final ImmutableScalarDef withFormulaOverAll(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.formulaOverAll, value)) return this;
return new ImmutableScalarDef(
this.token,
this.required,
this.name,
this.array,
this.context,
this.debugValue,
this.formula,
value,
this.type);
}
/**
* Copy the current immutable object by setting a value for the {@link BodyNode.ScalarDef#getType() type} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for type
* @return A modified copy of the {@code this} object
*/
public final ImmutableScalarDef withType(BodyNode.ScalarType value) {
if (this.type == value) return this;
BodyNode.ScalarType newValue = Objects.requireNonNull(value, "type");
if (this.type.equals(newValue)) return this;
return new ImmutableScalarDef(
this.token,
this.required,
this.name,
this.array,
this.context,
this.debugValue,
this.formula,
this.formulaOverAll,
newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableScalarDef} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableScalarDef
&& equalTo((ImmutableScalarDef) another);
}
private boolean equalTo(ImmutableScalarDef another) {
return token.equals(another.token)
&& required.equals(another.required)
&& name.equals(another.name)
&& array.equals(another.array)
&& context.equals(another.context)
&& Objects.equals(debugValue, another.debugValue)
&& Objects.equals(formula, another.formula)
&& Objects.equals(formulaOverAll, another.formulaOverAll)
&& type.equals(another.type);
}
/**
* Computes a hash code from attributes: {@code token}, {@code required}, {@code name}, {@code array}, {@code context}, {@code debugValue}, {@code formula}, {@code formulaOverAll}, {@code type}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + token.hashCode();
h += (h << 5) + required.hashCode();
h += (h << 5) + name.hashCode();
h += (h << 5) + array.hashCode();
h += (h << 5) + context.hashCode();
h += (h << 5) + Objects.hashCode(debugValue);
h += (h << 5) + Objects.hashCode(formula);
h += (h << 5) + Objects.hashCode(formulaOverAll);
h += (h << 5) + type.hashCode();
return h;
}
/**
* Prints the immutable value {@code ScalarDef} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("ScalarDef{");
builder.append("token=").append(token);
builder.append(", ");
builder.append("required=").append(required);
builder.append(", ");
builder.append("name=").append(name);
builder.append(", ");
builder.append("array=").append(array);
builder.append(", ");
builder.append("context=").append(context);
if (debugValue != null) {
builder.append(", ");
builder.append("debugValue=").append(debugValue);
}
if (formula != null) {
builder.append(", ");
builder.append("formula=").append(formula);
}
if (formulaOverAll != null) {
builder.append(", ");
builder.append("formulaOverAll=").append(formulaOverAll);
}
builder.append(", ");
builder.append("type=").append(type);
return builder.append("}").toString();
}
/**
* Creates an immutable copy of a {@link BodyNode.ScalarDef} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable ScalarDef instance
*/
public static ImmutableScalarDef copyOf(BodyNode.ScalarDef instance) {
if (instance instanceof ImmutableScalarDef) {
return (ImmutableScalarDef) instance;
}
return ImmutableScalarDef.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableScalarDef ImmutableScalarDef}.
*
* ImmutableScalarDef.builder()
* .token(io.resys.hdes.ast.api.nodes.HdesNode.Token) // required {@link BodyNode.ScalarDef#getToken() token}
* .required(Boolean) // required {@link BodyNode.ScalarDef#getRequired() required}
* .name(String) // required {@link BodyNode.ScalarDef#getName() name}
* .array(Boolean) // required {@link BodyNode.ScalarDef#getArray() array}
* .context(io.resys.hdes.ast.api.nodes.BodyNode.ContextTypeDef) // required {@link BodyNode.ScalarDef#getContext() context}
* .debugValue(String) // optional {@link BodyNode.ScalarDef#getDebugValue() debugValue}
* .formula(io.resys.hdes.ast.api.nodes.ExpressionNode.ExpressionBody) // optional {@link BodyNode.ScalarDef#getFormula() formula}
* .formulaOverAll(Boolean) // optional {@link BodyNode.ScalarDef#getFormulaOverAll() formulaOverAll}
* .type(io.resys.hdes.ast.api.nodes.BodyNode.ScalarType) // required {@link BodyNode.ScalarDef#getType() type}
* .build();
*
* @return A new ImmutableScalarDef builder
*/
public static ImmutableScalarDef.Builder builder() {
return new ImmutableScalarDef.Builder();
}
/**
* Builds instances of type {@link ImmutableScalarDef ImmutableScalarDef}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "BodyNode.ScalarDef", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_TOKEN = 0x1L;
private static final long INIT_BIT_REQUIRED = 0x2L;
private static final long INIT_BIT_NAME = 0x4L;
private static final long INIT_BIT_ARRAY = 0x8L;
private static final long INIT_BIT_CONTEXT = 0x10L;
private static final long INIT_BIT_TYPE = 0x20L;
private long initBits = 0x3fL;
private @Nullable HdesNode.Token token;
private @Nullable Boolean required;
private @Nullable String name;
private @Nullable Boolean array;
private @Nullable BodyNode.ContextTypeDef context;
private @Nullable String debugValue;
private @Nullable ExpressionNode.ExpressionBody formula;
private @Nullable Boolean formulaOverAll;
private @Nullable BodyNode.ScalarType type;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code io.resys.hdes.ast.api.nodes.HdesNode} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(HdesNode instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code io.resys.hdes.ast.api.nodes.BodyNode.TypeDef} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(BodyNode.TypeDef instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code io.resys.hdes.ast.api.nodes.BodyNode.ScalarDef} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(BodyNode.ScalarDef instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
if (object instanceof HdesNode) {
HdesNode instance = (HdesNode) object;
token(instance.getToken());
}
if (object instanceof BodyNode.TypeDef) {
BodyNode.TypeDef instance = (BodyNode.TypeDef) object;
name(instance.getName());
context(instance.getContext());
array(instance.getArray());
required(instance.getRequired());
}
if (object instanceof BodyNode.ScalarDef) {
BodyNode.ScalarDef instance = (BodyNode.ScalarDef) object;
Optional formulaOverAllOptional = instance.getFormulaOverAll();
if (formulaOverAllOptional.isPresent()) {
formulaOverAll(formulaOverAllOptional);
}
Optional formulaOptional = instance.getFormula();
if (formulaOptional.isPresent()) {
formula(formulaOptional);
}
type(instance.getType());
Optional debugValueOptional = instance.getDebugValue();
if (debugValueOptional.isPresent()) {
debugValue(debugValueOptional);
}
}
}
/**
* Initializes the value for the {@link BodyNode.ScalarDef#getToken() token} attribute.
* @param token The value for token
* @return {@code this} builder for use in a chained invocation
*/
public final Builder token(HdesNode.Token token) {
this.token = Objects.requireNonNull(token, "token");
initBits &= ~INIT_BIT_TOKEN;
return this;
}
/**
* Initializes the value for the {@link BodyNode.ScalarDef#getRequired() required} attribute.
* @param required The value for required
* @return {@code this} builder for use in a chained invocation
*/
public final Builder required(Boolean required) {
this.required = Objects.requireNonNull(required, "required");
initBits &= ~INIT_BIT_REQUIRED;
return this;
}
/**
* Initializes the value for the {@link BodyNode.ScalarDef#getName() name} attribute.
* @param name The value for name
* @return {@code this} builder for use in a chained invocation
*/
public final Builder name(String name) {
this.name = Objects.requireNonNull(name, "name");
initBits &= ~INIT_BIT_NAME;
return this;
}
/**
* Initializes the value for the {@link BodyNode.ScalarDef#getArray() array} attribute.
* @param array The value for array
* @return {@code this} builder for use in a chained invocation
*/
public final Builder array(Boolean array) {
this.array = Objects.requireNonNull(array, "array");
initBits &= ~INIT_BIT_ARRAY;
return this;
}
/**
* Initializes the value for the {@link BodyNode.ScalarDef#getContext() context} attribute.
* @param context The value for context
* @return {@code this} builder for use in a chained invocation
*/
public final Builder context(BodyNode.ContextTypeDef context) {
this.context = Objects.requireNonNull(context, "context");
initBits &= ~INIT_BIT_CONTEXT;
return this;
}
/**
* Initializes the optional value {@link BodyNode.ScalarDef#getDebugValue() debugValue} to debugValue.
* @param debugValue The value for debugValue
* @return {@code this} builder for chained invocation
*/
public final Builder debugValue(String debugValue) {
this.debugValue = Objects.requireNonNull(debugValue, "debugValue");
return this;
}
/**
* Initializes the optional value {@link BodyNode.ScalarDef#getDebugValue() debugValue} to debugValue.
* @param debugValue The value for debugValue
* @return {@code this} builder for use in a chained invocation
*/
public final Builder debugValue(Optional debugValue) {
this.debugValue = debugValue.orElse(null);
return this;
}
/**
* Initializes the optional value {@link BodyNode.ScalarDef#getFormula() formula} to formula.
* @param formula The value for formula
* @return {@code this} builder for chained invocation
*/
public final Builder formula(ExpressionNode.ExpressionBody formula) {
this.formula = Objects.requireNonNull(formula, "formula");
return this;
}
/**
* Initializes the optional value {@link BodyNode.ScalarDef#getFormula() formula} to formula.
* @param formula The value for formula
* @return {@code this} builder for use in a chained invocation
*/
public final Builder formula(Optional extends ExpressionNode.ExpressionBody> formula) {
this.formula = formula.orElse(null);
return this;
}
/**
* Initializes the optional value {@link BodyNode.ScalarDef#getFormulaOverAll() formulaOverAll} to formulaOverAll.
* @param formulaOverAll The value for formulaOverAll
* @return {@code this} builder for chained invocation
*/
public final Builder formulaOverAll(boolean formulaOverAll) {
this.formulaOverAll = formulaOverAll;
return this;
}
/**
* Initializes the optional value {@link BodyNode.ScalarDef#getFormulaOverAll() formulaOverAll} to formulaOverAll.
* @param formulaOverAll The value for formulaOverAll
* @return {@code this} builder for use in a chained invocation
*/
public final Builder formulaOverAll(Optional formulaOverAll) {
this.formulaOverAll = formulaOverAll.orElse(null);
return this;
}
/**
* Initializes the value for the {@link BodyNode.ScalarDef#getType() type} attribute.
* @param type The value for type
* @return {@code this} builder for use in a chained invocation
*/
public final Builder type(BodyNode.ScalarType type) {
this.type = Objects.requireNonNull(type, "type");
initBits &= ~INIT_BIT_TYPE;
return this;
}
/**
* Builds a new {@link ImmutableScalarDef ImmutableScalarDef}.
* @return An immutable instance of ScalarDef
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableScalarDef build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableScalarDef(token, required, name, array, context, debugValue, formula, formulaOverAll, type);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_TOKEN) != 0) attributes.add("token");
if ((initBits & INIT_BIT_REQUIRED) != 0) attributes.add("required");
if ((initBits & INIT_BIT_NAME) != 0) attributes.add("name");
if ((initBits & INIT_BIT_ARRAY) != 0) attributes.add("array");
if ((initBits & INIT_BIT_CONTEXT) != 0) attributes.add("context");
if ((initBits & INIT_BIT_TYPE) != 0) attributes.add("type");
return "Cannot build ScalarDef, some of required attributes are not set " + attributes;
}
}
}