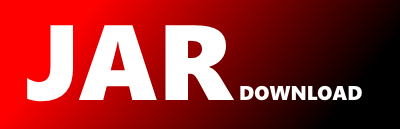
io.resys.hdes.client.api.ast.ImmutableAstDecision Maven / Gradle / Ivy
package io.resys.hdes.client.api.ast;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Maps;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link AstDecision}.
*
* Use the builder to create immutable instances:
* {@code ImmutableAstDecision.builder()}.
*/
@Generated(from = "AstDecision", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableAstDecision implements AstDecision {
private final String name;
private final @Nullable String description;
private final AstBody.Headers headers;
private final AstBody.AstBodyType bodyType;
private final ImmutableList messages;
private final ImmutableList headerTypes;
private final ImmutableMap> headerExpressions;
private final AstDecision.HitPolicy hitPolicy;
private final ImmutableList rows;
private ImmutableAstDecision(
String name,
@Nullable String description,
AstBody.Headers headers,
AstBody.AstBodyType bodyType,
ImmutableList messages,
ImmutableList headerTypes,
ImmutableMap> headerExpressions,
AstDecision.HitPolicy hitPolicy,
ImmutableList rows) {
this.name = name;
this.description = description;
this.headers = headers;
this.bodyType = bodyType;
this.messages = messages;
this.headerTypes = headerTypes;
this.headerExpressions = headerExpressions;
this.hitPolicy = hitPolicy;
this.rows = rows;
}
/**
* @return The value of the {@code name} attribute
*/
@JsonProperty("name")
@Override
public String getName() {
return name;
}
/**
* @return The value of the {@code description} attribute
*/
@JsonProperty("description")
@Override
public @Nullable String getDescription() {
return description;
}
/**
* @return The value of the {@code headers} attribute
*/
@JsonProperty("headers")
@Override
public AstBody.Headers getHeaders() {
return headers;
}
/**
* @return The value of the {@code bodyType} attribute
*/
@JsonProperty("bodyType")
@Override
public AstBody.AstBodyType getBodyType() {
return bodyType;
}
/**
* @return The value of the {@code messages} attribute
*/
@JsonProperty("messages")
@Override
public ImmutableList getMessages() {
return messages;
}
/**
* @return The value of the {@code headerTypes} attribute
*/
@JsonProperty("headerTypes")
@Override
public ImmutableList getHeaderTypes() {
return headerTypes;
}
/**
* @return The value of the {@code headerExpressions} attribute
*/
@JsonProperty("headerExpressions")
@Override
public ImmutableMap> getHeaderExpressions() {
return headerExpressions;
}
/**
* @return The value of the {@code hitPolicy} attribute
*/
@JsonProperty("hitPolicy")
@Override
public AstDecision.HitPolicy getHitPolicy() {
return hitPolicy;
}
/**
* @return The value of the {@code rows} attribute
*/
@JsonProperty("rows")
@Override
public ImmutableList getRows() {
return rows;
}
/**
* Copy the current immutable object by setting a value for the {@link AstDecision#getName() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name
* @return A modified copy of the {@code this} object
*/
public final ImmutableAstDecision withName(String value) {
String newValue = Objects.requireNonNull(value, "name");
if (this.name.equals(newValue)) return this;
return new ImmutableAstDecision(
newValue,
this.description,
this.headers,
this.bodyType,
this.messages,
this.headerTypes,
this.headerExpressions,
this.hitPolicy,
this.rows);
}
/**
* Copy the current immutable object by setting a value for the {@link AstDecision#getDescription() description} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for description (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableAstDecision withDescription(@Nullable String value) {
if (Objects.equals(this.description, value)) return this;
return new ImmutableAstDecision(
this.name,
value,
this.headers,
this.bodyType,
this.messages,
this.headerTypes,
this.headerExpressions,
this.hitPolicy,
this.rows);
}
/**
* Copy the current immutable object by setting a value for the {@link AstDecision#getHeaders() headers} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for headers
* @return A modified copy of the {@code this} object
*/
public final ImmutableAstDecision withHeaders(AstBody.Headers value) {
if (this.headers == value) return this;
AstBody.Headers newValue = Objects.requireNonNull(value, "headers");
return new ImmutableAstDecision(
this.name,
this.description,
newValue,
this.bodyType,
this.messages,
this.headerTypes,
this.headerExpressions,
this.hitPolicy,
this.rows);
}
/**
* Copy the current immutable object by setting a value for the {@link AstDecision#getBodyType() bodyType} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for bodyType
* @return A modified copy of the {@code this} object
*/
public final ImmutableAstDecision withBodyType(AstBody.AstBodyType value) {
if (this.bodyType == value) return this;
AstBody.AstBodyType newValue = Objects.requireNonNull(value, "bodyType");
if (this.bodyType.equals(newValue)) return this;
return new ImmutableAstDecision(
this.name,
this.description,
this.headers,
newValue,
this.messages,
this.headerTypes,
this.headerExpressions,
this.hitPolicy,
this.rows);
}
/**
* Copy the current immutable object with elements that replace the content of {@link AstDecision#getMessages() messages}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableAstDecision withMessages(AstBody.AstCommandMessage... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableAstDecision(
this.name,
this.description,
this.headers,
this.bodyType,
newValue,
this.headerTypes,
this.headerExpressions,
this.hitPolicy,
this.rows);
}
/**
* Copy the current immutable object with elements that replace the content of {@link AstDecision#getMessages() messages}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of messages elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableAstDecision withMessages(Iterable extends AstBody.AstCommandMessage> elements) {
if (this.messages == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableAstDecision(
this.name,
this.description,
this.headers,
this.bodyType,
newValue,
this.headerTypes,
this.headerExpressions,
this.hitPolicy,
this.rows);
}
/**
* Copy the current immutable object with elements that replace the content of {@link AstDecision#getHeaderTypes() headerTypes}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableAstDecision withHeaderTypes(String... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableAstDecision(
this.name,
this.description,
this.headers,
this.bodyType,
this.messages,
newValue,
this.headerExpressions,
this.hitPolicy,
this.rows);
}
/**
* Copy the current immutable object with elements that replace the content of {@link AstDecision#getHeaderTypes() headerTypes}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of headerTypes elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableAstDecision withHeaderTypes(Iterable elements) {
if (this.headerTypes == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableAstDecision(
this.name,
this.description,
this.headers,
this.bodyType,
this.messages,
newValue,
this.headerExpressions,
this.hitPolicy,
this.rows);
}
/**
* Copy the current immutable object by replacing the {@link AstDecision#getHeaderExpressions() headerExpressions} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the headerExpressions map
* @return A modified copy of {@code this} object
*/
public final ImmutableAstDecision withHeaderExpressions(Map> entries) {
if (this.headerExpressions == entries) return this;
ImmutableMap> newValue = Maps.immutableEnumMap(entries);
return new ImmutableAstDecision(
this.name,
this.description,
this.headers,
this.bodyType,
this.messages,
this.headerTypes,
newValue,
this.hitPolicy,
this.rows);
}
/**
* Copy the current immutable object by setting a value for the {@link AstDecision#getHitPolicy() hitPolicy} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for hitPolicy
* @return A modified copy of the {@code this} object
*/
public final ImmutableAstDecision withHitPolicy(AstDecision.HitPolicy value) {
if (this.hitPolicy == value) return this;
AstDecision.HitPolicy newValue = Objects.requireNonNull(value, "hitPolicy");
if (this.hitPolicy.equals(newValue)) return this;
return new ImmutableAstDecision(
this.name,
this.description,
this.headers,
this.bodyType,
this.messages,
this.headerTypes,
this.headerExpressions,
newValue,
this.rows);
}
/**
* Copy the current immutable object with elements that replace the content of {@link AstDecision#getRows() rows}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableAstDecision withRows(AstDecision.AstDecisionRow... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableAstDecision(
this.name,
this.description,
this.headers,
this.bodyType,
this.messages,
this.headerTypes,
this.headerExpressions,
this.hitPolicy,
newValue);
}
/**
* Copy the current immutable object with elements that replace the content of {@link AstDecision#getRows() rows}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of rows elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableAstDecision withRows(Iterable extends AstDecision.AstDecisionRow> elements) {
if (this.rows == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableAstDecision(
this.name,
this.description,
this.headers,
this.bodyType,
this.messages,
this.headerTypes,
this.headerExpressions,
this.hitPolicy,
newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableAstDecision} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableAstDecision
&& equalTo((ImmutableAstDecision) another);
}
private boolean equalTo(ImmutableAstDecision another) {
return name.equals(another.name)
&& Objects.equals(description, another.description)
&& headers.equals(another.headers)
&& bodyType.equals(another.bodyType)
&& messages.equals(another.messages)
&& headerTypes.equals(another.headerTypes)
&& headerExpressions.equals(another.headerExpressions)
&& hitPolicy.equals(another.hitPolicy)
&& rows.equals(another.rows);
}
/**
* Computes a hash code from attributes: {@code name}, {@code description}, {@code headers}, {@code bodyType}, {@code messages}, {@code headerTypes}, {@code headerExpressions}, {@code hitPolicy}, {@code rows}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + name.hashCode();
h += (h << 5) + Objects.hashCode(description);
h += (h << 5) + headers.hashCode();
h += (h << 5) + bodyType.hashCode();
h += (h << 5) + messages.hashCode();
h += (h << 5) + headerTypes.hashCode();
h += (h << 5) + headerExpressions.hashCode();
h += (h << 5) + hitPolicy.hashCode();
h += (h << 5) + rows.hashCode();
return h;
}
/**
* Prints the immutable value {@code AstDecision} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("AstDecision")
.omitNullValues()
.add("name", name)
.add("description", description)
.add("headers", headers)
.add("bodyType", bodyType)
.add("messages", messages)
.add("headerTypes", headerTypes)
.add("headerExpressions", headerExpressions)
.add("hitPolicy", hitPolicy)
.add("rows", rows)
.toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "AstDecision", generator = "Immutables")
@Deprecated
@SuppressWarnings("Immutable")
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements AstDecision {
@Nullable String name;
@Nullable String description;
@Nullable AstBody.Headers headers;
@Nullable AstBody.AstBodyType bodyType;
@Nullable List messages = ImmutableList.of();
@Nullable List headerTypes = ImmutableList.of();
@Nullable Map> headerExpressions = ImmutableMap.of();
@Nullable AstDecision.HitPolicy hitPolicy;
@Nullable List rows = ImmutableList.of();
@JsonProperty("name")
public void setName(String name) {
this.name = name;
}
@JsonProperty("description")
public void setDescription(@Nullable String description) {
this.description = description;
}
@JsonProperty("headers")
public void setHeaders(AstBody.Headers headers) {
this.headers = headers;
}
@JsonProperty("bodyType")
public void setBodyType(AstBody.AstBodyType bodyType) {
this.bodyType = bodyType;
}
@JsonProperty("messages")
public void setMessages(List messages) {
this.messages = messages;
}
@JsonProperty("headerTypes")
public void setHeaderTypes(List headerTypes) {
this.headerTypes = headerTypes;
}
@JsonProperty("headerExpressions")
public void setHeaderExpressions(Map> headerExpressions) {
this.headerExpressions = headerExpressions;
}
@JsonProperty("hitPolicy")
public void setHitPolicy(AstDecision.HitPolicy hitPolicy) {
this.hitPolicy = hitPolicy;
}
@JsonProperty("rows")
public void setRows(List rows) {
this.rows = rows;
}
@Override
public String getName() { throw new UnsupportedOperationException(); }
@Override
public String getDescription() { throw new UnsupportedOperationException(); }
@Override
public AstBody.Headers getHeaders() { throw new UnsupportedOperationException(); }
@Override
public AstBody.AstBodyType getBodyType() { throw new UnsupportedOperationException(); }
@Override
public List getMessages() { throw new UnsupportedOperationException(); }
@Override
public List getHeaderTypes() { throw new UnsupportedOperationException(); }
@Override
public Map> getHeaderExpressions() { throw new UnsupportedOperationException(); }
@Override
public AstDecision.HitPolicy getHitPolicy() { throw new UnsupportedOperationException(); }
@Override
public List getRows() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableAstDecision fromJson(Json json) {
ImmutableAstDecision.Builder builder = ImmutableAstDecision.builder();
if (json.name != null) {
builder.name(json.name);
}
if (json.description != null) {
builder.description(json.description);
}
if (json.headers != null) {
builder.headers(json.headers);
}
if (json.bodyType != null) {
builder.bodyType(json.bodyType);
}
if (json.messages != null) {
builder.addAllMessages(json.messages);
}
if (json.headerTypes != null) {
builder.addAllHeaderTypes(json.headerTypes);
}
if (json.headerExpressions != null) {
builder.putAllHeaderExpressions(json.headerExpressions);
}
if (json.hitPolicy != null) {
builder.hitPolicy(json.hitPolicy);
}
if (json.rows != null) {
builder.addAllRows(json.rows);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link AstDecision} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable AstDecision instance
*/
public static ImmutableAstDecision copyOf(AstDecision instance) {
if (instance instanceof ImmutableAstDecision) {
return (ImmutableAstDecision) instance;
}
return ImmutableAstDecision.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableAstDecision ImmutableAstDecision}.
*
* ImmutableAstDecision.builder()
* .name(String) // required {@link AstDecision#getName() name}
* .description(String | null) // nullable {@link AstDecision#getDescription() description}
* .headers(io.resys.hdes.client.api.ast.AstBody.Headers) // required {@link AstDecision#getHeaders() headers}
* .bodyType(io.resys.hdes.client.api.ast.AstBody.AstBodyType) // required {@link AstDecision#getBodyType() bodyType}
* .addMessages|addAllMessages(io.resys.hdes.client.api.ast.AstBody.AstCommandMessage) // {@link AstDecision#getMessages() messages} elements
* .addHeaderTypes|addAllHeaderTypes(String) // {@link AstDecision#getHeaderTypes() headerTypes} elements
* .putHeaderExpressions|putAllHeaderExpressions(io.resys.hdes.client.api.ast.TypeDef.ValueType => List<String>) // {@link AstDecision#getHeaderExpressions() headerExpressions} mappings
* .hitPolicy(io.resys.hdes.client.api.ast.AstDecision.HitPolicy) // required {@link AstDecision#getHitPolicy() hitPolicy}
* .addRows|addAllRows(io.resys.hdes.client.api.ast.AstDecision.AstDecisionRow) // {@link AstDecision#getRows() rows} elements
* .build();
*
* @return A new ImmutableAstDecision builder
*/
public static ImmutableAstDecision.Builder builder() {
return new ImmutableAstDecision.Builder();
}
/**
* Builds instances of type {@link ImmutableAstDecision ImmutableAstDecision}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "AstDecision", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_NAME = 0x1L;
private static final long INIT_BIT_HEADERS = 0x2L;
private static final long INIT_BIT_BODY_TYPE = 0x4L;
private static final long INIT_BIT_HIT_POLICY = 0x8L;
private long initBits = 0xfL;
private @Nullable String name;
private @Nullable String description;
private @Nullable AstBody.Headers headers;
private @Nullable AstBody.AstBodyType bodyType;
private ImmutableList.Builder messages = ImmutableList.builder();
private ImmutableList.Builder headerTypes = ImmutableList.builder();
private ImmutableMap.Builder> headerExpressions = ImmutableMap.builder();
private @Nullable AstDecision.HitPolicy hitPolicy;
private ImmutableList.Builder rows = ImmutableList.builder();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code io.resys.hdes.client.api.ast.AstDecision} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(AstDecision instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code io.resys.hdes.client.api.ast.AstBody} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(AstBody instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
if (object instanceof AstDecision) {
AstDecision instance = (AstDecision) object;
addAllHeaderTypes(instance.getHeaderTypes());
hitPolicy(instance.getHitPolicy());
putAllHeaderExpressions(instance.getHeaderExpressions());
addAllRows(instance.getRows());
}
if (object instanceof AstBody) {
AstBody instance = (AstBody) object;
bodyType(instance.getBodyType());
name(instance.getName());
headers(instance.getHeaders());
@Nullable String descriptionValue = instance.getDescription();
if (descriptionValue != null) {
description(descriptionValue);
}
addAllMessages(instance.getMessages());
}
}
/**
* Initializes the value for the {@link AstDecision#getName() name} attribute.
* @param name The value for name
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("name")
public final Builder name(String name) {
this.name = Objects.requireNonNull(name, "name");
initBits &= ~INIT_BIT_NAME;
return this;
}
/**
* Initializes the value for the {@link AstDecision#getDescription() description} attribute.
* @param description The value for description (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("description")
public final Builder description(@Nullable String description) {
this.description = description;
return this;
}
/**
* Initializes the value for the {@link AstDecision#getHeaders() headers} attribute.
* @param headers The value for headers
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("headers")
public final Builder headers(AstBody.Headers headers) {
this.headers = Objects.requireNonNull(headers, "headers");
initBits &= ~INIT_BIT_HEADERS;
return this;
}
/**
* Initializes the value for the {@link AstDecision#getBodyType() bodyType} attribute.
* @param bodyType The value for bodyType
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("bodyType")
public final Builder bodyType(AstBody.AstBodyType bodyType) {
this.bodyType = Objects.requireNonNull(bodyType, "bodyType");
initBits &= ~INIT_BIT_BODY_TYPE;
return this;
}
/**
* Adds one element to {@link AstDecision#getMessages() messages} list.
* @param element A messages element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addMessages(AstBody.AstCommandMessage element) {
this.messages.add(element);
return this;
}
/**
* Adds elements to {@link AstDecision#getMessages() messages} list.
* @param elements An array of messages elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addMessages(AstBody.AstCommandMessage... elements) {
this.messages.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link AstDecision#getMessages() messages} list.
* @param elements An iterable of messages elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("messages")
public final Builder messages(Iterable extends AstBody.AstCommandMessage> elements) {
this.messages = ImmutableList.builder();
return addAllMessages(elements);
}
/**
* Adds elements to {@link AstDecision#getMessages() messages} list.
* @param elements An iterable of messages elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllMessages(Iterable extends AstBody.AstCommandMessage> elements) {
this.messages.addAll(elements);
return this;
}
/**
* Adds one element to {@link AstDecision#getHeaderTypes() headerTypes} list.
* @param element A headerTypes element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addHeaderTypes(String element) {
this.headerTypes.add(element);
return this;
}
/**
* Adds elements to {@link AstDecision#getHeaderTypes() headerTypes} list.
* @param elements An array of headerTypes elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addHeaderTypes(String... elements) {
this.headerTypes.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link AstDecision#getHeaderTypes() headerTypes} list.
* @param elements An iterable of headerTypes elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("headerTypes")
public final Builder headerTypes(Iterable elements) {
this.headerTypes = ImmutableList.builder();
return addAllHeaderTypes(elements);
}
/**
* Adds elements to {@link AstDecision#getHeaderTypes() headerTypes} list.
* @param elements An iterable of headerTypes elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllHeaderTypes(Iterable elements) {
this.headerTypes.addAll(elements);
return this;
}
/**
* Put one entry to the {@link AstDecision#getHeaderExpressions() headerExpressions} map.
* @param key The key in the headerExpressions map
* @param value The associated value in the headerExpressions map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putHeaderExpressions(TypeDef.ValueType key, List value) {
this.headerExpressions.put(key, value);
return this;
}
/**
* Put one entry to the {@link AstDecision#getHeaderExpressions() headerExpressions} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putHeaderExpressions(Map.Entry> entry) {
this.headerExpressions.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link AstDecision#getHeaderExpressions() headerExpressions} map. Nulls are not permitted
* @param entries The entries that will be added to the headerExpressions map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("headerExpressions")
public final Builder headerExpressions(Map> entries) {
this.headerExpressions = ImmutableMap.builder();
return putAllHeaderExpressions(entries);
}
/**
* Put all mappings from the specified map as entries to {@link AstDecision#getHeaderExpressions() headerExpressions} map. Nulls are not permitted
* @param entries The entries that will be added to the headerExpressions map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllHeaderExpressions(Map> entries) {
this.headerExpressions.putAll(entries);
return this;
}
/**
* Initializes the value for the {@link AstDecision#getHitPolicy() hitPolicy} attribute.
* @param hitPolicy The value for hitPolicy
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("hitPolicy")
public final Builder hitPolicy(AstDecision.HitPolicy hitPolicy) {
this.hitPolicy = Objects.requireNonNull(hitPolicy, "hitPolicy");
initBits &= ~INIT_BIT_HIT_POLICY;
return this;
}
/**
* Adds one element to {@link AstDecision#getRows() rows} list.
* @param element A rows element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addRows(AstDecision.AstDecisionRow element) {
this.rows.add(element);
return this;
}
/**
* Adds elements to {@link AstDecision#getRows() rows} list.
* @param elements An array of rows elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addRows(AstDecision.AstDecisionRow... elements) {
this.rows.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link AstDecision#getRows() rows} list.
* @param elements An iterable of rows elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("rows")
public final Builder rows(Iterable extends AstDecision.AstDecisionRow> elements) {
this.rows = ImmutableList.builder();
return addAllRows(elements);
}
/**
* Adds elements to {@link AstDecision#getRows() rows} list.
* @param elements An iterable of rows elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllRows(Iterable extends AstDecision.AstDecisionRow> elements) {
this.rows.addAll(elements);
return this;
}
/**
* Builds a new {@link ImmutableAstDecision ImmutableAstDecision}.
* @return An immutable instance of AstDecision
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableAstDecision build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableAstDecision(
name,
description,
headers,
bodyType,
messages.build(),
headerTypes.build(),
Maps.immutableEnumMap(headerExpressions.build()),
hitPolicy,
rows.build());
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_NAME) != 0) attributes.add("name");
if ((initBits & INIT_BIT_HEADERS) != 0) attributes.add("headers");
if ((initBits & INIT_BIT_BODY_TYPE) != 0) attributes.add("bodyType");
if ((initBits & INIT_BIT_HIT_POLICY) != 0) attributes.add("hitPolicy");
return "Cannot build AstDecision, some of required attributes are not set " + attributes;
}
}
}