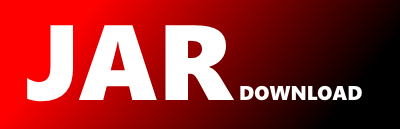
io.resys.hdes.client.api.programs.ImmutableFlowResultLog Maven / Gradle / Ivy
package io.resys.hdes.client.api.programs;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.io.Serializable;
import java.time.LocalDateTime;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link FlowProgram.FlowResultLog}.
*
* Use the builder to create immutable instances:
* {@code ImmutableFlowResultLog.builder()}.
*/
@Generated(from = "FlowProgram.FlowResultLog", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableFlowResultLog
implements FlowProgram.FlowResultLog {
private final Integer id;
private final String stepId;
private final LocalDateTime start;
private final LocalDateTime end;
private final ImmutableList errors;
private final FlowProgram.FlowExecutionStatus status;
private final ImmutableMap accepts;
private final ImmutableMap returns;
private final @Nullable Serializable returnsValue;
private ImmutableFlowResultLog(
Integer id,
String stepId,
LocalDateTime start,
LocalDateTime end,
ImmutableList errors,
FlowProgram.FlowExecutionStatus status,
ImmutableMap accepts,
ImmutableMap returns,
@Nullable Serializable returnsValue) {
this.id = id;
this.stepId = stepId;
this.start = start;
this.end = end;
this.errors = errors;
this.status = status;
this.accepts = accepts;
this.returns = returns;
this.returnsValue = returnsValue;
}
/**
* @return The value of the {@code id} attribute
*/
@Override
public Integer getId() {
return id;
}
/**
* @return The value of the {@code stepId} attribute
*/
@Override
public String getStepId() {
return stepId;
}
/**
* @return The value of the {@code start} attribute
*/
@Override
public LocalDateTime getStart() {
return start;
}
/**
* @return The value of the {@code end} attribute
*/
@Override
public LocalDateTime getEnd() {
return end;
}
/**
* @return The value of the {@code errors} attribute
*/
@Override
public ImmutableList getErrors() {
return errors;
}
/**
* @return The value of the {@code status} attribute
*/
@Override
public FlowProgram.FlowExecutionStatus getStatus() {
return status;
}
/**
* @return The value of the {@code accepts} attribute
*/
@Override
public ImmutableMap getAccepts() {
return accepts;
}
/**
* @return The value of the {@code returns} attribute
*/
@Override
public ImmutableMap getReturns() {
return returns;
}
/**
* @return The value of the {@code returnsValue} attribute
*/
@Override
public @Nullable Serializable getReturnsValue() {
return returnsValue;
}
/**
* Copy the current immutable object by setting a value for the {@link FlowProgram.FlowResultLog#getId() id} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for id
* @return A modified copy of the {@code this} object
*/
public final ImmutableFlowResultLog withId(Integer value) {
Integer newValue = Objects.requireNonNull(value, "id");
if (this.id.equals(newValue)) return this;
return new ImmutableFlowResultLog(
newValue,
this.stepId,
this.start,
this.end,
this.errors,
this.status,
this.accepts,
this.returns,
this.returnsValue);
}
/**
* Copy the current immutable object by setting a value for the {@link FlowProgram.FlowResultLog#getStepId() stepId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for stepId
* @return A modified copy of the {@code this} object
*/
public final ImmutableFlowResultLog withStepId(String value) {
String newValue = Objects.requireNonNull(value, "stepId");
if (this.stepId.equals(newValue)) return this;
return new ImmutableFlowResultLog(
this.id,
newValue,
this.start,
this.end,
this.errors,
this.status,
this.accepts,
this.returns,
this.returnsValue);
}
/**
* Copy the current immutable object by setting a value for the {@link FlowProgram.FlowResultLog#getStart() start} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for start
* @return A modified copy of the {@code this} object
*/
public final ImmutableFlowResultLog withStart(LocalDateTime value) {
if (this.start == value) return this;
LocalDateTime newValue = Objects.requireNonNull(value, "start");
return new ImmutableFlowResultLog(
this.id,
this.stepId,
newValue,
this.end,
this.errors,
this.status,
this.accepts,
this.returns,
this.returnsValue);
}
/**
* Copy the current immutable object by setting a value for the {@link FlowProgram.FlowResultLog#getEnd() end} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for end
* @return A modified copy of the {@code this} object
*/
public final ImmutableFlowResultLog withEnd(LocalDateTime value) {
if (this.end == value) return this;
LocalDateTime newValue = Objects.requireNonNull(value, "end");
return new ImmutableFlowResultLog(
this.id,
this.stepId,
this.start,
newValue,
this.errors,
this.status,
this.accepts,
this.returns,
this.returnsValue);
}
/**
* Copy the current immutable object with elements that replace the content of {@link FlowProgram.FlowResultLog#getErrors() errors}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableFlowResultLog withErrors(FlowProgram.FlowResultErrorLog... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableFlowResultLog(
this.id,
this.stepId,
this.start,
this.end,
newValue,
this.status,
this.accepts,
this.returns,
this.returnsValue);
}
/**
* Copy the current immutable object with elements that replace the content of {@link FlowProgram.FlowResultLog#getErrors() errors}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of errors elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableFlowResultLog withErrors(Iterable extends FlowProgram.FlowResultErrorLog> elements) {
if (this.errors == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableFlowResultLog(
this.id,
this.stepId,
this.start,
this.end,
newValue,
this.status,
this.accepts,
this.returns,
this.returnsValue);
}
/**
* Copy the current immutable object by setting a value for the {@link FlowProgram.FlowResultLog#getStatus() status} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for status
* @return A modified copy of the {@code this} object
*/
public final ImmutableFlowResultLog withStatus(FlowProgram.FlowExecutionStatus value) {
if (this.status == value) return this;
FlowProgram.FlowExecutionStatus newValue = Objects.requireNonNull(value, "status");
if (this.status.equals(newValue)) return this;
return new ImmutableFlowResultLog(
this.id,
this.stepId,
this.start,
this.end,
this.errors,
newValue,
this.accepts,
this.returns,
this.returnsValue);
}
/**
* Copy the current immutable object by replacing the {@link FlowProgram.FlowResultLog#getAccepts() accepts} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the accepts map
* @return A modified copy of {@code this} object
*/
public final ImmutableFlowResultLog withAccepts(Map entries) {
if (this.accepts == entries) return this;
ImmutableMap newValue = ImmutableMap.copyOf(entries);
return new ImmutableFlowResultLog(
this.id,
this.stepId,
this.start,
this.end,
this.errors,
this.status,
newValue,
this.returns,
this.returnsValue);
}
/**
* Copy the current immutable object by replacing the {@link FlowProgram.FlowResultLog#getReturns() returns} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the returns map
* @return A modified copy of {@code this} object
*/
public final ImmutableFlowResultLog withReturns(Map entries) {
if (this.returns == entries) return this;
ImmutableMap newValue = ImmutableMap.copyOf(entries);
return new ImmutableFlowResultLog(
this.id,
this.stepId,
this.start,
this.end,
this.errors,
this.status,
this.accepts,
newValue,
this.returnsValue);
}
/**
* Copy the current immutable object by setting a value for the {@link FlowProgram.FlowResultLog#getReturnsValue() returnsValue} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for returnsValue (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableFlowResultLog withReturnsValue(@Nullable Serializable value) {
if (this.returnsValue == value) return this;
return new ImmutableFlowResultLog(
this.id,
this.stepId,
this.start,
this.end,
this.errors,
this.status,
this.accepts,
this.returns,
value);
}
/**
* This instance is equal to all instances of {@code ImmutableFlowResultLog} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableFlowResultLog
&& equalTo((ImmutableFlowResultLog) another);
}
private boolean equalTo(ImmutableFlowResultLog another) {
return id.equals(another.id)
&& stepId.equals(another.stepId)
&& start.equals(another.start)
&& end.equals(another.end)
&& errors.equals(another.errors)
&& status.equals(another.status)
&& accepts.equals(another.accepts)
&& returns.equals(another.returns)
&& Objects.equals(returnsValue, another.returnsValue);
}
/**
* Computes a hash code from attributes: {@code id}, {@code stepId}, {@code start}, {@code end}, {@code errors}, {@code status}, {@code accepts}, {@code returns}, {@code returnsValue}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + id.hashCode();
h += (h << 5) + stepId.hashCode();
h += (h << 5) + start.hashCode();
h += (h << 5) + end.hashCode();
h += (h << 5) + errors.hashCode();
h += (h << 5) + status.hashCode();
h += (h << 5) + accepts.hashCode();
h += (h << 5) + returns.hashCode();
h += (h << 5) + Objects.hashCode(returnsValue);
return h;
}
/**
* Prints the immutable value {@code FlowResultLog} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("FlowResultLog")
.omitNullValues()
.add("id", id)
.add("stepId", stepId)
.add("start", start)
.add("end", end)
.add("errors", errors)
.add("status", status)
.add("accepts", accepts)
.add("returns", returns)
.add("returnsValue", returnsValue)
.toString();
}
/**
* Creates an immutable copy of a {@link FlowProgram.FlowResultLog} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable FlowResultLog instance
*/
public static ImmutableFlowResultLog copyOf(FlowProgram.FlowResultLog instance) {
if (instance instanceof ImmutableFlowResultLog) {
return (ImmutableFlowResultLog) instance;
}
return ImmutableFlowResultLog.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableFlowResultLog ImmutableFlowResultLog}.
*
* ImmutableFlowResultLog.builder()
* .id(Integer) // required {@link FlowProgram.FlowResultLog#getId() id}
* .stepId(String) // required {@link FlowProgram.FlowResultLog#getStepId() stepId}
* .start(java.time.LocalDateTime) // required {@link FlowProgram.FlowResultLog#getStart() start}
* .end(java.time.LocalDateTime) // required {@link FlowProgram.FlowResultLog#getEnd() end}
* .addErrors|addAllErrors(io.resys.hdes.client.api.programs.FlowProgram.FlowResultErrorLog) // {@link FlowProgram.FlowResultLog#getErrors() errors} elements
* .status(io.resys.hdes.client.api.programs.FlowProgram.FlowExecutionStatus) // required {@link FlowProgram.FlowResultLog#getStatus() status}
* .putAccepts|putAllAccepts(String => java.io.Serializable) // {@link FlowProgram.FlowResultLog#getAccepts() accepts} mappings
* .putReturns|putAllReturns(String => java.io.Serializable) // {@link FlowProgram.FlowResultLog#getReturns() returns} mappings
* .returnsValue(java.io.Serializable | null) // nullable {@link FlowProgram.FlowResultLog#getReturnsValue() returnsValue}
* .build();
*
* @return A new ImmutableFlowResultLog builder
*/
public static ImmutableFlowResultLog.Builder builder() {
return new ImmutableFlowResultLog.Builder();
}
/**
* Builds instances of type {@link ImmutableFlowResultLog ImmutableFlowResultLog}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "FlowProgram.FlowResultLog", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_ID = 0x1L;
private static final long INIT_BIT_STEP_ID = 0x2L;
private static final long INIT_BIT_START = 0x4L;
private static final long INIT_BIT_END = 0x8L;
private static final long INIT_BIT_STATUS = 0x10L;
private long initBits = 0x1fL;
private @Nullable Integer id;
private @Nullable String stepId;
private @Nullable LocalDateTime start;
private @Nullable LocalDateTime end;
private ImmutableList.Builder errors = ImmutableList.builder();
private @Nullable FlowProgram.FlowExecutionStatus status;
private ImmutableMap.Builder accepts = ImmutableMap.builder();
private ImmutableMap.Builder returns = ImmutableMap.builder();
private @Nullable Serializable returnsValue;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code FlowResultLog} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(FlowProgram.FlowResultLog instance) {
Objects.requireNonNull(instance, "instance");
id(instance.getId());
stepId(instance.getStepId());
start(instance.getStart());
end(instance.getEnd());
addAllErrors(instance.getErrors());
status(instance.getStatus());
putAllAccepts(instance.getAccepts());
putAllReturns(instance.getReturns());
@Nullable Serializable returnsValueValue = instance.getReturnsValue();
if (returnsValueValue != null) {
returnsValue(returnsValueValue);
}
return this;
}
/**
* Initializes the value for the {@link FlowProgram.FlowResultLog#getId() id} attribute.
* @param id The value for id
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder id(Integer id) {
this.id = Objects.requireNonNull(id, "id");
initBits &= ~INIT_BIT_ID;
return this;
}
/**
* Initializes the value for the {@link FlowProgram.FlowResultLog#getStepId() stepId} attribute.
* @param stepId The value for stepId
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder stepId(String stepId) {
this.stepId = Objects.requireNonNull(stepId, "stepId");
initBits &= ~INIT_BIT_STEP_ID;
return this;
}
/**
* Initializes the value for the {@link FlowProgram.FlowResultLog#getStart() start} attribute.
* @param start The value for start
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder start(LocalDateTime start) {
this.start = Objects.requireNonNull(start, "start");
initBits &= ~INIT_BIT_START;
return this;
}
/**
* Initializes the value for the {@link FlowProgram.FlowResultLog#getEnd() end} attribute.
* @param end The value for end
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder end(LocalDateTime end) {
this.end = Objects.requireNonNull(end, "end");
initBits &= ~INIT_BIT_END;
return this;
}
/**
* Adds one element to {@link FlowProgram.FlowResultLog#getErrors() errors} list.
* @param element A errors element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addErrors(FlowProgram.FlowResultErrorLog element) {
this.errors.add(element);
return this;
}
/**
* Adds elements to {@link FlowProgram.FlowResultLog#getErrors() errors} list.
* @param elements An array of errors elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addErrors(FlowProgram.FlowResultErrorLog... elements) {
this.errors.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link FlowProgram.FlowResultLog#getErrors() errors} list.
* @param elements An iterable of errors elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder errors(Iterable extends FlowProgram.FlowResultErrorLog> elements) {
this.errors = ImmutableList.builder();
return addAllErrors(elements);
}
/**
* Adds elements to {@link FlowProgram.FlowResultLog#getErrors() errors} list.
* @param elements An iterable of errors elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllErrors(Iterable extends FlowProgram.FlowResultErrorLog> elements) {
this.errors.addAll(elements);
return this;
}
/**
* Initializes the value for the {@link FlowProgram.FlowResultLog#getStatus() status} attribute.
* @param status The value for status
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder status(FlowProgram.FlowExecutionStatus status) {
this.status = Objects.requireNonNull(status, "status");
initBits &= ~INIT_BIT_STATUS;
return this;
}
/**
* Put one entry to the {@link FlowProgram.FlowResultLog#getAccepts() accepts} map.
* @param key The key in the accepts map
* @param value The associated value in the accepts map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAccepts(String key, Serializable value) {
this.accepts.put(key, value);
return this;
}
/**
* Put one entry to the {@link FlowProgram.FlowResultLog#getAccepts() accepts} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAccepts(Map.Entry entry) {
this.accepts.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link FlowProgram.FlowResultLog#getAccepts() accepts} map. Nulls are not permitted
* @param entries The entries that will be added to the accepts map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder accepts(Map entries) {
this.accepts = ImmutableMap.builder();
return putAllAccepts(entries);
}
/**
* Put all mappings from the specified map as entries to {@link FlowProgram.FlowResultLog#getAccepts() accepts} map. Nulls are not permitted
* @param entries The entries that will be added to the accepts map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllAccepts(Map entries) {
this.accepts.putAll(entries);
return this;
}
/**
* Put one entry to the {@link FlowProgram.FlowResultLog#getReturns() returns} map.
* @param key The key in the returns map
* @param value The associated value in the returns map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putReturns(String key, Serializable value) {
this.returns.put(key, value);
return this;
}
/**
* Put one entry to the {@link FlowProgram.FlowResultLog#getReturns() returns} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putReturns(Map.Entry entry) {
this.returns.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link FlowProgram.FlowResultLog#getReturns() returns} map. Nulls are not permitted
* @param entries The entries that will be added to the returns map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder returns(Map entries) {
this.returns = ImmutableMap.builder();
return putAllReturns(entries);
}
/**
* Put all mappings from the specified map as entries to {@link FlowProgram.FlowResultLog#getReturns() returns} map. Nulls are not permitted
* @param entries The entries that will be added to the returns map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllReturns(Map entries) {
this.returns.putAll(entries);
return this;
}
/**
* Initializes the value for the {@link FlowProgram.FlowResultLog#getReturnsValue() returnsValue} attribute.
* @param returnsValue The value for returnsValue (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder returnsValue(@Nullable Serializable returnsValue) {
this.returnsValue = returnsValue;
return this;
}
/**
* Builds a new {@link ImmutableFlowResultLog ImmutableFlowResultLog}.
* @return An immutable instance of FlowResultLog
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableFlowResultLog build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableFlowResultLog(
id,
stepId,
start,
end,
errors.build(),
status,
accepts.build(),
returns.build(),
returnsValue);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_ID) != 0) attributes.add("id");
if ((initBits & INIT_BIT_STEP_ID) != 0) attributes.add("stepId");
if ((initBits & INIT_BIT_START) != 0) attributes.add("start");
if ((initBits & INIT_BIT_END) != 0) attributes.add("end");
if ((initBits & INIT_BIT_STATUS) != 0) attributes.add("status");
return "Cannot build FlowResultLog, some of required attributes are not set " + attributes;
}
}
}