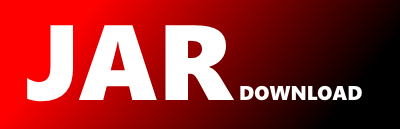
io.resys.hdes.client.api.programs.ImmutableProgramEnvir Maven / Gradle / Ivy
package io.resys.hdes.client.api.programs;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableMap;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import io.resys.hdes.client.api.ast.AstDecision;
import io.resys.hdes.client.api.ast.AstFlow;
import io.resys.hdes.client.api.ast.AstService;
import io.resys.hdes.client.api.ast.AstTag;
import java.util.Map;
import java.util.Objects;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link ProgramEnvir}.
*
* Use the builder to create immutable instances:
* {@code ImmutableProgramEnvir.builder()}.
*/
@Generated(from = "ProgramEnvir", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableProgramEnvir implements ProgramEnvir {
private final ImmutableMap> values;
private final ImmutableMap> tagsByName;
private final ImmutableMap> flowsByName;
private final ImmutableMap> decisionsByName;
private final ImmutableMap> servicesByName;
private ImmutableProgramEnvir(
ImmutableMap> values,
ImmutableMap> tagsByName,
ImmutableMap> flowsByName,
ImmutableMap> decisionsByName,
ImmutableMap> servicesByName) {
this.values = values;
this.tagsByName = tagsByName;
this.flowsByName = flowsByName;
this.decisionsByName = decisionsByName;
this.servicesByName = servicesByName;
}
/**
* @return The value of the {@code values} attribute
*/
@Override
public ImmutableMap> getValues() {
return values;
}
/**
* @return The value of the {@code tagsByName} attribute
*/
@Override
public ImmutableMap> getTagsByName() {
return tagsByName;
}
/**
* @return The value of the {@code flowsByName} attribute
*/
@Override
public ImmutableMap> getFlowsByName() {
return flowsByName;
}
/**
* @return The value of the {@code decisionsByName} attribute
*/
@Override
public ImmutableMap> getDecisionsByName() {
return decisionsByName;
}
/**
* @return The value of the {@code servicesByName} attribute
*/
@Override
public ImmutableMap> getServicesByName() {
return servicesByName;
}
/**
* Copy the current immutable object by replacing the {@link ProgramEnvir#getValues() values} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the values map
* @return A modified copy of {@code this} object
*/
public final ImmutableProgramEnvir withValues(Map> entries) {
if (this.values == entries) return this;
ImmutableMap> newValue = ImmutableMap.copyOf(entries);
return new ImmutableProgramEnvir(newValue, this.tagsByName, this.flowsByName, this.decisionsByName, this.servicesByName);
}
/**
* Copy the current immutable object by replacing the {@link ProgramEnvir#getTagsByName() tagsByName} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the tagsByName map
* @return A modified copy of {@code this} object
*/
public final ImmutableProgramEnvir withTagsByName(Map> entries) {
if (this.tagsByName == entries) return this;
ImmutableMap> newValue = ImmutableMap.copyOf(entries);
return new ImmutableProgramEnvir(this.values, newValue, this.flowsByName, this.decisionsByName, this.servicesByName);
}
/**
* Copy the current immutable object by replacing the {@link ProgramEnvir#getFlowsByName() flowsByName} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the flowsByName map
* @return A modified copy of {@code this} object
*/
public final ImmutableProgramEnvir withFlowsByName(Map> entries) {
if (this.flowsByName == entries) return this;
ImmutableMap> newValue = ImmutableMap.copyOf(entries);
return new ImmutableProgramEnvir(this.values, this.tagsByName, newValue, this.decisionsByName, this.servicesByName);
}
/**
* Copy the current immutable object by replacing the {@link ProgramEnvir#getDecisionsByName() decisionsByName} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the decisionsByName map
* @return A modified copy of {@code this} object
*/
public final ImmutableProgramEnvir withDecisionsByName(Map> entries) {
if (this.decisionsByName == entries) return this;
ImmutableMap> newValue = ImmutableMap.copyOf(entries);
return new ImmutableProgramEnvir(this.values, this.tagsByName, this.flowsByName, newValue, this.servicesByName);
}
/**
* Copy the current immutable object by replacing the {@link ProgramEnvir#getServicesByName() servicesByName} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the servicesByName map
* @return A modified copy of {@code this} object
*/
public final ImmutableProgramEnvir withServicesByName(Map> entries) {
if (this.servicesByName == entries) return this;
ImmutableMap> newValue = ImmutableMap.copyOf(entries);
return new ImmutableProgramEnvir(this.values, this.tagsByName, this.flowsByName, this.decisionsByName, newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableProgramEnvir} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableProgramEnvir
&& equalTo((ImmutableProgramEnvir) another);
}
private boolean equalTo(ImmutableProgramEnvir another) {
return values.equals(another.values)
&& tagsByName.equals(another.tagsByName)
&& flowsByName.equals(another.flowsByName)
&& decisionsByName.equals(another.decisionsByName)
&& servicesByName.equals(another.servicesByName);
}
/**
* Computes a hash code from attributes: {@code values}, {@code tagsByName}, {@code flowsByName}, {@code decisionsByName}, {@code servicesByName}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + values.hashCode();
h += (h << 5) + tagsByName.hashCode();
h += (h << 5) + flowsByName.hashCode();
h += (h << 5) + decisionsByName.hashCode();
h += (h << 5) + servicesByName.hashCode();
return h;
}
/**
* Prints the immutable value {@code ProgramEnvir} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("ProgramEnvir")
.omitNullValues()
.add("values", values)
.add("tagsByName", tagsByName)
.add("flowsByName", flowsByName)
.add("decisionsByName", decisionsByName)
.add("servicesByName", servicesByName)
.toString();
}
/**
* Creates an immutable copy of a {@link ProgramEnvir} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable ProgramEnvir instance
*/
public static ImmutableProgramEnvir copyOf(ProgramEnvir instance) {
if (instance instanceof ImmutableProgramEnvir) {
return (ImmutableProgramEnvir) instance;
}
return ImmutableProgramEnvir.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableProgramEnvir ImmutableProgramEnvir}.
*
* ImmutableProgramEnvir.builder()
* .putValues|putAllValues(String => io.resys.hdes.client.api.programs.ProgramEnvir.ProgramWrapper<?, ?>) // {@link ProgramEnvir#getValues() values} mappings
* .putTagsByName|putAllTagsByName(String => io.resys.hdes.client.api.programs.ProgramEnvir.ProgramWrapper<io.resys.hdes.client.api.ast.AstTag, io.resys.hdes.client.api.programs.TagProgram>) // {@link ProgramEnvir#getTagsByName() tagsByName} mappings
* .putFlowsByName|putAllFlowsByName(String => io.resys.hdes.client.api.programs.ProgramEnvir.ProgramWrapper<io.resys.hdes.client.api.ast.AstFlow, io.resys.hdes.client.api.programs.FlowProgram>) // {@link ProgramEnvir#getFlowsByName() flowsByName} mappings
* .putDecisionsByName|putAllDecisionsByName(String => io.resys.hdes.client.api.programs.ProgramEnvir.ProgramWrapper<io.resys.hdes.client.api.ast.AstDecision, io.resys.hdes.client.api.programs.DecisionProgram>) // {@link ProgramEnvir#getDecisionsByName() decisionsByName} mappings
* .putServicesByName|putAllServicesByName(String => io.resys.hdes.client.api.programs.ProgramEnvir.ProgramWrapper<io.resys.hdes.client.api.ast.AstService, io.resys.hdes.client.api.programs.ServiceProgram>) // {@link ProgramEnvir#getServicesByName() servicesByName} mappings
* .build();
*
* @return A new ImmutableProgramEnvir builder
*/
public static ImmutableProgramEnvir.Builder builder() {
return new ImmutableProgramEnvir.Builder();
}
/**
* Builds instances of type {@link ImmutableProgramEnvir ImmutableProgramEnvir}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "ProgramEnvir", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private ImmutableMap.Builder> values = ImmutableMap.builder();
private ImmutableMap.Builder> tagsByName = ImmutableMap.builder();
private ImmutableMap.Builder> flowsByName = ImmutableMap.builder();
private ImmutableMap.Builder> decisionsByName = ImmutableMap.builder();
private ImmutableMap.Builder> servicesByName = ImmutableMap.builder();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code ProgramEnvir} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(ProgramEnvir instance) {
Objects.requireNonNull(instance, "instance");
putAllValues(instance.getValues());
putAllTagsByName(instance.getTagsByName());
putAllFlowsByName(instance.getFlowsByName());
putAllDecisionsByName(instance.getDecisionsByName());
putAllServicesByName(instance.getServicesByName());
return this;
}
/**
* Put one entry to the {@link ProgramEnvir#getValues() values} map.
* @param key The key in the values map
* @param value The associated value in the values map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putValues(String key, ProgramEnvir.ProgramWrapper, ?> value) {
this.values.put(key, value);
return this;
}
/**
* Put one entry to the {@link ProgramEnvir#getValues() values} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putValues(Map.Entry> entry) {
this.values.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link ProgramEnvir#getValues() values} map. Nulls are not permitted
* @param entries The entries that will be added to the values map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder values(Map> entries) {
this.values = ImmutableMap.builder();
return putAllValues(entries);
}
/**
* Put all mappings from the specified map as entries to {@link ProgramEnvir#getValues() values} map. Nulls are not permitted
* @param entries The entries that will be added to the values map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllValues(Map> entries) {
this.values.putAll(entries);
return this;
}
/**
* Put one entry to the {@link ProgramEnvir#getTagsByName() tagsByName} map.
* @param key The key in the tagsByName map
* @param value The associated value in the tagsByName map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putTagsByName(String key, ProgramEnvir.ProgramWrapper value) {
this.tagsByName.put(key, value);
return this;
}
/**
* Put one entry to the {@link ProgramEnvir#getTagsByName() tagsByName} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putTagsByName(Map.Entry> entry) {
this.tagsByName.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link ProgramEnvir#getTagsByName() tagsByName} map. Nulls are not permitted
* @param entries The entries that will be added to the tagsByName map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder tagsByName(Map> entries) {
this.tagsByName = ImmutableMap.builder();
return putAllTagsByName(entries);
}
/**
* Put all mappings from the specified map as entries to {@link ProgramEnvir#getTagsByName() tagsByName} map. Nulls are not permitted
* @param entries The entries that will be added to the tagsByName map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllTagsByName(Map> entries) {
this.tagsByName.putAll(entries);
return this;
}
/**
* Put one entry to the {@link ProgramEnvir#getFlowsByName() flowsByName} map.
* @param key The key in the flowsByName map
* @param value The associated value in the flowsByName map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putFlowsByName(String key, ProgramEnvir.ProgramWrapper value) {
this.flowsByName.put(key, value);
return this;
}
/**
* Put one entry to the {@link ProgramEnvir#getFlowsByName() flowsByName} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putFlowsByName(Map.Entry> entry) {
this.flowsByName.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link ProgramEnvir#getFlowsByName() flowsByName} map. Nulls are not permitted
* @param entries The entries that will be added to the flowsByName map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder flowsByName(Map> entries) {
this.flowsByName = ImmutableMap.builder();
return putAllFlowsByName(entries);
}
/**
* Put all mappings from the specified map as entries to {@link ProgramEnvir#getFlowsByName() flowsByName} map. Nulls are not permitted
* @param entries The entries that will be added to the flowsByName map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllFlowsByName(Map> entries) {
this.flowsByName.putAll(entries);
return this;
}
/**
* Put one entry to the {@link ProgramEnvir#getDecisionsByName() decisionsByName} map.
* @param key The key in the decisionsByName map
* @param value The associated value in the decisionsByName map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putDecisionsByName(String key, ProgramEnvir.ProgramWrapper value) {
this.decisionsByName.put(key, value);
return this;
}
/**
* Put one entry to the {@link ProgramEnvir#getDecisionsByName() decisionsByName} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putDecisionsByName(Map.Entry> entry) {
this.decisionsByName.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link ProgramEnvir#getDecisionsByName() decisionsByName} map. Nulls are not permitted
* @param entries The entries that will be added to the decisionsByName map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder decisionsByName(Map> entries) {
this.decisionsByName = ImmutableMap.builder();
return putAllDecisionsByName(entries);
}
/**
* Put all mappings from the specified map as entries to {@link ProgramEnvir#getDecisionsByName() decisionsByName} map. Nulls are not permitted
* @param entries The entries that will be added to the decisionsByName map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllDecisionsByName(Map> entries) {
this.decisionsByName.putAll(entries);
return this;
}
/**
* Put one entry to the {@link ProgramEnvir#getServicesByName() servicesByName} map.
* @param key The key in the servicesByName map
* @param value The associated value in the servicesByName map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putServicesByName(String key, ProgramEnvir.ProgramWrapper value) {
this.servicesByName.put(key, value);
return this;
}
/**
* Put one entry to the {@link ProgramEnvir#getServicesByName() servicesByName} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putServicesByName(Map.Entry> entry) {
this.servicesByName.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link ProgramEnvir#getServicesByName() servicesByName} map. Nulls are not permitted
* @param entries The entries that will be added to the servicesByName map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder servicesByName(Map> entries) {
this.servicesByName = ImmutableMap.builder();
return putAllServicesByName(entries);
}
/**
* Put all mappings from the specified map as entries to {@link ProgramEnvir#getServicesByName() servicesByName} map. Nulls are not permitted
* @param entries The entries that will be added to the servicesByName map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllServicesByName(Map> entries) {
this.servicesByName.putAll(entries);
return this;
}
/**
* Builds a new {@link ImmutableProgramEnvir ImmutableProgramEnvir}.
* @return An immutable instance of ProgramEnvir
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableProgramEnvir build() {
return new ImmutableProgramEnvir(
values.build(),
tagsByName.build(),
flowsByName.build(),
decisionsByName.build(),
servicesByName.build());
}
}
}