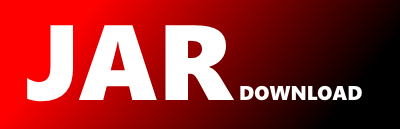
io.resys.hdes.client.api.programs.ImmutableProgramWrapper Maven / Gradle / Ivy
package io.resys.hdes.client.api.programs;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableList;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import io.resys.hdes.client.api.ast.AstBody;
import io.resys.hdes.client.api.ast.TypeDef;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link ProgramEnvir.ProgramWrapper}.
*
* Use the builder to create immutable instances:
* {@code ImmutableProgramWrapper.builder()}.
*/
@Generated(from = "ProgramEnvir.ProgramWrapper", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableProgramWrapper
implements ProgramEnvir.ProgramWrapper {
private final String id;
private final AstBody.AstBodyType type;
private final ProgramEnvir.ProgramStatus status;
private final ImmutableList warnings;
private final ImmutableList errors;
private final ImmutableList headers;
private final ImmutableList associations;
private final AstBody.AstSource source;
private final @Nullable A ast;
private final @Nullable P program;
private ImmutableProgramWrapper(
String id,
AstBody.AstBodyType type,
ProgramEnvir.ProgramStatus status,
ImmutableList warnings,
ImmutableList errors,
ImmutableList headers,
ImmutableList associations,
AstBody.AstSource source,
@Nullable A ast,
@Nullable P program) {
this.id = id;
this.type = type;
this.status = status;
this.warnings = warnings;
this.errors = errors;
this.headers = headers;
this.associations = associations;
this.source = source;
this.ast = ast;
this.program = program;
}
/**
* @return The value of the {@code id} attribute
*/
@Override
public String getId() {
return id;
}
/**
* @return The value of the {@code type} attribute
*/
@Override
public AstBody.AstBodyType getType() {
return type;
}
/**
* @return The value of the {@code status} attribute
*/
@Override
public ProgramEnvir.ProgramStatus getStatus() {
return status;
}
/**
* @return The value of the {@code warnings} attribute
*/
@Override
public ImmutableList getWarnings() {
return warnings;
}
/**
* @return The value of the {@code errors} attribute
*/
@Override
public ImmutableList getErrors() {
return errors;
}
/**
* @return The value of the {@code headers} attribute
*/
@Override
public ImmutableList getHeaders() {
return headers;
}
/**
* @return The value of the {@code associations} attribute
*/
@Override
public ImmutableList getAssociations() {
return associations;
}
/**
* @return The value of the {@code source} attribute
*/
@Override
public AstBody.AstSource getSource() {
return source;
}
/**
* @return The value of the {@code ast} attribute
*/
@Override
public Optional getAst() {
return Optional.ofNullable(ast);
}
/**
* @return The value of the {@code program} attribute
*/
@Override
public Optional getProgram() {
return Optional.ofNullable(program);
}
/**
* Copy the current immutable object by setting a value for the {@link ProgramEnvir.ProgramWrapper#getId() id} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for id
* @return A modified copy of the {@code this} object
*/
public final ImmutableProgramWrapper withId(String value) {
String newValue = Objects.requireNonNull(value, "id");
if (this.id.equals(newValue)) return this;
return new ImmutableProgramWrapper<>(
newValue,
this.type,
this.status,
this.warnings,
this.errors,
this.headers,
this.associations,
this.source,
this.ast,
this.program);
}
/**
* Copy the current immutable object by setting a value for the {@link ProgramEnvir.ProgramWrapper#getType() type} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for type
* @return A modified copy of the {@code this} object
*/
public final ImmutableProgramWrapper withType(AstBody.AstBodyType value) {
if (this.type == value) return this;
AstBody.AstBodyType newValue = Objects.requireNonNull(value, "type");
if (this.type.equals(newValue)) return this;
return new ImmutableProgramWrapper<>(
this.id,
newValue,
this.status,
this.warnings,
this.errors,
this.headers,
this.associations,
this.source,
this.ast,
this.program);
}
/**
* Copy the current immutable object by setting a value for the {@link ProgramEnvir.ProgramWrapper#getStatus() status} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for status
* @return A modified copy of the {@code this} object
*/
public final ImmutableProgramWrapper withStatus(ProgramEnvir.ProgramStatus value) {
if (this.status == value) return this;
ProgramEnvir.ProgramStatus newValue = Objects.requireNonNull(value, "status");
if (this.status.equals(newValue)) return this;
return new ImmutableProgramWrapper<>(
this.id,
this.type,
newValue,
this.warnings,
this.errors,
this.headers,
this.associations,
this.source,
this.ast,
this.program);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ProgramEnvir.ProgramWrapper#getWarnings() warnings}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableProgramWrapper withWarnings(ProgramEnvir.ProgramMessage... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableProgramWrapper<>(
this.id,
this.type,
this.status,
newValue,
this.errors,
this.headers,
this.associations,
this.source,
this.ast,
this.program);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ProgramEnvir.ProgramWrapper#getWarnings() warnings}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of warnings elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableProgramWrapper withWarnings(Iterable extends ProgramEnvir.ProgramMessage> elements) {
if (this.warnings == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableProgramWrapper<>(
this.id,
this.type,
this.status,
newValue,
this.errors,
this.headers,
this.associations,
this.source,
this.ast,
this.program);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ProgramEnvir.ProgramWrapper#getErrors() errors}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableProgramWrapper withErrors(ProgramEnvir.ProgramMessage... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableProgramWrapper<>(
this.id,
this.type,
this.status,
this.warnings,
newValue,
this.headers,
this.associations,
this.source,
this.ast,
this.program);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ProgramEnvir.ProgramWrapper#getErrors() errors}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of errors elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableProgramWrapper withErrors(Iterable extends ProgramEnvir.ProgramMessage> elements) {
if (this.errors == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableProgramWrapper<>(
this.id,
this.type,
this.status,
this.warnings,
newValue,
this.headers,
this.associations,
this.source,
this.ast,
this.program);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ProgramEnvir.ProgramWrapper#getHeaders() headers}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableProgramWrapper withHeaders(TypeDef... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableProgramWrapper<>(
this.id,
this.type,
this.status,
this.warnings,
this.errors,
newValue,
this.associations,
this.source,
this.ast,
this.program);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ProgramEnvir.ProgramWrapper#getHeaders() headers}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of headers elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableProgramWrapper withHeaders(Iterable extends TypeDef> elements) {
if (this.headers == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableProgramWrapper<>(
this.id,
this.type,
this.status,
this.warnings,
this.errors,
newValue,
this.associations,
this.source,
this.ast,
this.program);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ProgramEnvir.ProgramWrapper#getAssociations() associations}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableProgramWrapper withAssociations(ProgramEnvir.ProgramAssociation... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableProgramWrapper<>(
this.id,
this.type,
this.status,
this.warnings,
this.errors,
this.headers,
newValue,
this.source,
this.ast,
this.program);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ProgramEnvir.ProgramWrapper#getAssociations() associations}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of associations elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableProgramWrapper withAssociations(Iterable extends ProgramEnvir.ProgramAssociation> elements) {
if (this.associations == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableProgramWrapper<>(
this.id,
this.type,
this.status,
this.warnings,
this.errors,
this.headers,
newValue,
this.source,
this.ast,
this.program);
}
/**
* Copy the current immutable object by setting a value for the {@link ProgramEnvir.ProgramWrapper#getSource() source} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for source
* @return A modified copy of the {@code this} object
*/
public final ImmutableProgramWrapper withSource(AstBody.AstSource value) {
if (this.source == value) return this;
AstBody.AstSource newValue = Objects.requireNonNull(value, "source");
return new ImmutableProgramWrapper<>(
this.id,
this.type,
this.status,
this.warnings,
this.errors,
this.headers,
this.associations,
newValue,
this.ast,
this.program);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link ProgramEnvir.ProgramWrapper#getAst() ast} attribute.
* @param value The value for ast
* @return A modified copy of {@code this} object
*/
public final ImmutableProgramWrapper withAst(A value) {
@Nullable A newValue = Objects.requireNonNull(value, "ast");
if (this.ast == newValue) return this;
return new ImmutableProgramWrapper<>(
this.id,
this.type,
this.status,
this.warnings,
this.errors,
this.headers,
this.associations,
this.source,
newValue,
this.program);
}
/**
* Copy the current immutable object by setting an optional value for the {@link ProgramEnvir.ProgramWrapper#getAst() ast} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for ast
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final ImmutableProgramWrapper withAst(Optional extends A> optional) {
@Nullable A value = optional.orElse(null);
if (this.ast == value) return this;
return new ImmutableProgramWrapper<>(
this.id,
this.type,
this.status,
this.warnings,
this.errors,
this.headers,
this.associations,
this.source,
value,
this.program);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link ProgramEnvir.ProgramWrapper#getProgram() program} attribute.
* @param value The value for program
* @return A modified copy of {@code this} object
*/
public final ImmutableProgramWrapper withProgram(P value) {
@Nullable P newValue = Objects.requireNonNull(value, "program");
if (this.program == newValue) return this;
return new ImmutableProgramWrapper<>(
this.id,
this.type,
this.status,
this.warnings,
this.errors,
this.headers,
this.associations,
this.source,
this.ast,
newValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link ProgramEnvir.ProgramWrapper#getProgram() program} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for program
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final ImmutableProgramWrapper withProgram(Optional extends P> optional) {
@Nullable P value = optional.orElse(null);
if (this.program == value) return this;
return new ImmutableProgramWrapper<>(
this.id,
this.type,
this.status,
this.warnings,
this.errors,
this.headers,
this.associations,
this.source,
this.ast,
value);
}
/**
* This instance is equal to all instances of {@code ImmutableProgramWrapper} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableProgramWrapper, ?>
&& equalTo((ImmutableProgramWrapper, ?>) another);
}
private boolean equalTo(ImmutableProgramWrapper, ?> another) {
return id.equals(another.id)
&& type.equals(another.type)
&& status.equals(another.status)
&& warnings.equals(another.warnings)
&& errors.equals(another.errors)
&& headers.equals(another.headers)
&& associations.equals(another.associations)
&& source.equals(another.source)
&& Objects.equals(ast, another.ast)
&& Objects.equals(program, another.program);
}
/**
* Computes a hash code from attributes: {@code id}, {@code type}, {@code status}, {@code warnings}, {@code errors}, {@code headers}, {@code associations}, {@code source}, {@code ast}, {@code program}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + id.hashCode();
h += (h << 5) + type.hashCode();
h += (h << 5) + status.hashCode();
h += (h << 5) + warnings.hashCode();
h += (h << 5) + errors.hashCode();
h += (h << 5) + headers.hashCode();
h += (h << 5) + associations.hashCode();
h += (h << 5) + source.hashCode();
h += (h << 5) + Objects.hashCode(ast);
h += (h << 5) + Objects.hashCode(program);
return h;
}
/**
* Prints the immutable value {@code ProgramWrapper} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("ProgramWrapper")
.omitNullValues()
.add("id", id)
.add("type", type)
.add("status", status)
.add("warnings", warnings)
.add("errors", errors)
.add("headers", headers)
.add("associations", associations)
.add("source", source)
.add("ast", ast)
.add("program", program)
.toString();
}
/**
* Creates an immutable copy of a {@link ProgramEnvir.ProgramWrapper} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param generic parameter A
* @param generic parameter P
* @param instance The instance to copy
* @return A copied immutable ProgramWrapper instance
*/
public static ImmutableProgramWrapper copyOf(ProgramEnvir.ProgramWrapper instance) {
if (instance instanceof ImmutableProgramWrapper, ?>) {
return (ImmutableProgramWrapper) instance;
}
return ImmutableProgramWrapper.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableProgramWrapper ImmutableProgramWrapper}.
*
* ImmutableProgramWrapper.<A, P>builder()
* .id(String) // required {@link ProgramEnvir.ProgramWrapper#getId() id}
* .type(io.resys.hdes.client.api.ast.AstBody.AstBodyType) // required {@link ProgramEnvir.ProgramWrapper#getType() type}
* .status(io.resys.hdes.client.api.programs.ProgramEnvir.ProgramStatus) // required {@link ProgramEnvir.ProgramWrapper#getStatus() status}
* .addWarnings|addAllWarnings(io.resys.hdes.client.api.programs.ProgramEnvir.ProgramMessage) // {@link ProgramEnvir.ProgramWrapper#getWarnings() warnings} elements
* .addErrors|addAllErrors(io.resys.hdes.client.api.programs.ProgramEnvir.ProgramMessage) // {@link ProgramEnvir.ProgramWrapper#getErrors() errors} elements
* .addHeaders|addAllHeaders(io.resys.hdes.client.api.ast.TypeDef) // {@link ProgramEnvir.ProgramWrapper#getHeaders() headers} elements
* .addAssociations|addAllAssociations(io.resys.hdes.client.api.programs.ProgramEnvir.ProgramAssociation) // {@link ProgramEnvir.ProgramWrapper#getAssociations() associations} elements
* .source(io.resys.hdes.client.api.ast.AstBody.AstSource) // required {@link ProgramEnvir.ProgramWrapper#getSource() source}
* .ast(A) // optional {@link ProgramEnvir.ProgramWrapper#getAst() ast}
* .program(P) // optional {@link ProgramEnvir.ProgramWrapper#getProgram() program}
* .build();
*
* @param generic parameter A
* @param generic parameter P
* @return A new ImmutableProgramWrapper builder
*/
public static ImmutableProgramWrapper.Builder builder() {
return new ImmutableProgramWrapper.Builder<>();
}
/**
* Builds instances of type {@link ImmutableProgramWrapper ImmutableProgramWrapper}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "ProgramEnvir.ProgramWrapper", generator = "Immutables")
@NotThreadSafe
public static final class Builder
{
private static final long INIT_BIT_ID = 0x1L;
private static final long INIT_BIT_TYPE = 0x2L;
private static final long INIT_BIT_STATUS = 0x4L;
private static final long INIT_BIT_SOURCE = 0x8L;
private long initBits = 0xfL;
private @Nullable String id;
private @Nullable AstBody.AstBodyType type;
private @Nullable ProgramEnvir.ProgramStatus status;
private ImmutableList.Builder warnings = ImmutableList.builder();
private ImmutableList.Builder errors = ImmutableList.builder();
private ImmutableList.Builder headers = ImmutableList.builder();
private ImmutableList.Builder associations = ImmutableList.builder();
private @Nullable AstBody.AstSource source;
private @Nullable A ast;
private @Nullable P program;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code ProgramWrapper} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(ProgramEnvir.ProgramWrapper instance) {
Objects.requireNonNull(instance, "instance");
id(instance.getId());
type(instance.getType());
status(instance.getStatus());
addAllWarnings(instance.getWarnings());
addAllErrors(instance.getErrors());
addAllHeaders(instance.getHeaders());
addAllAssociations(instance.getAssociations());
source(instance.getSource());
Optional astOptional = instance.getAst();
if (astOptional.isPresent()) {
ast(astOptional);
}
Optional programOptional = instance.getProgram();
if (programOptional.isPresent()) {
program(programOptional);
}
return this;
}
/**
* Initializes the value for the {@link ProgramEnvir.ProgramWrapper#getId() id} attribute.
* @param id The value for id
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder id(String id) {
this.id = Objects.requireNonNull(id, "id");
initBits &= ~INIT_BIT_ID;
return this;
}
/**
* Initializes the value for the {@link ProgramEnvir.ProgramWrapper#getType() type} attribute.
* @param type The value for type
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder type(AstBody.AstBodyType type) {
this.type = Objects.requireNonNull(type, "type");
initBits &= ~INIT_BIT_TYPE;
return this;
}
/**
* Initializes the value for the {@link ProgramEnvir.ProgramWrapper#getStatus() status} attribute.
* @param status The value for status
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder status(ProgramEnvir.ProgramStatus status) {
this.status = Objects.requireNonNull(status, "status");
initBits &= ~INIT_BIT_STATUS;
return this;
}
/**
* Adds one element to {@link ProgramEnvir.ProgramWrapper#getWarnings() warnings} list.
* @param element A warnings element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addWarnings(ProgramEnvir.ProgramMessage element) {
this.warnings.add(element);
return this;
}
/**
* Adds elements to {@link ProgramEnvir.ProgramWrapper#getWarnings() warnings} list.
* @param elements An array of warnings elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addWarnings(ProgramEnvir.ProgramMessage... elements) {
this.warnings.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link ProgramEnvir.ProgramWrapper#getWarnings() warnings} list.
* @param elements An iterable of warnings elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder warnings(Iterable extends ProgramEnvir.ProgramMessage> elements) {
this.warnings = ImmutableList.builder();
return addAllWarnings(elements);
}
/**
* Adds elements to {@link ProgramEnvir.ProgramWrapper#getWarnings() warnings} list.
* @param elements An iterable of warnings elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllWarnings(Iterable extends ProgramEnvir.ProgramMessage> elements) {
this.warnings.addAll(elements);
return this;
}
/**
* Adds one element to {@link ProgramEnvir.ProgramWrapper#getErrors() errors} list.
* @param element A errors element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addErrors(ProgramEnvir.ProgramMessage element) {
this.errors.add(element);
return this;
}
/**
* Adds elements to {@link ProgramEnvir.ProgramWrapper#getErrors() errors} list.
* @param elements An array of errors elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addErrors(ProgramEnvir.ProgramMessage... elements) {
this.errors.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link ProgramEnvir.ProgramWrapper#getErrors() errors} list.
* @param elements An iterable of errors elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder errors(Iterable extends ProgramEnvir.ProgramMessage> elements) {
this.errors = ImmutableList.builder();
return addAllErrors(elements);
}
/**
* Adds elements to {@link ProgramEnvir.ProgramWrapper#getErrors() errors} list.
* @param elements An iterable of errors elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllErrors(Iterable extends ProgramEnvir.ProgramMessage> elements) {
this.errors.addAll(elements);
return this;
}
/**
* Adds one element to {@link ProgramEnvir.ProgramWrapper#getHeaders() headers} list.
* @param element A headers element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addHeaders(TypeDef element) {
this.headers.add(element);
return this;
}
/**
* Adds elements to {@link ProgramEnvir.ProgramWrapper#getHeaders() headers} list.
* @param elements An array of headers elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addHeaders(TypeDef... elements) {
this.headers.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link ProgramEnvir.ProgramWrapper#getHeaders() headers} list.
* @param elements An iterable of headers elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder headers(Iterable extends TypeDef> elements) {
this.headers = ImmutableList.builder();
return addAllHeaders(elements);
}
/**
* Adds elements to {@link ProgramEnvir.ProgramWrapper#getHeaders() headers} list.
* @param elements An iterable of headers elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllHeaders(Iterable extends TypeDef> elements) {
this.headers.addAll(elements);
return this;
}
/**
* Adds one element to {@link ProgramEnvir.ProgramWrapper#getAssociations() associations} list.
* @param element A associations element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAssociations(ProgramEnvir.ProgramAssociation element) {
this.associations.add(element);
return this;
}
/**
* Adds elements to {@link ProgramEnvir.ProgramWrapper#getAssociations() associations} list.
* @param elements An array of associations elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAssociations(ProgramEnvir.ProgramAssociation... elements) {
this.associations.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link ProgramEnvir.ProgramWrapper#getAssociations() associations} list.
* @param elements An iterable of associations elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder associations(Iterable extends ProgramEnvir.ProgramAssociation> elements) {
this.associations = ImmutableList.builder();
return addAllAssociations(elements);
}
/**
* Adds elements to {@link ProgramEnvir.ProgramWrapper#getAssociations() associations} list.
* @param elements An iterable of associations elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllAssociations(Iterable extends ProgramEnvir.ProgramAssociation> elements) {
this.associations.addAll(elements);
return this;
}
/**
* Initializes the value for the {@link ProgramEnvir.ProgramWrapper#getSource() source} attribute.
* @param source The value for source
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder source(AstBody.AstSource source) {
this.source = Objects.requireNonNull(source, "source");
initBits &= ~INIT_BIT_SOURCE;
return this;
}
/**
* Initializes the optional value {@link ProgramEnvir.ProgramWrapper#getAst() ast} to ast.
* @param ast The value for ast
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder ast(A ast) {
this.ast = Objects.requireNonNull(ast, "ast");
return this;
}
/**
* Initializes the optional value {@link ProgramEnvir.ProgramWrapper#getAst() ast} to ast.
* @param ast The value for ast
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder ast(Optional extends A> ast) {
this.ast = ast.orElse(null);
return this;
}
/**
* Initializes the optional value {@link ProgramEnvir.ProgramWrapper#getProgram() program} to program.
* @param program The value for program
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder program(P program) {
this.program = Objects.requireNonNull(program, "program");
return this;
}
/**
* Initializes the optional value {@link ProgramEnvir.ProgramWrapper#getProgram() program} to program.
* @param program The value for program
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder program(Optional extends P> program) {
this.program = program.orElse(null);
return this;
}
/**
* Builds a new {@link ImmutableProgramWrapper ImmutableProgramWrapper}.
* @return An immutable instance of ProgramWrapper
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableProgramWrapper build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableProgramWrapper<>(
id,
type,
status,
warnings.build(),
errors.build(),
headers.build(),
associations.build(),
source,
ast,
program);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_ID) != 0) attributes.add("id");
if ((initBits & INIT_BIT_TYPE) != 0) attributes.add("type");
if ((initBits & INIT_BIT_STATUS) != 0) attributes.add("status");
if ((initBits & INIT_BIT_SOURCE) != 0) attributes.add("source");
return "Cannot build ProgramWrapper, some of required attributes are not set " + attributes;
}
}
}