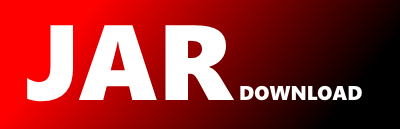
io.resys.hdes.client.api.ImmutableComposerState Maven / Gradle / Ivy
package io.resys.hdes.client.api;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableMap;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import io.resys.hdes.client.api.ast.AstBranch;
import io.resys.hdes.client.api.ast.AstDecision;
import io.resys.hdes.client.api.ast.AstFlow;
import io.resys.hdes.client.api.ast.AstService;
import io.resys.hdes.client.api.ast.AstTag;
import java.util.Map;
import java.util.Objects;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link HdesComposer.ComposerState}.
*
* Use the builder to create immutable instances:
* {@code ImmutableComposerState.builder()}.
*/
@Generated(from = "HdesComposer.ComposerState", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableComposerState implements HdesComposer.ComposerState {
private final ImmutableMap> tags;
private final ImmutableMap> flows;
private final ImmutableMap> services;
private final ImmutableMap> decisions;
private final ImmutableMap> branches;
private ImmutableComposerState(
ImmutableMap> tags,
ImmutableMap> flows,
ImmutableMap> services,
ImmutableMap> decisions,
ImmutableMap> branches) {
this.tags = tags;
this.flows = flows;
this.services = services;
this.decisions = decisions;
this.branches = branches;
}
/**
* @return The value of the {@code tags} attribute
*/
@JsonProperty("tags")
@Override
public ImmutableMap> getTags() {
return tags;
}
/**
* @return The value of the {@code flows} attribute
*/
@JsonProperty("flows")
@Override
public ImmutableMap> getFlows() {
return flows;
}
/**
* @return The value of the {@code services} attribute
*/
@JsonProperty("services")
@Override
public ImmutableMap> getServices() {
return services;
}
/**
* @return The value of the {@code decisions} attribute
*/
@JsonProperty("decisions")
@Override
public ImmutableMap> getDecisions() {
return decisions;
}
/**
* @return The value of the {@code branches} attribute
*/
@JsonProperty("branches")
@Override
public ImmutableMap> getBranches() {
return branches;
}
/**
* Copy the current immutable object by replacing the {@link HdesComposer.ComposerState#getTags() tags} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the tags map
* @return A modified copy of {@code this} object
*/
public final ImmutableComposerState withTags(Map> entries) {
if (this.tags == entries) return this;
ImmutableMap> newValue = ImmutableMap.copyOf(entries);
return new ImmutableComposerState(newValue, this.flows, this.services, this.decisions, this.branches);
}
/**
* Copy the current immutable object by replacing the {@link HdesComposer.ComposerState#getFlows() flows} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the flows map
* @return A modified copy of {@code this} object
*/
public final ImmutableComposerState withFlows(Map> entries) {
if (this.flows == entries) return this;
ImmutableMap> newValue = ImmutableMap.copyOf(entries);
return new ImmutableComposerState(this.tags, newValue, this.services, this.decisions, this.branches);
}
/**
* Copy the current immutable object by replacing the {@link HdesComposer.ComposerState#getServices() services} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the services map
* @return A modified copy of {@code this} object
*/
public final ImmutableComposerState withServices(Map> entries) {
if (this.services == entries) return this;
ImmutableMap> newValue = ImmutableMap.copyOf(entries);
return new ImmutableComposerState(this.tags, this.flows, newValue, this.decisions, this.branches);
}
/**
* Copy the current immutable object by replacing the {@link HdesComposer.ComposerState#getDecisions() decisions} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the decisions map
* @return A modified copy of {@code this} object
*/
public final ImmutableComposerState withDecisions(Map> entries) {
if (this.decisions == entries) return this;
ImmutableMap> newValue = ImmutableMap.copyOf(entries);
return new ImmutableComposerState(this.tags, this.flows, this.services, newValue, this.branches);
}
/**
* Copy the current immutable object by replacing the {@link HdesComposer.ComposerState#getBranches() branches} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the branches map
* @return A modified copy of {@code this} object
*/
public final ImmutableComposerState withBranches(Map> entries) {
if (this.branches == entries) return this;
ImmutableMap> newValue = ImmutableMap.copyOf(entries);
return new ImmutableComposerState(this.tags, this.flows, this.services, this.decisions, newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableComposerState} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableComposerState
&& equalTo((ImmutableComposerState) another);
}
private boolean equalTo(ImmutableComposerState another) {
return tags.equals(another.tags)
&& flows.equals(another.flows)
&& services.equals(another.services)
&& decisions.equals(another.decisions)
&& branches.equals(another.branches);
}
/**
* Computes a hash code from attributes: {@code tags}, {@code flows}, {@code services}, {@code decisions}, {@code branches}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + tags.hashCode();
h += (h << 5) + flows.hashCode();
h += (h << 5) + services.hashCode();
h += (h << 5) + decisions.hashCode();
h += (h << 5) + branches.hashCode();
return h;
}
/**
* Prints the immutable value {@code ComposerState} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("ComposerState")
.omitNullValues()
.add("tags", tags)
.add("flows", flows)
.add("services", services)
.add("decisions", decisions)
.add("branches", branches)
.toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "HdesComposer.ComposerState", generator = "Immutables")
@Deprecated
@SuppressWarnings("Immutable")
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements HdesComposer.ComposerState {
@Nullable Map> tags = ImmutableMap.of();
@Nullable Map> flows = ImmutableMap.of();
@Nullable Map> services = ImmutableMap.of();
@Nullable Map> decisions = ImmutableMap.of();
@Nullable Map> branches = ImmutableMap.of();
@JsonProperty("tags")
public void setTags(Map> tags) {
this.tags = tags;
}
@JsonProperty("flows")
public void setFlows(Map> flows) {
this.flows = flows;
}
@JsonProperty("services")
public void setServices(Map> services) {
this.services = services;
}
@JsonProperty("decisions")
public void setDecisions(Map> decisions) {
this.decisions = decisions;
}
@JsonProperty("branches")
public void setBranches(Map> branches) {
this.branches = branches;
}
@Override
public Map> getTags() { throw new UnsupportedOperationException(); }
@Override
public Map> getFlows() { throw new UnsupportedOperationException(); }
@Override
public Map> getServices() { throw new UnsupportedOperationException(); }
@Override
public Map> getDecisions() { throw new UnsupportedOperationException(); }
@Override
public Map> getBranches() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableComposerState fromJson(Json json) {
ImmutableComposerState.Builder builder = ImmutableComposerState.builder();
if (json.tags != null) {
builder.putAllTags(json.tags);
}
if (json.flows != null) {
builder.putAllFlows(json.flows);
}
if (json.services != null) {
builder.putAllServices(json.services);
}
if (json.decisions != null) {
builder.putAllDecisions(json.decisions);
}
if (json.branches != null) {
builder.putAllBranches(json.branches);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link HdesComposer.ComposerState} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable ComposerState instance
*/
public static ImmutableComposerState copyOf(HdesComposer.ComposerState instance) {
if (instance instanceof ImmutableComposerState) {
return (ImmutableComposerState) instance;
}
return ImmutableComposerState.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableComposerState ImmutableComposerState}.
*
* ImmutableComposerState.builder()
* .putTags|putAllTags(String => io.resys.hdes.client.api.HdesComposer.ComposerEntity<io.resys.hdes.client.api.ast.AstTag>) // {@link HdesComposer.ComposerState#getTags() tags} mappings
* .putFlows|putAllFlows(String => io.resys.hdes.client.api.HdesComposer.ComposerEntity<io.resys.hdes.client.api.ast.AstFlow>) // {@link HdesComposer.ComposerState#getFlows() flows} mappings
* .putServices|putAllServices(String => io.resys.hdes.client.api.HdesComposer.ComposerEntity<io.resys.hdes.client.api.ast.AstService>) // {@link HdesComposer.ComposerState#getServices() services} mappings
* .putDecisions|putAllDecisions(String => io.resys.hdes.client.api.HdesComposer.ComposerEntity<io.resys.hdes.client.api.ast.AstDecision>) // {@link HdesComposer.ComposerState#getDecisions() decisions} mappings
* .putBranches|putAllBranches(String => io.resys.hdes.client.api.HdesComposer.ComposerEntity<io.resys.hdes.client.api.ast.AstBranch>) // {@link HdesComposer.ComposerState#getBranches() branches} mappings
* .build();
*
* @return A new ImmutableComposerState builder
*/
public static ImmutableComposerState.Builder builder() {
return new ImmutableComposerState.Builder();
}
/**
* Builds instances of type {@link ImmutableComposerState ImmutableComposerState}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "HdesComposer.ComposerState", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private ImmutableMap.Builder> tags = ImmutableMap.builder();
private ImmutableMap.Builder> flows = ImmutableMap.builder();
private ImmutableMap.Builder> services = ImmutableMap.builder();
private ImmutableMap.Builder> decisions = ImmutableMap.builder();
private ImmutableMap.Builder> branches = ImmutableMap.builder();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code ComposerState} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(HdesComposer.ComposerState instance) {
Objects.requireNonNull(instance, "instance");
putAllTags(instance.getTags());
putAllFlows(instance.getFlows());
putAllServices(instance.getServices());
putAllDecisions(instance.getDecisions());
putAllBranches(instance.getBranches());
return this;
}
/**
* Put one entry to the {@link HdesComposer.ComposerState#getTags() tags} map.
* @param key The key in the tags map
* @param value The associated value in the tags map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putTags(String key, HdesComposer.ComposerEntity value) {
this.tags.put(key, value);
return this;
}
/**
* Put one entry to the {@link HdesComposer.ComposerState#getTags() tags} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putTags(Map.Entry> entry) {
this.tags.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link HdesComposer.ComposerState#getTags() tags} map. Nulls are not permitted
* @param entries The entries that will be added to the tags map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("tags")
public final Builder tags(Map> entries) {
this.tags = ImmutableMap.builder();
return putAllTags(entries);
}
/**
* Put all mappings from the specified map as entries to {@link HdesComposer.ComposerState#getTags() tags} map. Nulls are not permitted
* @param entries The entries that will be added to the tags map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllTags(Map> entries) {
this.tags.putAll(entries);
return this;
}
/**
* Put one entry to the {@link HdesComposer.ComposerState#getFlows() flows} map.
* @param key The key in the flows map
* @param value The associated value in the flows map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putFlows(String key, HdesComposer.ComposerEntity value) {
this.flows.put(key, value);
return this;
}
/**
* Put one entry to the {@link HdesComposer.ComposerState#getFlows() flows} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putFlows(Map.Entry> entry) {
this.flows.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link HdesComposer.ComposerState#getFlows() flows} map. Nulls are not permitted
* @param entries The entries that will be added to the flows map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("flows")
public final Builder flows(Map> entries) {
this.flows = ImmutableMap.builder();
return putAllFlows(entries);
}
/**
* Put all mappings from the specified map as entries to {@link HdesComposer.ComposerState#getFlows() flows} map. Nulls are not permitted
* @param entries The entries that will be added to the flows map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllFlows(Map> entries) {
this.flows.putAll(entries);
return this;
}
/**
* Put one entry to the {@link HdesComposer.ComposerState#getServices() services} map.
* @param key The key in the services map
* @param value The associated value in the services map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putServices(String key, HdesComposer.ComposerEntity value) {
this.services.put(key, value);
return this;
}
/**
* Put one entry to the {@link HdesComposer.ComposerState#getServices() services} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putServices(Map.Entry> entry) {
this.services.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link HdesComposer.ComposerState#getServices() services} map. Nulls are not permitted
* @param entries The entries that will be added to the services map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("services")
public final Builder services(Map> entries) {
this.services = ImmutableMap.builder();
return putAllServices(entries);
}
/**
* Put all mappings from the specified map as entries to {@link HdesComposer.ComposerState#getServices() services} map. Nulls are not permitted
* @param entries The entries that will be added to the services map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllServices(Map> entries) {
this.services.putAll(entries);
return this;
}
/**
* Put one entry to the {@link HdesComposer.ComposerState#getDecisions() decisions} map.
* @param key The key in the decisions map
* @param value The associated value in the decisions map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putDecisions(String key, HdesComposer.ComposerEntity value) {
this.decisions.put(key, value);
return this;
}
/**
* Put one entry to the {@link HdesComposer.ComposerState#getDecisions() decisions} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putDecisions(Map.Entry> entry) {
this.decisions.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link HdesComposer.ComposerState#getDecisions() decisions} map. Nulls are not permitted
* @param entries The entries that will be added to the decisions map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("decisions")
public final Builder decisions(Map> entries) {
this.decisions = ImmutableMap.builder();
return putAllDecisions(entries);
}
/**
* Put all mappings from the specified map as entries to {@link HdesComposer.ComposerState#getDecisions() decisions} map. Nulls are not permitted
* @param entries The entries that will be added to the decisions map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllDecisions(Map> entries) {
this.decisions.putAll(entries);
return this;
}
/**
* Put one entry to the {@link HdesComposer.ComposerState#getBranches() branches} map.
* @param key The key in the branches map
* @param value The associated value in the branches map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putBranches(String key, HdesComposer.ComposerEntity value) {
this.branches.put(key, value);
return this;
}
/**
* Put one entry to the {@link HdesComposer.ComposerState#getBranches() branches} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putBranches(Map.Entry> entry) {
this.branches.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link HdesComposer.ComposerState#getBranches() branches} map. Nulls are not permitted
* @param entries The entries that will be added to the branches map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("branches")
public final Builder branches(Map> entries) {
this.branches = ImmutableMap.builder();
return putAllBranches(entries);
}
/**
* Put all mappings from the specified map as entries to {@link HdesComposer.ComposerState#getBranches() branches} map. Nulls are not permitted
* @param entries The entries that will be added to the branches map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllBranches(Map> entries) {
this.branches.putAll(entries);
return this;
}
/**
* Builds a new {@link ImmutableComposerState ImmutableComposerState}.
* @return An immutable instance of ComposerState
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableComposerState build() {
return new ImmutableComposerState(tags.build(), flows.build(), services.build(), decisions.build(), branches.build());
}
}
}