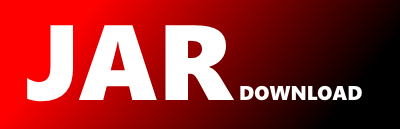
io.resys.hdes.client.api.programs.ImmutableFlowExecutionLog Maven / Gradle / Ivy
package io.resys.hdes.client.api.programs;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableMap;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.io.Serializable;
import java.util.Map;
import java.util.Objects;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link Program.FlowExecutionLog}.
*
* Use the builder to create immutable instances:
* {@code ImmutableFlowExecutionLog.builder()}.
*/
@Generated(from = "Program.FlowExecutionLog", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableFlowExecutionLog
implements Program.FlowExecutionLog {
private final ImmutableMap accepts;
private final ImmutableMap steps;
private ImmutableFlowExecutionLog(
ImmutableMap accepts,
ImmutableMap steps) {
this.accepts = accepts;
this.steps = steps;
}
/**
* @return The value of the {@code accepts} attribute
*/
@JsonProperty("accepts")
@Override
public ImmutableMap getAccepts() {
return accepts;
}
/**
* @return The value of the {@code steps} attribute
*/
@JsonProperty("steps")
@Override
public ImmutableMap getSteps() {
return steps;
}
/**
* Copy the current immutable object by replacing the {@link Program.FlowExecutionLog#getAccepts() accepts} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the accepts map
* @return A modified copy of {@code this} object
*/
public final ImmutableFlowExecutionLog withAccepts(Map entries) {
if (this.accepts == entries) return this;
ImmutableMap newValue = ImmutableMap.copyOf(entries);
return new ImmutableFlowExecutionLog(newValue, this.steps);
}
/**
* Copy the current immutable object by replacing the {@link Program.FlowExecutionLog#getSteps() steps} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the steps map
* @return A modified copy of {@code this} object
*/
public final ImmutableFlowExecutionLog withSteps(Map entries) {
if (this.steps == entries) return this;
ImmutableMap newValue = ImmutableMap.copyOf(entries);
return new ImmutableFlowExecutionLog(this.accepts, newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableFlowExecutionLog} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableFlowExecutionLog
&& equalTo((ImmutableFlowExecutionLog) another);
}
private boolean equalTo(ImmutableFlowExecutionLog another) {
return accepts.equals(another.accepts)
&& steps.equals(another.steps);
}
/**
* Computes a hash code from attributes: {@code accepts}, {@code steps}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + accepts.hashCode();
h += (h << 5) + steps.hashCode();
return h;
}
/**
* Prints the immutable value {@code FlowExecutionLog} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("FlowExecutionLog")
.omitNullValues()
.add("accepts", accepts)
.add("steps", steps)
.toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "Program.FlowExecutionLog", generator = "Immutables")
@Deprecated
@SuppressWarnings("Immutable")
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements Program.FlowExecutionLog {
@Nullable Map accepts = ImmutableMap.of();
@Nullable Map steps = ImmutableMap.of();
@JsonProperty("accepts")
public void setAccepts(Map accepts) {
this.accepts = accepts;
}
@JsonProperty("steps")
public void setSteps(Map steps) {
this.steps = steps;
}
@Override
public Map getAccepts() { throw new UnsupportedOperationException(); }
@Override
public Map getSteps() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableFlowExecutionLog fromJson(Json json) {
ImmutableFlowExecutionLog.Builder builder = ImmutableFlowExecutionLog.builder();
if (json.accepts != null) {
builder.putAllAccepts(json.accepts);
}
if (json.steps != null) {
builder.putAllSteps(json.steps);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link Program.FlowExecutionLog} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable FlowExecutionLog instance
*/
public static ImmutableFlowExecutionLog copyOf(Program.FlowExecutionLog instance) {
if (instance instanceof ImmutableFlowExecutionLog) {
return (ImmutableFlowExecutionLog) instance;
}
return ImmutableFlowExecutionLog.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableFlowExecutionLog ImmutableFlowExecutionLog}.
*
* ImmutableFlowExecutionLog.builder()
* .putAccepts|putAllAccepts(String => java.io.Serializable) // {@link Program.FlowExecutionLog#getAccepts() accepts} mappings
* .putSteps|putAllSteps(String => io.resys.hdes.client.api.programs.FlowProgram.FlowResultLog) // {@link Program.FlowExecutionLog#getSteps() steps} mappings
* .build();
*
* @return A new ImmutableFlowExecutionLog builder
*/
public static ImmutableFlowExecutionLog.Builder builder() {
return new ImmutableFlowExecutionLog.Builder();
}
/**
* Builds instances of type {@link ImmutableFlowExecutionLog ImmutableFlowExecutionLog}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "Program.FlowExecutionLog", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private ImmutableMap.Builder accepts = ImmutableMap.builder();
private ImmutableMap.Builder steps = ImmutableMap.builder();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code FlowExecutionLog} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(Program.FlowExecutionLog instance) {
Objects.requireNonNull(instance, "instance");
putAllAccepts(instance.getAccepts());
putAllSteps(instance.getSteps());
return this;
}
/**
* Put one entry to the {@link Program.FlowExecutionLog#getAccepts() accepts} map.
* @param key The key in the accepts map
* @param value The associated value in the accepts map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAccepts(String key, Serializable value) {
this.accepts.put(key, value);
return this;
}
/**
* Put one entry to the {@link Program.FlowExecutionLog#getAccepts() accepts} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAccepts(Map.Entry entry) {
this.accepts.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link Program.FlowExecutionLog#getAccepts() accepts} map. Nulls are not permitted
* @param entries The entries that will be added to the accepts map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("accepts")
public final Builder accepts(Map entries) {
this.accepts = ImmutableMap.builder();
return putAllAccepts(entries);
}
/**
* Put all mappings from the specified map as entries to {@link Program.FlowExecutionLog#getAccepts() accepts} map. Nulls are not permitted
* @param entries The entries that will be added to the accepts map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllAccepts(Map entries) {
this.accepts.putAll(entries);
return this;
}
/**
* Put one entry to the {@link Program.FlowExecutionLog#getSteps() steps} map.
* @param key The key in the steps map
* @param value The associated value in the steps map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putSteps(String key, FlowProgram.FlowResultLog value) {
this.steps.put(key, value);
return this;
}
/**
* Put one entry to the {@link Program.FlowExecutionLog#getSteps() steps} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putSteps(Map.Entry entry) {
this.steps.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link Program.FlowExecutionLog#getSteps() steps} map. Nulls are not permitted
* @param entries The entries that will be added to the steps map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("steps")
public final Builder steps(Map entries) {
this.steps = ImmutableMap.builder();
return putAllSteps(entries);
}
/**
* Put all mappings from the specified map as entries to {@link Program.FlowExecutionLog#getSteps() steps} map. Nulls are not permitted
* @param entries The entries that will be added to the steps map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllSteps(Map entries) {
this.steps.putAll(entries);
return this;
}
/**
* Builds a new {@link ImmutableFlowExecutionLog ImmutableFlowExecutionLog}.
* @return An immutable instance of FlowExecutionLog
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableFlowExecutionLog build() {
return new ImmutableFlowExecutionLog(accepts.build(), steps.build());
}
}
}