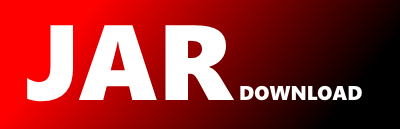
io.resys.hdes.client.spi.ImmutableGitConfig Maven / Gradle / Ivy
package io.resys.hdes.client.spi;
import com.google.common.base.MoreObjects;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import io.resys.hdes.client.api.HdesStore;
import io.resys.hdes.client.spi.staticresources.StoreEntityLocation;
import java.nio.file.Path;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.eclipse.jgit.api.Git;
import org.eclipse.jgit.api.TransportConfigCallback;
import org.ehcache.CacheManager;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link GitConfig}.
*
* Use the builder to create immutable instances:
* {@code ImmutableGitConfig.builder()}.
*/
@Generated(from = "GitConfig", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableGitConfig implements GitConfig {
private final GitConfig.GitInit init;
private final StoreEntityLocation location;
private final GitConfig.GitSerializer serializer;
private final HdesStore.HdesCredsSupplier creds;
private final CacheManager cacheManager;
private final String cacheName;
private final Integer cacheHeap;
private final String assetsPath;
private final Path parentPath;
private final String absolutePath;
private final String absoluteAssetsPath;
private final TransportConfigCallback callback;
private final Git client;
private ImmutableGitConfig(
GitConfig.GitInit init,
StoreEntityLocation location,
GitConfig.GitSerializer serializer,
HdesStore.HdesCredsSupplier creds,
CacheManager cacheManager,
String cacheName,
Integer cacheHeap,
String assetsPath,
Path parentPath,
String absolutePath,
String absoluteAssetsPath,
TransportConfigCallback callback,
Git client) {
this.init = init;
this.location = location;
this.serializer = serializer;
this.creds = creds;
this.cacheManager = cacheManager;
this.cacheName = cacheName;
this.cacheHeap = cacheHeap;
this.assetsPath = assetsPath;
this.parentPath = parentPath;
this.absolutePath = absolutePath;
this.absoluteAssetsPath = absoluteAssetsPath;
this.callback = callback;
this.client = client;
}
/**
* @return The value of the {@code init} attribute
*/
@Override
public GitConfig.GitInit getInit() {
return init;
}
/**
* @return The value of the {@code location} attribute
*/
@Override
public StoreEntityLocation getLocation() {
return location;
}
/**
* @return The value of the {@code serializer} attribute
*/
@Override
public GitConfig.GitSerializer getSerializer() {
return serializer;
}
/**
* @return The value of the {@code creds} attribute
*/
@Override
public HdesStore.HdesCredsSupplier getCreds() {
return creds;
}
/**
* @return The value of the {@code cacheManager} attribute
*/
@Override
public CacheManager getCacheManager() {
return cacheManager;
}
/**
* @return The value of the {@code cacheName} attribute
*/
@Override
public String getCacheName() {
return cacheName;
}
/**
* @return The value of the {@code cacheHeap} attribute
*/
@Override
public Integer getCacheHeap() {
return cacheHeap;
}
/**
* @return The value of the {@code assetsPath} attribute
*/
@Override
public String getAssetsPath() {
return assetsPath;
}
/**
* @return The value of the {@code parentPath} attribute
*/
@Override
public Path getParentPath() {
return parentPath;
}
/**
* @return The value of the {@code absolutePath} attribute
*/
@Override
public String getAbsolutePath() {
return absolutePath;
}
/**
* @return The value of the {@code absoluteAssetsPath} attribute
*/
@Override
public String getAbsoluteAssetsPath() {
return absoluteAssetsPath;
}
/**
* @return The value of the {@code callback} attribute
*/
@Override
public TransportConfigCallback getCallback() {
return callback;
}
/**
* @return The value of the {@code client} attribute
*/
@Override
public Git getClient() {
return client;
}
/**
* Copy the current immutable object by setting a value for the {@link GitConfig#getInit() init} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for init
* @return A modified copy of the {@code this} object
*/
public final ImmutableGitConfig withInit(GitConfig.GitInit value) {
if (this.init == value) return this;
GitConfig.GitInit newValue = Objects.requireNonNull(value, "init");
return new ImmutableGitConfig(
newValue,
this.location,
this.serializer,
this.creds,
this.cacheManager,
this.cacheName,
this.cacheHeap,
this.assetsPath,
this.parentPath,
this.absolutePath,
this.absoluteAssetsPath,
this.callback,
this.client);
}
/**
* Copy the current immutable object by setting a value for the {@link GitConfig#getLocation() location} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for location
* @return A modified copy of the {@code this} object
*/
public final ImmutableGitConfig withLocation(StoreEntityLocation value) {
if (this.location == value) return this;
StoreEntityLocation newValue = Objects.requireNonNull(value, "location");
return new ImmutableGitConfig(
this.init,
newValue,
this.serializer,
this.creds,
this.cacheManager,
this.cacheName,
this.cacheHeap,
this.assetsPath,
this.parentPath,
this.absolutePath,
this.absoluteAssetsPath,
this.callback,
this.client);
}
/**
* Copy the current immutable object by setting a value for the {@link GitConfig#getSerializer() serializer} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for serializer
* @return A modified copy of the {@code this} object
*/
public final ImmutableGitConfig withSerializer(GitConfig.GitSerializer value) {
if (this.serializer == value) return this;
GitConfig.GitSerializer newValue = Objects.requireNonNull(value, "serializer");
return new ImmutableGitConfig(
this.init,
this.location,
newValue,
this.creds,
this.cacheManager,
this.cacheName,
this.cacheHeap,
this.assetsPath,
this.parentPath,
this.absolutePath,
this.absoluteAssetsPath,
this.callback,
this.client);
}
/**
* Copy the current immutable object by setting a value for the {@link GitConfig#getCreds() creds} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for creds
* @return A modified copy of the {@code this} object
*/
public final ImmutableGitConfig withCreds(HdesStore.HdesCredsSupplier value) {
if (this.creds == value) return this;
HdesStore.HdesCredsSupplier newValue = Objects.requireNonNull(value, "creds");
return new ImmutableGitConfig(
this.init,
this.location,
this.serializer,
newValue,
this.cacheManager,
this.cacheName,
this.cacheHeap,
this.assetsPath,
this.parentPath,
this.absolutePath,
this.absoluteAssetsPath,
this.callback,
this.client);
}
/**
* Copy the current immutable object by setting a value for the {@link GitConfig#getCacheManager() cacheManager} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for cacheManager
* @return A modified copy of the {@code this} object
*/
public final ImmutableGitConfig withCacheManager(CacheManager value) {
if (this.cacheManager == value) return this;
CacheManager newValue = Objects.requireNonNull(value, "cacheManager");
return new ImmutableGitConfig(
this.init,
this.location,
this.serializer,
this.creds,
newValue,
this.cacheName,
this.cacheHeap,
this.assetsPath,
this.parentPath,
this.absolutePath,
this.absoluteAssetsPath,
this.callback,
this.client);
}
/**
* Copy the current immutable object by setting a value for the {@link GitConfig#getCacheName() cacheName} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for cacheName
* @return A modified copy of the {@code this} object
*/
public final ImmutableGitConfig withCacheName(String value) {
String newValue = Objects.requireNonNull(value, "cacheName");
if (this.cacheName.equals(newValue)) return this;
return new ImmutableGitConfig(
this.init,
this.location,
this.serializer,
this.creds,
this.cacheManager,
newValue,
this.cacheHeap,
this.assetsPath,
this.parentPath,
this.absolutePath,
this.absoluteAssetsPath,
this.callback,
this.client);
}
/**
* Copy the current immutable object by setting a value for the {@link GitConfig#getCacheHeap() cacheHeap} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for cacheHeap
* @return A modified copy of the {@code this} object
*/
public final ImmutableGitConfig withCacheHeap(Integer value) {
Integer newValue = Objects.requireNonNull(value, "cacheHeap");
if (this.cacheHeap.equals(newValue)) return this;
return new ImmutableGitConfig(
this.init,
this.location,
this.serializer,
this.creds,
this.cacheManager,
this.cacheName,
newValue,
this.assetsPath,
this.parentPath,
this.absolutePath,
this.absoluteAssetsPath,
this.callback,
this.client);
}
/**
* Copy the current immutable object by setting a value for the {@link GitConfig#getAssetsPath() assetsPath} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for assetsPath
* @return A modified copy of the {@code this} object
*/
public final ImmutableGitConfig withAssetsPath(String value) {
String newValue = Objects.requireNonNull(value, "assetsPath");
if (this.assetsPath.equals(newValue)) return this;
return new ImmutableGitConfig(
this.init,
this.location,
this.serializer,
this.creds,
this.cacheManager,
this.cacheName,
this.cacheHeap,
newValue,
this.parentPath,
this.absolutePath,
this.absoluteAssetsPath,
this.callback,
this.client);
}
/**
* Copy the current immutable object by setting a value for the {@link GitConfig#getParentPath() parentPath} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for parentPath
* @return A modified copy of the {@code this} object
*/
public final ImmutableGitConfig withParentPath(Path value) {
if (this.parentPath == value) return this;
Path newValue = Objects.requireNonNull(value, "parentPath");
return new ImmutableGitConfig(
this.init,
this.location,
this.serializer,
this.creds,
this.cacheManager,
this.cacheName,
this.cacheHeap,
this.assetsPath,
newValue,
this.absolutePath,
this.absoluteAssetsPath,
this.callback,
this.client);
}
/**
* Copy the current immutable object by setting a value for the {@link GitConfig#getAbsolutePath() absolutePath} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for absolutePath
* @return A modified copy of the {@code this} object
*/
public final ImmutableGitConfig withAbsolutePath(String value) {
String newValue = Objects.requireNonNull(value, "absolutePath");
if (this.absolutePath.equals(newValue)) return this;
return new ImmutableGitConfig(
this.init,
this.location,
this.serializer,
this.creds,
this.cacheManager,
this.cacheName,
this.cacheHeap,
this.assetsPath,
this.parentPath,
newValue,
this.absoluteAssetsPath,
this.callback,
this.client);
}
/**
* Copy the current immutable object by setting a value for the {@link GitConfig#getAbsoluteAssetsPath() absoluteAssetsPath} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for absoluteAssetsPath
* @return A modified copy of the {@code this} object
*/
public final ImmutableGitConfig withAbsoluteAssetsPath(String value) {
String newValue = Objects.requireNonNull(value, "absoluteAssetsPath");
if (this.absoluteAssetsPath.equals(newValue)) return this;
return new ImmutableGitConfig(
this.init,
this.location,
this.serializer,
this.creds,
this.cacheManager,
this.cacheName,
this.cacheHeap,
this.assetsPath,
this.parentPath,
this.absolutePath,
newValue,
this.callback,
this.client);
}
/**
* Copy the current immutable object by setting a value for the {@link GitConfig#getCallback() callback} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for callback
* @return A modified copy of the {@code this} object
*/
public final ImmutableGitConfig withCallback(TransportConfigCallback value) {
if (this.callback == value) return this;
TransportConfigCallback newValue = Objects.requireNonNull(value, "callback");
return new ImmutableGitConfig(
this.init,
this.location,
this.serializer,
this.creds,
this.cacheManager,
this.cacheName,
this.cacheHeap,
this.assetsPath,
this.parentPath,
this.absolutePath,
this.absoluteAssetsPath,
newValue,
this.client);
}
/**
* Copy the current immutable object by setting a value for the {@link GitConfig#getClient() client} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for client
* @return A modified copy of the {@code this} object
*/
public final ImmutableGitConfig withClient(Git value) {
if (this.client == value) return this;
Git newValue = Objects.requireNonNull(value, "client");
return new ImmutableGitConfig(
this.init,
this.location,
this.serializer,
this.creds,
this.cacheManager,
this.cacheName,
this.cacheHeap,
this.assetsPath,
this.parentPath,
this.absolutePath,
this.absoluteAssetsPath,
this.callback,
newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableGitConfig} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableGitConfig
&& equalTo((ImmutableGitConfig) another);
}
private boolean equalTo(ImmutableGitConfig another) {
return init.equals(another.init)
&& location.equals(another.location)
&& serializer.equals(another.serializer)
&& creds.equals(another.creds)
&& cacheManager.equals(another.cacheManager)
&& cacheName.equals(another.cacheName)
&& cacheHeap.equals(another.cacheHeap)
&& assetsPath.equals(another.assetsPath)
&& parentPath.equals(another.parentPath)
&& absolutePath.equals(another.absolutePath)
&& absoluteAssetsPath.equals(another.absoluteAssetsPath)
&& callback.equals(another.callback)
&& client.equals(another.client);
}
/**
* Computes a hash code from attributes: {@code init}, {@code location}, {@code serializer}, {@code creds}, {@code cacheManager}, {@code cacheName}, {@code cacheHeap}, {@code assetsPath}, {@code parentPath}, {@code absolutePath}, {@code absoluteAssetsPath}, {@code callback}, {@code client}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + init.hashCode();
h += (h << 5) + location.hashCode();
h += (h << 5) + serializer.hashCode();
h += (h << 5) + creds.hashCode();
h += (h << 5) + cacheManager.hashCode();
h += (h << 5) + cacheName.hashCode();
h += (h << 5) + cacheHeap.hashCode();
h += (h << 5) + assetsPath.hashCode();
h += (h << 5) + parentPath.hashCode();
h += (h << 5) + absolutePath.hashCode();
h += (h << 5) + absoluteAssetsPath.hashCode();
h += (h << 5) + callback.hashCode();
h += (h << 5) + client.hashCode();
return h;
}
/**
* Prints the immutable value {@code GitConfig} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("GitConfig")
.omitNullValues()
.add("init", init)
.add("location", location)
.add("serializer", serializer)
.add("creds", creds)
.add("cacheManager", cacheManager)
.add("cacheName", cacheName)
.add("cacheHeap", cacheHeap)
.add("assetsPath", assetsPath)
.add("parentPath", parentPath)
.add("absolutePath", absolutePath)
.add("absoluteAssetsPath", absoluteAssetsPath)
.add("callback", callback)
.add("client", client)
.toString();
}
/**
* Creates an immutable copy of a {@link GitConfig} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable GitConfig instance
*/
public static ImmutableGitConfig copyOf(GitConfig instance) {
if (instance instanceof ImmutableGitConfig) {
return (ImmutableGitConfig) instance;
}
return ImmutableGitConfig.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableGitConfig ImmutableGitConfig}.
*
* ImmutableGitConfig.builder()
* .init(io.resys.hdes.client.spi.GitConfig.GitInit) // required {@link GitConfig#getInit() init}
* .location(io.resys.hdes.client.spi.staticresources.StoreEntityLocation) // required {@link GitConfig#getLocation() location}
* .serializer(io.resys.hdes.client.spi.GitConfig.GitSerializer) // required {@link GitConfig#getSerializer() serializer}
* .creds(io.resys.hdes.client.api.HdesStore.HdesCredsSupplier) // required {@link GitConfig#getCreds() creds}
* .cacheManager(org.ehcache.CacheManager) // required {@link GitConfig#getCacheManager() cacheManager}
* .cacheName(String) // required {@link GitConfig#getCacheName() cacheName}
* .cacheHeap(Integer) // required {@link GitConfig#getCacheHeap() cacheHeap}
* .assetsPath(String) // required {@link GitConfig#getAssetsPath() assetsPath}
* .parentPath(java.nio.file.Path) // required {@link GitConfig#getParentPath() parentPath}
* .absolutePath(String) // required {@link GitConfig#getAbsolutePath() absolutePath}
* .absoluteAssetsPath(String) // required {@link GitConfig#getAbsoluteAssetsPath() absoluteAssetsPath}
* .callback(org.eclipse.jgit.api.TransportConfigCallback) // required {@link GitConfig#getCallback() callback}
* .client(org.eclipse.jgit.api.Git) // required {@link GitConfig#getClient() client}
* .build();
*
* @return A new ImmutableGitConfig builder
*/
public static ImmutableGitConfig.Builder builder() {
return new ImmutableGitConfig.Builder();
}
/**
* Builds instances of type {@link ImmutableGitConfig ImmutableGitConfig}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "GitConfig", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_INIT = 0x1L;
private static final long INIT_BIT_LOCATION = 0x2L;
private static final long INIT_BIT_SERIALIZER = 0x4L;
private static final long INIT_BIT_CREDS = 0x8L;
private static final long INIT_BIT_CACHE_MANAGER = 0x10L;
private static final long INIT_BIT_CACHE_NAME = 0x20L;
private static final long INIT_BIT_CACHE_HEAP = 0x40L;
private static final long INIT_BIT_ASSETS_PATH = 0x80L;
private static final long INIT_BIT_PARENT_PATH = 0x100L;
private static final long INIT_BIT_ABSOLUTE_PATH = 0x200L;
private static final long INIT_BIT_ABSOLUTE_ASSETS_PATH = 0x400L;
private static final long INIT_BIT_CALLBACK = 0x800L;
private static final long INIT_BIT_CLIENT = 0x1000L;
private long initBits = 0x1fffL;
private @Nullable GitConfig.GitInit init;
private @Nullable StoreEntityLocation location;
private @Nullable GitConfig.GitSerializer serializer;
private @Nullable HdesStore.HdesCredsSupplier creds;
private @Nullable CacheManager cacheManager;
private @Nullable String cacheName;
private @Nullable Integer cacheHeap;
private @Nullable String assetsPath;
private @Nullable Path parentPath;
private @Nullable String absolutePath;
private @Nullable String absoluteAssetsPath;
private @Nullable TransportConfigCallback callback;
private @Nullable Git client;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code GitConfig} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(GitConfig instance) {
Objects.requireNonNull(instance, "instance");
init(instance.getInit());
location(instance.getLocation());
serializer(instance.getSerializer());
creds(instance.getCreds());
cacheManager(instance.getCacheManager());
cacheName(instance.getCacheName());
cacheHeap(instance.getCacheHeap());
assetsPath(instance.getAssetsPath());
parentPath(instance.getParentPath());
absolutePath(instance.getAbsolutePath());
absoluteAssetsPath(instance.getAbsoluteAssetsPath());
callback(instance.getCallback());
client(instance.getClient());
return this;
}
/**
* Initializes the value for the {@link GitConfig#getInit() init} attribute.
* @param init The value for init
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder init(GitConfig.GitInit init) {
this.init = Objects.requireNonNull(init, "init");
initBits &= ~INIT_BIT_INIT;
return this;
}
/**
* Initializes the value for the {@link GitConfig#getLocation() location} attribute.
* @param location The value for location
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder location(StoreEntityLocation location) {
this.location = Objects.requireNonNull(location, "location");
initBits &= ~INIT_BIT_LOCATION;
return this;
}
/**
* Initializes the value for the {@link GitConfig#getSerializer() serializer} attribute.
* @param serializer The value for serializer
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder serializer(GitConfig.GitSerializer serializer) {
this.serializer = Objects.requireNonNull(serializer, "serializer");
initBits &= ~INIT_BIT_SERIALIZER;
return this;
}
/**
* Initializes the value for the {@link GitConfig#getCreds() creds} attribute.
* @param creds The value for creds
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder creds(HdesStore.HdesCredsSupplier creds) {
this.creds = Objects.requireNonNull(creds, "creds");
initBits &= ~INIT_BIT_CREDS;
return this;
}
/**
* Initializes the value for the {@link GitConfig#getCacheManager() cacheManager} attribute.
* @param cacheManager The value for cacheManager
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder cacheManager(CacheManager cacheManager) {
this.cacheManager = Objects.requireNonNull(cacheManager, "cacheManager");
initBits &= ~INIT_BIT_CACHE_MANAGER;
return this;
}
/**
* Initializes the value for the {@link GitConfig#getCacheName() cacheName} attribute.
* @param cacheName The value for cacheName
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder cacheName(String cacheName) {
this.cacheName = Objects.requireNonNull(cacheName, "cacheName");
initBits &= ~INIT_BIT_CACHE_NAME;
return this;
}
/**
* Initializes the value for the {@link GitConfig#getCacheHeap() cacheHeap} attribute.
* @param cacheHeap The value for cacheHeap
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder cacheHeap(Integer cacheHeap) {
this.cacheHeap = Objects.requireNonNull(cacheHeap, "cacheHeap");
initBits &= ~INIT_BIT_CACHE_HEAP;
return this;
}
/**
* Initializes the value for the {@link GitConfig#getAssetsPath() assetsPath} attribute.
* @param assetsPath The value for assetsPath
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder assetsPath(String assetsPath) {
this.assetsPath = Objects.requireNonNull(assetsPath, "assetsPath");
initBits &= ~INIT_BIT_ASSETS_PATH;
return this;
}
/**
* Initializes the value for the {@link GitConfig#getParentPath() parentPath} attribute.
* @param parentPath The value for parentPath
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder parentPath(Path parentPath) {
this.parentPath = Objects.requireNonNull(parentPath, "parentPath");
initBits &= ~INIT_BIT_PARENT_PATH;
return this;
}
/**
* Initializes the value for the {@link GitConfig#getAbsolutePath() absolutePath} attribute.
* @param absolutePath The value for absolutePath
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder absolutePath(String absolutePath) {
this.absolutePath = Objects.requireNonNull(absolutePath, "absolutePath");
initBits &= ~INIT_BIT_ABSOLUTE_PATH;
return this;
}
/**
* Initializes the value for the {@link GitConfig#getAbsoluteAssetsPath() absoluteAssetsPath} attribute.
* @param absoluteAssetsPath The value for absoluteAssetsPath
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder absoluteAssetsPath(String absoluteAssetsPath) {
this.absoluteAssetsPath = Objects.requireNonNull(absoluteAssetsPath, "absoluteAssetsPath");
initBits &= ~INIT_BIT_ABSOLUTE_ASSETS_PATH;
return this;
}
/**
* Initializes the value for the {@link GitConfig#getCallback() callback} attribute.
* @param callback The value for callback
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder callback(TransportConfigCallback callback) {
this.callback = Objects.requireNonNull(callback, "callback");
initBits &= ~INIT_BIT_CALLBACK;
return this;
}
/**
* Initializes the value for the {@link GitConfig#getClient() client} attribute.
* @param client The value for client
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder client(Git client) {
this.client = Objects.requireNonNull(client, "client");
initBits &= ~INIT_BIT_CLIENT;
return this;
}
/**
* Builds a new {@link ImmutableGitConfig ImmutableGitConfig}.
* @return An immutable instance of GitConfig
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableGitConfig build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableGitConfig(
init,
location,
serializer,
creds,
cacheManager,
cacheName,
cacheHeap,
assetsPath,
parentPath,
absolutePath,
absoluteAssetsPath,
callback,
client);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_INIT) != 0) attributes.add("init");
if ((initBits & INIT_BIT_LOCATION) != 0) attributes.add("location");
if ((initBits & INIT_BIT_SERIALIZER) != 0) attributes.add("serializer");
if ((initBits & INIT_BIT_CREDS) != 0) attributes.add("creds");
if ((initBits & INIT_BIT_CACHE_MANAGER) != 0) attributes.add("cacheManager");
if ((initBits & INIT_BIT_CACHE_NAME) != 0) attributes.add("cacheName");
if ((initBits & INIT_BIT_CACHE_HEAP) != 0) attributes.add("cacheHeap");
if ((initBits & INIT_BIT_ASSETS_PATH) != 0) attributes.add("assetsPath");
if ((initBits & INIT_BIT_PARENT_PATH) != 0) attributes.add("parentPath");
if ((initBits & INIT_BIT_ABSOLUTE_PATH) != 0) attributes.add("absolutePath");
if ((initBits & INIT_BIT_ABSOLUTE_ASSETS_PATH) != 0) attributes.add("absoluteAssetsPath");
if ((initBits & INIT_BIT_CALLBACK) != 0) attributes.add("callback");
if ((initBits & INIT_BIT_CLIENT) != 0) attributes.add("client");
return "Cannot build GitConfig, some of required attributes are not set " + attributes;
}
}
}