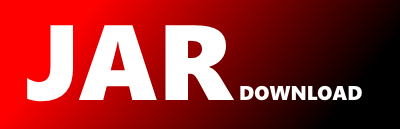
io.rocketbase.commons.resource.ValidationResource Maven / Gradle / Ivy
package io.rocketbase.commons.resource;
import io.rocketbase.commons.dto.validation.*;
import org.springframework.core.ParameterizedTypeReference;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpMethod;
import org.springframework.http.ResponseEntity;
import org.springframework.util.Assert;
import org.springframework.web.client.RestTemplate;
public class ValidationResource implements BaseRestResource {
protected String baseAuthApiUrl;
protected RestTemplate restTemplate;
public ValidationResource(String baseAuthApiUrl) {
this(baseAuthApiUrl, null);
}
public ValidationResource(String baseAuthApiUrl, RestTemplate restTemplate) {
Assert.hasText(baseAuthApiUrl, "baseAuthApiUrl is required");
this.baseAuthApiUrl = baseAuthApiUrl;
this.restTemplate = restTemplate;
}
protected RestTemplate getRestTemplate() {
if (restTemplate == null) {
restTemplate = new RestTemplate();
restTemplate.setErrorHandler(new BasicResponseErrorHandler());
}
return restTemplate;
}
public ValidationResponse validatePassword(String password) {
ResponseEntity> response = getRestTemplate()
.exchange(createUriComponentsBuilder(baseAuthApiUrl)
.path("/auth/validate/password").toUriString(),
HttpMethod.POST,
new HttpEntity<>(password, createHeaderWithLanguage()),
new ParameterizedTypeReference>() {
});
return response.getBody();
}
public ValidationResponse validateUsername(String username) {
ResponseEntity> response = getRestTemplate()
.exchange(createUriComponentsBuilder(baseAuthApiUrl)
.path("/auth/validate/username").toUriString(),
HttpMethod.POST,
new HttpEntity<>(username, createHeaderWithLanguage()),
new ParameterizedTypeReference>() {
});
return response.getBody();
}
public ValidationResponse validateEmail(String email) {
ResponseEntity> response = getRestTemplate()
.exchange(createUriComponentsBuilder(baseAuthApiUrl)
.path("/auth/validate/email").toUriString(),
HttpMethod.POST,
new HttpEntity<>(email, createHeaderWithLanguage()),
new ParameterizedTypeReference>() {
});
return response.getBody();
}
public ValidationResponse validateToken(String token) {
ResponseEntity> response = getRestTemplate()
.exchange(createUriComponentsBuilder(baseAuthApiUrl)
.path("/auth/validate/token").toUriString(),
HttpMethod.POST,
new HttpEntity<>(token, createHeaderWithLanguage()),
new ParameterizedTypeReference>() {
});
return response.getBody();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy