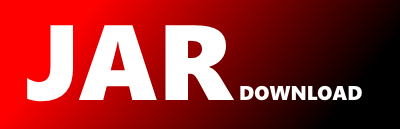
io.rsocket.internal.UnicastMonoEmpty Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rsocket-core Show documentation
Show all versions of rsocket-core Show documentation
Core functionality for the RSocket library
package io.rsocket.internal;
import java.time.Duration;
import java.util.concurrent.atomic.AtomicIntegerFieldUpdater;
import reactor.core.CoreSubscriber;
import reactor.core.Scannable;
import reactor.core.publisher.Mono;
import reactor.core.publisher.Operators;
import reactor.util.annotation.Nullable;
/**
* Represents an empty publisher which only calls onSubscribe and onComplete and allows only a
* single subscriber.
*
* @see Reactive-Streams-Commons
*/
public final class UnicastMonoEmpty extends Mono
© 2015 - 2025 Weber Informatics LLC | Privacy Policy