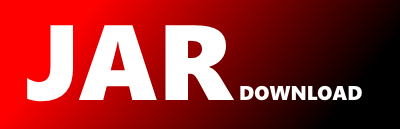
io.rsocket.plugins.InterceptorRegistry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rsocket-core Show documentation
Show all versions of rsocket-core Show documentation
Core functionality for the RSocket library
The newest version!
/*
* Copyright 2015-2020 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.rsocket.plugins;
import io.rsocket.RSocket;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Consumer;
import java.util.function.Function;
/**
* Provides support for registering interceptors at the following levels:
*
*
* - {@link #forConnection(DuplexConnectionInterceptor)} -- transport level
*
- {@link #forSocketAcceptor(SocketAcceptorInterceptor)} -- for accepting new connections
*
- {@link #forRequester(RSocketInterceptor)} -- for performing of requests
*
- {@link #forResponder(RSocketInterceptor)} -- for responding to requests
*
*/
public class InterceptorRegistry {
private List> requesterRequestInterceptors =
new ArrayList<>();
private List> responderRequestInterceptors =
new ArrayList<>();
private List requesterRSocketInterceptors = new ArrayList<>();
private List responderRSocketInterceptors = new ArrayList<>();
private List socketAcceptorInterceptors = new ArrayList<>();
private List connectionInterceptors = new ArrayList<>();
/**
* Add an {@link RequestInterceptor} that will hook into Requester RSocket requests' phases.
*
* @param interceptor a function which accepts an {@link RSocket} and returns a new {@link
* RequestInterceptor}
* @since 1.1
*/
public InterceptorRegistry forRequestsInRequester(
Function interceptor) {
requesterRequestInterceptors.add(interceptor);
return this;
}
/**
* Add an {@link RequestInterceptor} that will hook into Requester RSocket requests' phases.
*
* @param interceptor a function which accepts an {@link RSocket} and returns a new {@link
* RequestInterceptor}
* @since 1.1
*/
public InterceptorRegistry forRequestsInResponder(
Function interceptor) {
responderRequestInterceptors.add(interceptor);
return this;
}
/**
* Add an {@link RSocketInterceptor} that will decorate the RSocket used for performing requests.
*/
public InterceptorRegistry forRequester(RSocketInterceptor interceptor) {
requesterRSocketInterceptors.add(interceptor);
return this;
}
/**
* Variant of {@link #forRequester(RSocketInterceptor)} with access to the list of existing
* registrations.
*/
public InterceptorRegistry forRequester(Consumer> consumer) {
consumer.accept(requesterRSocketInterceptors);
return this;
}
/**
* Add an {@link RSocketInterceptor} that will decorate the RSocket used for resonding to
* requests.
*/
public InterceptorRegistry forResponder(RSocketInterceptor interceptor) {
responderRSocketInterceptors.add(interceptor);
return this;
}
/**
* Variant of {@link #forResponder(RSocketInterceptor)} with access to the list of existing
* registrations.
*/
public InterceptorRegistry forResponder(Consumer> consumer) {
consumer.accept(responderRSocketInterceptors);
return this;
}
/**
* Add a {@link SocketAcceptorInterceptor} that will intercept the accepting of new connections.
*/
public InterceptorRegistry forSocketAcceptor(SocketAcceptorInterceptor interceptor) {
socketAcceptorInterceptors.add(interceptor);
return this;
}
/**
* Variant of {@link #forSocketAcceptor(SocketAcceptorInterceptor)} with access to the list of
* existing registrations.
*/
public InterceptorRegistry forSocketAcceptor(Consumer> consumer) {
consumer.accept(socketAcceptorInterceptors);
return this;
}
/** Add a {@link DuplexConnectionInterceptor}. */
public InterceptorRegistry forConnection(DuplexConnectionInterceptor interceptor) {
connectionInterceptors.add(interceptor);
return this;
}
/**
* Variant of {@link #forConnection(DuplexConnectionInterceptor)} with access to the list of
* existing registrations.
*/
public InterceptorRegistry forConnection(Consumer> consumer) {
consumer.accept(connectionInterceptors);
return this;
}
List> getRequestInterceptorsForRequester() {
return requesterRequestInterceptors;
}
List> getRequestInterceptorsForResponder() {
return responderRequestInterceptors;
}
List getRequesterInterceptors() {
return requesterRSocketInterceptors;
}
List getResponderInterceptors() {
return responderRSocketInterceptors;
}
List getConnectionInterceptors() {
return connectionInterceptors;
}
List getSocketAcceptorInterceptors() {
return socketAcceptorInterceptors;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy