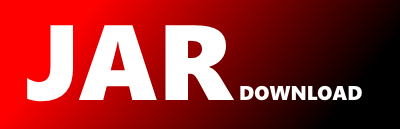
io.runon.collect.api.RunonCandleApi Maven / Gradle / Ivy
The newest version!
package io.runon.collect.api;
import io.runon.collect.api.code.RunonApiUrl;
import io.runon.collect.api.code.RunonStockType;
import io.runon.collect.api.dto.CandleDataResponse;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestTemplate;
/**
* @author ccsweets
* Candle API 호출
*/
public class RunonCandleApi {
/**
* 국내 주식 일봉 조회
* @param host API 호스트
* @param apiKey API 키
* @param stockCode 주식 코드
* @param startDate 조회 시작일
* @param size 조회 개수
* @return CandleDataResponse
*/
public static CandleDataResponse getDomesticDailyCandle(String host, String apiKey, String stockCode, String startDate, int size) {
return getCandleData(host, RunonApiUrl.CANDLE_DAILY, apiKey, stockCode, RunonStockType.KR, startDate, size);
}
/**
* 미국 주식 일봉 조회
* @param host API 호스트
* @param apiKey API 키
* @param stockCode 주식 코드
* @param startDate 조회 시작일
* @param size 조회 개수
* @return CandleDataResponse
*/
public static CandleDataResponse getUSADailyCandle(String host, String apiKey, String stockCode, String startDate, int size) {
return getCandleData(host, RunonApiUrl.CANDLE_DAILY, apiKey, stockCode, RunonStockType.US, startDate, size);
}
/**
* 국내 주식 일봉 전체 조회
* @param host API 호스트
* @param apiKey API 키
* @param stockCode 주식 코드
* @return CandleDataResponse
*/
public static CandleDataResponse getAllDomesticDailyCandle(String host, String apiKey, String stockCode) {
return getAllCandleData(host, RunonApiUrl.CANDLE_DAILY_ALL, apiKey, stockCode, RunonStockType.KR);
}
/**
* 미국 주식 일봉 전체 조회
* @param host API 호스트
* @param apiKey API 키
* @param stockCode 주식 코드
* @return CandleDataResponse
*/
public static CandleDataResponse getAllUSADailyCandle(String host, String apiKey, String stockCode) {
return getAllCandleData(host, RunonApiUrl.CANDLE_DAILY_ALL, apiKey, stockCode, RunonStockType.US);
}
/**
* 국내 주식 분봉 조회
* @param host API 호스트
* @param apiKey API 키
* @param stockCode 주식 코드
* @param startDate 조회 시작일
* @param size 조회 개수
* @return CandleDataResponse
*/
public static CandleDataResponse getDomesticMinuteCandle(String host, String apiKey, String stockCode, String startDate, int size) {
return getCandleData(host, RunonApiUrl.CANDLE_MINUTELY, apiKey, stockCode, RunonStockType.KR, startDate, size);
}
/**
* 국내 주식 분봉 전체 조회
* @param host API 호스트
* @param apiKey API 키
* @param stockCode 주식 코드
* @return CandleDataResponse
*/
public static CandleDataResponse getAllDomesticMinuteCandle(String host, String apiKey, String stockCode) {
return getAllCandleData(host, RunonApiUrl.CANDLE_MINUTELY_ALL, apiKey, stockCode, RunonStockType.KR);
}
/**
* 주식 캔들 조회
* @param host API 호스트
* @param url API URL
* @param apiKey API 키
* @param stockCode 주식 코드
* @param stockType 주식 타입
* @param startDate 조회 시작일
* @param size 조회 개수
* @return CandleDataResponse
*/
public static CandleDataResponse getCandleData(String host, String url, String apiKey, String stockCode, String stockType, String startDate, int size) {
RestTemplate restTemplate = new RestTemplate();
String getUrl = host + url + "?apiKey="+apiKey+"&stockCode=" + stockCode + "&stockType=" + stockType + "&startDate=" + startDate + "&size=" + size;
ResponseEntity response = restTemplate.getForEntity(getUrl, CandleDataResponse.class);
if(response.getStatusCode() == HttpStatus.OK) {
return response.getBody();
} else {
return CandleDataResponse.failOf(response.getStatusCode().toString());
}
}
/**
* 주식 캔들 전체 조회
* @param host API 호스트
* @param url API URL
* @param apiKey API 키
* @param stockCode 주식 코드
* @param stockType 주식 타입
* @return CandleDataResponse
*/
public static CandleDataResponse getAllCandleData(String host, String url, String apiKey, String stockCode, String stockType) {
RestTemplate restTemplate = new RestTemplate();
String getUrl = host + url + "?apiKey="+apiKey+"&stockCode=" + stockCode + "&stockType=" + stockType ;
ResponseEntity response = restTemplate.getForEntity(getUrl, CandleDataResponse.class);
if(response.getStatusCode() == HttpStatus.OK) {
return response.getBody();
} else {
return CandleDataResponse.failOf(response.getStatusCode().toString());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy