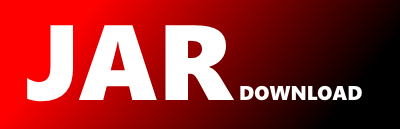
io.scalajs.dom.Event.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dom-html_sjs0.6_2.11 Show documentation
Show all versions of dom-html_sjs0.6_2.11 Show documentation
DOM/HTML bindings for Scala.js
package io.scalajs.dom
import scala.scalajs.js
/**
* The Event interface represents any event which takes place in the DOM; some are user-generated
* (such as mouse or keyboard events), while others are generated by APIs (such as events that indicate
* an animation has finished running, a video has been paused, and so forth). There are many types of
* event, some of which use are other interfaces based on the main Event interface. Event itself contains
* the properties and methods which are common to all events.
* @see https://developer.mozilla.org/en-US/docs/Web/API/Event
* @author [email protected]
*/
@js.native
trait Event extends js.Object {
//////////////////////////////////////////////////////////////
// Constants
//////////////////////////////////////////////////////////////
/**
* The current event phase is the capture phase (1)
*/
val CAPTURING_PHASE: Int = js.native
/**
* The current event is in the target phase, i.e. it is being evaluated at the event target (2)
*/
val AT_TARGET: Int = js.native
/**
* The current event phase is the bubbling phase (3)
*/
val BUBBLING_PHASE: Int = js.native
//////////////////////////////////////////////////////////////
// Properties
//////////////////////////////////////////////////////////////
/**
* Returns whether or not a specific event is a bubbling event
*/
def bubbles: Boolean = js.native
/**
* Returns whether or not an event can have its default action prevented
*/
def cancelable: Boolean = js.native
/**
* Returns the element whose event listeners triggered the event
*/
def currentTarget: Element = js.native
/**
* Returns whether or not the preventDefault() method was called for the event
*/
def defaultPrevented: Boolean = js.native
/**
* Returns which phase of the event flow is currently being evaluated
*/
def eventPhase: String = js.native
/**
* Returns whether or not an event is trusted
*/
def isTrusted: Boolean = js.native
/**
* Returns the element that triggered the event
*/
def target: Element = js.native
/**
* Returns the time (in milliseconds relative to the epoch) at which the event was created
*/
def timeStamp: Double = js.native
/**
* Returns the name of the event
*/
def `type`: String = js.native
/**
* Returns a reference to the Window object where the event occurred
* TODO is this really a reference to Window?
*/
def view: js.Any /*Window*/ = js.native
//////////////////////////////////////////////////////////////
// Methods
//////////////////////////////////////////////////////////////
/**
* Cancels the event if it is cancelable, meaning that the default action that belongs to the event will not occur
*/
def preventDefault(): Unit = js.native
/**
* Prevents other listeners of the same event from being called
*/
def stopImmediatePropagation(): Unit = js.native
/**
* Prevents further propagation of an event during event flow
*/
def stopPropagation(): Unit = js.native
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy