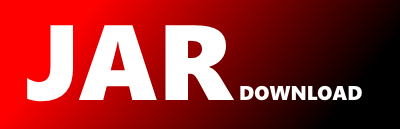
io.scalecube.config.AbstractSimpleConfigProperty Maven / Gradle / Ivy
package io.scalecube.config;
import io.scalecube.config.source.LoadedConfigProperty;
import java.util.Collections;
import java.util.Map;
import java.util.NoSuchElementException;
import java.util.Optional;
import java.util.concurrent.ConcurrentHashMap;
import java.util.function.Function;
/**
* Parent class for 'simple' property types, such as: double, int, string, list and etc.
*
* @param type of the property value
*/
class AbstractSimpleConfigProperty extends AbstractConfigProperty implements ConfigProperty {
/** Constructor for non-object config property. */
AbstractSimpleConfigProperty(
String name,
Class> propertyClass,
Map propertyMap,
Map> propertyCallbackMap,
Function valueParser) {
super(name, propertyClass);
// noinspection unchecked
setPropertyCallback(computePropertyCallback(valueParser, propertyCallbackMap));
LoadedConfigProperty configProperty = propertyMap.get(name);
computeValue(configProperty != null ? Collections.singletonList(configProperty) : null);
}
@Override
public final Optional source() {
return mapToString(list -> list.get(0).source().orElse(null));
}
@Override
public final Optional origin() {
return mapToString(list -> list.get(0).origin().orElse(null));
}
@Override
public final Optional valueAsString() {
return mapToString(list -> list.get(0).valueAsString().orElse(null));
}
@Override
public final String valueAsString(String defaultValue) {
return valueAsString().orElse(defaultValue);
}
final NoSuchElementException newNoSuchElementException() {
return new NoSuchElementException("Value is null for property '" + name + "'");
}
private PropertyCallback computePropertyCallback(
Function valueParser,
Map> propertyCallbackMap) {
PropertyCallback propertyCallback =
new PropertyCallback<>(list -> list.get(0).valueAsString().map(valueParser).orElse(null));
propertyCallbackMap.putIfAbsent(name, new ConcurrentHashMap<>());
Map callbackMap = propertyCallbackMap.get(name);
callbackMap.putIfAbsent(propertyClass, propertyCallback);
return callbackMap.get(propertyClass);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy