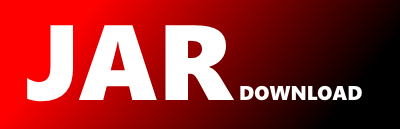
io.sealights.agents.plugin.EnhancedLogger Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sealights-maven-plugin
Show all versions of sealights-maven-plugin
A maven plugin to run java agents.
package io.sealights.agents.plugin;
import org.apache.maven.plugin.logging.Log;
import io.sealights.onpremise.agents.java.agent.infra.logging.ILogger;
import io.sealights.onpremise.agents.java.agent.infra.logging.LogFormatAdapter;
/**
* Created by Nadav on 06/11/2017.
*/
public class EnhancedLogger implements Log, ILogger {
private Log log;
final static String PLUGIN_TAG = "- [Sealights Maven Plugin] - ";
public EnhancedLogger(Log log){
this.log = log;
}
@Override
public boolean isDebugEnabled() {
return log.isDebugEnabled();
}
@Override
public void debug(CharSequence charSequence) {
log.debug(PLUGIN_TAG + charSequence);
}
@Override
public void debug(CharSequence charSequence, Throwable throwable) {
log.debug(PLUGIN_TAG + charSequence, throwable);
}
@Override
public void debug(Throwable throwable) {
log.debug(PLUGIN_TAG + throwable);
}
@Override
public boolean isInfoEnabled() {
return log.isInfoEnabled();
}
@Override
public void info(CharSequence charSequence) {
log.info(PLUGIN_TAG + charSequence);
}
@Override
public void info(CharSequence charSequence, Throwable throwable) {
log.info(PLUGIN_TAG + charSequence, throwable);
}
@Override
public void info(Throwable throwable) {
log.info(PLUGIN_TAG + throwable);
}
@Override
public boolean isWarnEnabled() {
return log.isWarnEnabled();
}
@Override
public void warn(CharSequence charSequence) {
log.warn(PLUGIN_TAG + charSequence);
}
@Override
public void warn(CharSequence charSequence, Throwable throwable) {
log.warn(PLUGIN_TAG + charSequence, throwable);
}
@Override
public void warn(Throwable throwable) {
log.warn(PLUGIN_TAG + throwable);
}
@Override
public boolean isErrorEnabled() {
return false;
}
@Override
public void error(CharSequence charSequence) {
log.error(PLUGIN_TAG + charSequence);
}
@Override
public void error(CharSequence charSequence, Throwable throwable) {
log.error(PLUGIN_TAG + charSequence, throwable);
}
@Override
public void error(Throwable throwable) {
log.error(PLUGIN_TAG + throwable);
}
// The methods below implement the bridge to the ILogger interface
// Format string will not work, since this is the logback format and not String format
@Override
public void trace(String string) {
debug(string);
}
@Override
public void trace(String format, Object arg) {
// Trace is not supported
debug(format, arg);
}
@Override
public void trace(String format, Object... args) {
// Trace is not supported
debug(format, args);
}
@Override
public void debug(String string) {
debug((CharSequence) string);
}
@Override
public void debug(String format, Object arg) {
debug(LogFormatAdapter.normalizedMessage(format, arg));
}
@Override
public void debug(String format, Object... args) {
debug(LogFormatAdapter.normalizedMessage(format, args));
}
@Override
public void info(String string) {
info((CharSequence) string);
}
@Override
public void info(String format, Object arg) {
info(LogFormatAdapter.normalizedMessage(format, arg));
}
@Override
public void info(String format, Object... args) {
info(LogFormatAdapter.normalizedMessage(format, args));
}
@Override
public void warning(String string) {
warn(string);
}
@Override
public void warning(String format, Object arg) {
warn(LogFormatAdapter.normalizedMessage(format, arg));
}
@Override
public void warning(String format, Object... args) {
warn(LogFormatAdapter.normalizedMessage(format, args));
}
@Override
public void error(String string) {
error((CharSequence) string);
}
@Override
public void error(String format, Object arg) {
error(LogFormatAdapter.normalizedMessage(format, arg));
}
@Override
public void error(String format, Object... args) {
error(LogFormatAdapter.normalizedMessage(format, args));
}
@Override
public void error(String string, Throwable e) {
error((CharSequence) string, e);
}
@Override
public void dispose() {
// Nothing so far
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy