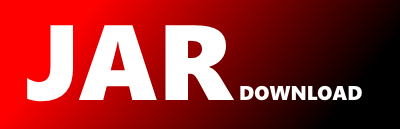
io.sealights.agents.plugin.SeaLightsMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sealights-maven-plugin
Show all versions of sealights-maven-plugin
A maven plugin to run java agents.
package io.sealights.agents.plugin;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import org.apache.maven.execution.MavenSession;
import org.apache.maven.model.Dependency;
import org.apache.maven.model.Plugin;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.project.MavenProject;
import io.sealights.onpremise.agents.java.agent.infra.logging.ILogger;
import io.sealights.onpremise.agents.java.agent.infra.utils.StringUtils;
import io.sealights.onpremise.agents.java.agent.infra.version.Version;
import io.sealights.onpremise.agents.java.agent.plugins.engine.api.DependencyInfo;
import io.sealights.onpremise.agents.java.agent.plugins.engine.api.ExecutionStage;
import io.sealights.onpremise.agents.java.agent.plugins.engine.api.PluginEngine;
import io.sealights.onpremise.agents.java.agent.plugins.engine.api.PluginExecData;
import io.sealights.onpremise.agents.java.agent.plugins.engine.api.PluginGoal;
import io.sealights.onpremise.agents.java.agent.plugins.engine.api.PluginParameters;
import io.sealights.onpremise.agents.java.agent.plugins.engine.api.PluginType;
import io.sealights.onpremise.agents.java.agent.plugins.engine.api.ProjectDescriptor;
import io.sealights.onpremise.agents.java.agent.plugins.engine.lifecycle.BuildEndInfo;
import io.sealights.onpremise.agents.java.agent.plugins.engine.lifecycle.BuildEndListener;
import io.sealights.onpremise.agents.java.agent.plugins.engine.lifecycle.BuildLifeCycle;
import io.sealights.onpremise.agents.java.agent.plugins.engine.procsexecutor.PluginEngineHandler;
import lombok.Getter;
import lombok.Setter;
/**
* An abstract Mojo for sealights mojos
*/
public abstract class SeaLightsMojo extends AbstractMojo implements PluginGoal, BuildEndListener {
public final static String SEALIGHTS_MAVEN_PLUGIN = "sealights-mvn-plugin";
public static final String LOGGER_TAG = "[Sealights Maven Plugin]";
public final static String SUREFIRE_ARG_LINE = "argLine";
public final static String SEALIGHTS_ARG_LINE = "sealightsArgLine";
private final static String MIN_SUREFIRE_VERSION_SUPPORTED = "2.9";
@Setter
@Getter
private PluginEngine pluginEngine = new PluginEngineHandler();
@Getter
private EnhancedLogger enhancedLog = new EnhancedLogger(getLog());
/**
* Maven project.
*
* @parameter property="project"
* @readonly
*/
@Setter
@Getter
private MavenProject project;
/**
* Define property which will be used to add sealights Test Listener.
* If not specified, 'argLine' will be set for surefire.
*
* @parameter property="argLineProperty"
*/
@Getter
private String argLineProperty;
/**
* The token provided by SeaLights.
*
* @parameter property="token"
*/
@Getter
private String token;
/**
* The token provided by SeaLights in file.
*
* @parameter property="tokenFile"
*/
@Getter
private String tokenFile;
/**
* Customer id.
*
* @parameter property="customerid"
*/
@Getter
private String customerid;
/**
* The url to sealights server.
*
* @parameter property="server"
*/
@Getter
private String server;
/**
* Url to proxy.
*
* @parameter property="proxy"
*/
@Getter
private String proxy;
/**
* Path to the source code root folder.
*
* @parameter property="workspacepath" default-value="${basedir}/target/classes"
*/
@Getter
private String workspacepath;
/**
* Use buildSessionId feature for this build.
*
* @parameter property="createBuildSessionId" default-value="false"
*/
@Getter
private boolean createBuildSessionId;
/**
* Use buildSessionId for build session data.
*
* @parameter property="buildSessionId"
*/
@Getter
private String buildSessionId;
/**
* Build session id from file.
*
* @parameter property="buildSessionId"
*/
@Getter
private String buildSessionIdFile;
/**
* The application name.
*
* @parameter property="appName"
*/
@Getter
private String appName;
/**
* The module name.
*
* @parameter property="moduleName"
*/
@Getter
private String moduleName;
/**
* The development environment.
*
* @parameter property="environment"
*/
@Getter
private String environment;
/**
* The branch name.
*
* @parameter property="branch"
*/
@Getter
private String branch;
/**
* The build version.
*
* @parameter property="build" default-value="${version}"
*/
@Getter
private String build;
/**
* Comma-separated list of packages to scan.
* Supports wildcards (* = any string, ? = any character).
* For example:'com.example.* ,io.*.demo, com.?ello.world'.
*
* @parameter property="packagesincluded"
*/
@Getter
private String packagesincluded;
/**
* Comma-separated list of packages to exclude from scan.
* Supports wildcards (* = any string, ? = any character).
* For example: 'com.example.* ,io.*.demo, com.?ello.world'.
*
* @parameter property="packagesexcluded"
*/
@Getter
private String packagesexcluded;
/**
* Comma-separated list of files to scan.
* Supports wildcards (* = any string, ? = any character).
* For example: 'original-*.jar ,demo?.jar'.
*
* @parameter property="filesincluded" default-value="*.class"
*/
@Getter
private String filesincluded;
/**
* Comma-separated list of files to exclude from scan.
* Supports wildcards (* = any string, ? = any character).
* For example: '*-with-dependencies.jar , bad-bad?.war, *-source.jar'.
*
* @parameter property="filesexcluded" default-value="*test-classes*"
*/
@Getter
private String filesexcluded;
/**
* Flag to start a recursive search for files to scan in the folder specified by the 'workspacepath' option.
*
* @parameter property="recursive" default-value="true"
*/
@Getter
private boolean recursive;
/**
* Flag to enable logs.
*
* @parameter property="logEnabled" default-value="false"
*/
@Getter
private boolean logEnabled;
/**
* Log level (e.g info, debug..)
*
* @parameter property="logLevel" default-value="info"
*/
@Getter
private String logLevel;
/**
* Flag to write logs to file.
*
* @parameter property="logToFile" default-value="false"
*/
@Getter
private boolean logToFile;
/**
* Flag to write logs to file.
*
* @parameter property="logToConsole" default-value="false"
*/
@Getter
private boolean logToConsole;
/**
* Path to folder where log file will be written.
*
* @parameter property="logFolder"
*/
@Getter
private String logFolder;
/**
* Path to specific java version.
*
* @parameter property="javaPath" default-value="java"
*/
@Getter
private String javaPath;
/**
* Path to a folder where Sealights can save files.
*
* @parameter property="filesStorage"
*/
@Getter
private String filesStorage;
// /**
// * Meta data of the build currently executed.
// *
// * @parameter property="metadata"
// */
// private Map metadata;
/**
* Jvm params for testListener.
*
* @parameter property="sealightsJvmParams"
*
* */
@Getter
private Map sealightsJvmParams;
/**
* Force this plugin to create the test listener in this path.
*
* @parameter property="overrideMetaJsonPath"
*/
@Getter
private String overrideMetaJsonPath;
/**
* Include token and buildSessionId files in jar.
*
* @parameter property="includeResources" default-value="false"
*/
@Getter
private boolean includeResources;
/**
* Path to the sealights Build Scanner.
*
* @parameter property="buildScannerJar"
*/
@Getter
public String buildScannerJar;
/**
* Path to the sealights Test Listener.
*
* @parameter property="testListenerJar"
*/
@Getter
public String testListenerJar;
/**
* The Maven Session Object
*
* @parameter property="session"
* @required
* @readonly
*/
@Getter
private MavenSession session;
/*
* The maven project properties
*
* */
@Setter
@Getter
private Properties projectProperties;
private String sealightsPluginVersion;
@Override
public PluginType getPluginType() {
return PluginType.maven;
}
@Override
public void execute() throws MojoExecutionException, MojoFailureException {
initSealightsArgLine();
try {
if (getExecData().getExecStage() != ExecutionStage.initFailed) {
executePluginGoal();
}
else {
enhancedLog.info(PluginExecData.SKIP_SEALIGHTS_MSG);
}
}
catch(Exception e) {
enhancedLog.info("Failed to execute goal '{}'; proceed build", getName());
}
}
@Override
public void onSuccess(PluginExecData execData) {
}
@Override
public void onFailure() {
}
@Override
public boolean initGoalInternals() {
projectProperties = project.getProperties();
verifySurefireSet();
setModuleNameIfNull();
if (StringUtils.isNullOrEmpty(filesStorage)) {
filesStorage = System.getProperty("java.io.tmpdir");
}
return MavenEventsListener.INSTANCE.initialize(session, this);
}
@Override
public ProjectDescriptor getProjectDecriptor() {
List pluginsDeps = buildProjectPluginsData();
List projectDeps = buildProjectDependencyData();
return new ProjectDescriptor(
SEALIGHTS_MAVEN_PLUGIN,
sealightsPluginVersion,
project.getName(),
project.getVersion(),
projectProperties,
project.getBuild().getSourceDirectory(),
project.getBasedir() != null ? project.getBasedir().getAbsolutePath() : null,
project.getBuild().getOutputDirectory(),
projectDeps,
pluginsDeps);
}
@Override
public PluginExecData getExecData() {
return BuildLifeCycle.INSTANCE.getExecData();
}
@Override
public ILogger getLogger() {
return getEnhancedLog();
}
/*
* Implementation of BuildEndListener interface
*/
@Override
public void notifyBuildEndInfo(BuildEndInfo buildEndInfo) {
pluginEngine.notifyBuildEnd(this, buildEndInfo);
}
@Override
public ILogger getPluginLogger() {
return getLogger();
}
protected List buildProjectDependencyData(){
List dependenciesData = new ArrayList<>();
for (Dependency d: project.getDependencies()){
dependenciesData.add(new DependencyInfo(d.getGroupId(), d.getArtifactId(), d.getVersion()));
}
return dependenciesData;
}
protected List buildProjectPluginsData() {
List pluginsData = new ArrayList<>();
for (Plugin p: project.getBuildPlugins()) {
pluginsData.add(new DependencyInfo(p.getGroupId(), p.getArtifactId(), p.getVersion()));
if (p.getArtifactId().contains("sealights")) {
sealightsPluginVersion = p.getVersion();
}
}
return pluginsData;
}
protected PluginParameters getGenParams() {
PluginParameters params = new PluginParameters();
params.getGeneralParams().setToken(token);
params.getGeneralParams().setTokenFile(tokenFile);
params.getGeneralParams().setCustomerid(customerid);
params.getGeneralParams().setServer(server);
params.getGeneralParams().setProxy(proxy);
params.getGeneralParams().setWorkspacepath(workspacepath);
params.getGeneralParams().setCreateBuildSessionId(createBuildSessionId);
params.getGeneralParams().setBuildSessionId(buildSessionId);
params.getGeneralParams().setBuildSessionIdFile(buildSessionIdFile);
params.getGeneralParams().setAppName(appName);
params.getGeneralParams().setModuleName(moduleName);
params.getGeneralParams().setEnvironment(environment);
params.getGeneralParams().setBranch(branch);
params.getGeneralParams().setBuild(build);
params.getGeneralParams().setPackagesincluded(packagesincluded);
params.getGeneralParams().setPackagesexcluded(packagesexcluded);
params.getGeneralParams().setFilesincluded(filesincluded);
params.getGeneralParams().setFilesexcluded(filesexcluded);
params.getGeneralParams().setRecursive(recursive);
params.getGeneralParams().setLogEnabled(logEnabled);
params.getGeneralParams().setLogLevel(logLevel);
params.getGeneralParams().setLogToFile(logToFile);
params.getGeneralParams().setLogToConsole(logToConsole);
params.getGeneralParams().setLogFolder(logFolder);
params.getGeneralParams().setJavaPath(javaPath);
params.getGeneralParams().setFilesStorage(filesStorage);
params.getGeneralParams().setSealightsJvmParams(sealightsJvmParams);
params.getGeneralParams().setOverrideMetaJsonPath(overrideMetaJsonPath);
params.getGeneralParams().setIncludeResources(includeResources);
params.getGeneralParams().setBuildScannerJar(buildScannerJar);
params.getGeneralParams().setTestListenerJar(testListenerJar);
return params;
}
protected String getArgLinePropertyName() {
if (StringUtils.isNullOrEmpty(argLineProperty)) {
return SUREFIRE_ARG_LINE;
}
return argLineProperty;
}
private void setModuleNameIfNull(){
if (StringUtils.isNullOrEmpty(moduleName)){
moduleName = "__root-module__" + System.currentTimeMillis();
}
}
private void verifySurefireSet() {
for (Plugin plugin : project.getBuildPlugins()) {
if ("maven-surefire-plugin".equals(plugin.getArtifactId())) {
String surefireVersion = plugin.getVersion();
if (!isSureFireVersionSupported(surefireVersion, MIN_SUREFIRE_VERSION_SUPPORTED))
enhancedLog.warn("[SeaLights] - surefire plugin version is not supported. please use version "
+ MIN_SUREFIRE_VERSION_SUPPORTED + " or later.");
return;
}
}
enhancedLog.warn("[SeaLights] - surefire plugin is not set, SeaLights Test Listener will not run.");
}
private boolean isSureFireVersionSupported(String actual, String minExpected) {
Version actualVersion = new Version(actual);
Version minExpectedVersion = new Version(minExpected);
return minExpectedVersion.compareTo(actualVersion) != 1;
}
abstract protected boolean executePluginGoal();
/**
Sets the default value of sealightsArgline property to prevent surefire failure.
This happens, if surefire argLine contains sealightsArgline property and sealights is disabled.
then argLine contains '@{sealightsArgLine}' which equals to null and tests wont run.
*/
private void initSealightsArgLine() {
if (project != null && project.getProperties().getProperty(SEALIGHTS_ARG_LINE) == null){
project.getProperties().setProperty(SEALIGHTS_ARG_LINE, "");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy