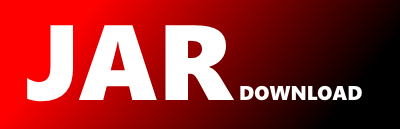
io.sealights.agents.plugin.TstListnrMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sealights-maven-plugin
Show all versions of sealights-maven-plugin
A maven plugin to run java agents.
package io.sealights.agents.plugin;
import static io.sealights.onpremise.agents.java.agent.infra.constants.SLProperties.toJvmAgr;
import java.util.Map;
import org.apache.maven.plugins.annotations.Mojo;
import io.sealights.onpremise.agents.java.agent.infra.constants.Constants;
import io.sealights.onpremise.agents.java.agent.infra.constants.SLProperties;
import io.sealights.onpremise.agents.java.agent.infra.utils.StringUtils;
import io.sealights.onpremise.agents.java.agent.plugins.engine.api.PluginExecData;
import io.sealights.onpremise.agents.java.agent.plugins.engine.api.PluginParameters;
import io.sealights.onpremise.agents.java.agent.plugins.engine.clibuilders.TokenValueFormatter;
/**
* Runs the Test Listener.
*
* @phase generate-sources
* @goal test-listener
* @threadSafe
* @since 1.0.0
*/
@Mojo(name = "listener")
public class TstListnrMojo extends SeaLightsMojo {
/**
* Flag to enable/disable Test Listener.
*
* @parameter property="testListenerEnabled" default-value="true"
*/
private boolean testListenerEnabled;
/**
* Path to the Test Listener configuration file.
*
* @parameter property="testListenerConfigFile"
*/
private String testListenerConfigFile;
/**
* Jvm params for testListener.
*
* @parameter property="testListenerJvmParams"
*
* */
private Map testListenerJvmParams;
/**
* Initial color for the Test Listener.
*
* @parameter property="initialColor"
*/
private String initialColor;
/**
* Class loaders to be excluded.
*
* @parameter property="classLoadersExcluded"
*/
private String classLoadersExcluded;
/**
* The testStage.
*
* @parameter property="testStage"
*/
private String testStage;
/**
* The labId.
*
* @parameter property="labId"
*/
private String labId;
/**
* Force this plugin to create the test listener in this path.
*
* @parameter property="overrideTestListenerPath"
*/
private String overrideTestListenerPath;
/**
* Flag to disable test listener logs in spite of common logEnabled=true
*
* @parameter property="testListenerIgnoreLogEnabled" default-value="false"
*/
private boolean testListenerIgnoreLogEnabled;
@Override
public PluginParameters getParams() {
PluginParameters params = getGenParams();
params.getTstListnrParams().setOverrideTestListenerPath(overrideTestListenerPath);
params.getTstListnrParams().setTestListenerEnabled(testListenerEnabled);
params.getTstListnrParams().setTestListenerConfigFile(testListenerConfigFile);
params.getTstListnrParams().setTestListenerJvmParams(testListenerJvmParams);
params.getTstListnrParams().setInitialColor(initialColor);
params.getTstListnrParams().setClassLoadersExcluded(classLoadersExcluded);
params.getTstListnrParams().setTestStage(testStage);
params.getTstListnrParams().setLabId(labId);
params.getTstListnrParams().setTestListenerIgnoreLogEnabled(testListenerIgnoreLogEnabled);
return params;
}
@Override
protected boolean executePluginGoal() {
return getPluginEngine().executeTstLisnrGoal(this).isOk();
}
@Override
public void onSuccess(PluginExecData execData) {
super.onSuccess(execData);
setTstLsnrPathSysProperty();
setMavenPropertiesForNewVM(getExecData().getTstLisnrCliData().getMavenPluginTstLisnrCli());
}
@Override
public void onFailure() {
// In the jenkins plugin,
// if the 'configuration.argLine' element inside surefire plugin is present,
// we will add the ${argLine} variable.
// In case sealights is disabled, we need to make sure this variable is not null so maven won't fail.
setMavenPropertiesForNewVM(toJvmAgr(SLProperties.ENABLED, Constants.FALSE));
}
@Override
public String getName() {
return "test listener";
}
private void setMavenPropertiesForNewVM(String slArgument) {
String argLineVarPropertyName = getArgLinePropertyName();
String argLineVarValue = getProjectProperties().getProperty(argLineVarPropertyName);
getEnhancedLog().info("'{}' original value:'{}'", argLineVarPropertyName, argLineVarValue);
argLineVarValue = argLineVarValue != null ? argLineVarValue : "";
String newArgLineVariable = createNewArgLine(argLineVarValue, slArgument);
informNewArgLineVar(newArgLineVariable);
getProjectProperties().setProperty(argLineVarPropertyName, newArgLineVariable);
getProjectProperties().setProperty(SEALIGHTS_ARG_LINE, slArgument);
}
private String createNewArgLine(String argLine, String slArgument) {
SurefireManager surefireManager = new SurefireManager();
String unduplicatedArgline = surefireManager.preventArgLineDuplication(argLine);
warnForOtherJavaAgents(unduplicatedArgline);
StringBuilder newArgLine = new StringBuilder(slArgument);
if (!StringUtils.isNullOrEmpty(unduplicatedArgline)) {
newArgLine.append(" ");
newArgLine.append(unduplicatedArgline);
}
return newArgLine.toString();
}
private void warnForOtherJavaAgents(String argLineVariable) {
if (argLineVariable != null && argLineVariable.contains(" -javaagent:")) {
getEnhancedLog().warn("---------------------------------------------");
getEnhancedLog().warn("Another java agent is running with SeaLights.");
getEnhancedLog().warn("This may interfere the Test Listener process and result in low coverage.");
getEnhancedLog().warn("---------------------------------------------");
}
}
private void informNewArgLineVar(String newArgLineVariable) {
getEnhancedLog().info("---------------------------------------------");
getEnhancedLog().info("new ${argLine}: ");
getEnhancedLog().info(TokenValueFormatter.truncateTokenSysProperty(newArgLineVariable, getExecData().getToken()));
getEnhancedLog().info("---------------------------------------------");
}
private void setTstLsnrPathSysProperty(){
if(getProject() != null && getProject().getProperties() != null) {
getProject().getProperties().setProperty(SLProperties.PATH_TO_TEST_LISTENER, getExecData().getTstLisnrLocation());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy