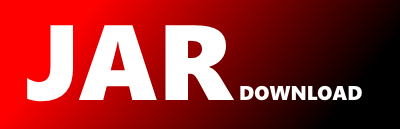
io.sealights.onpremise.agents.plugin.logging.MavenLogWrapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sealights-maven-plugin
Show all versions of sealights-maven-plugin
A maven plugin to run java agents.
The newest version!
package io.sealights.onpremise.agents.plugin.logging;
import io.sealights.onpremise.agents.infra.logging.Level;
import io.sealights.onpremise.agents.infra.logging.LogFormatAdapter;
import org.apache.maven.plugin.logging.Log;
import org.slf4j.Logger;
import org.slf4j.helpers.MarkerIgnoringBase;
/**
* Created by Nadav on 06/11/2017.
*/
public class MavenLogWrapper extends MarkerIgnoringBase implements Logger {
static final String PLUGIN_TAG = "- [Sealights Maven Plugin] - ";
private final Log log;
private final boolean enabled;
public MavenLogWrapper(Log log, boolean enabled) {
this.log = log;
this.enabled = enabled;
}
@Override
public boolean isTraceEnabled() {
return enabled;
}
@Override
public boolean isDebugEnabled() {
return enabled;
}
@Override
public boolean isInfoEnabled() {
return enabled;
}
@Override
public boolean isWarnEnabled() {
return enabled;
}
@Override
public boolean isErrorEnabled() {
return enabled;
}
// The methods below implement the bridge to the ILogger and SLF4J interfaces
// Format string will not work, since this is the logback format and not String format
@Override
public void trace(String string) {
delegate(Level.TRACE, string);
}
@Override
public void trace(String format, Object arg) {
delegate(Level.TRACE, LogFormatAdapter.normalizedMessage(format, arg));
}
@Override
public void trace(String format, Object... args) {
delegate(Level.TRACE, LogFormatAdapter.normalizedMessage(format, args));
}
@Override
public void debug(String string) {
delegate(Level.DEBUG, string);
}
@Override
public void debug(String format, Object arg) {
delegate(Level.DEBUG, LogFormatAdapter.normalizedMessage(format, arg));
}
@Override
public void debug(String format, Object... args) {
delegate(Level.DEBUG, LogFormatAdapter.normalizedMessage(format, args));
}
@Override
public void info(String string) {
delegate(Level.INFO, string);
}
@Override
public void info(String format, Object arg) {
delegate(Level.INFO, LogFormatAdapter.normalizedMessage(format, arg));
}
@Override
public void info(String format, Object... args) {
delegate(Level.INFO, LogFormatAdapter.normalizedMessage(format, args));
}
@Override
public void warn(String string) {
delegate(Level.WARN, string);
}
@Override
public void warn(String format, Object arg) {
delegate(Level.WARN, LogFormatAdapter.normalizedMessage(format, arg));
}
@Override
public void warn(String format, Object... args) {
delegate(Level.WARN, LogFormatAdapter.normalizedMessage(format, args));
}
@Override
public void error(String string) {
delegate(Level.ERROR, string);
}
@Override
public void error(String format, Object arg) {
delegate(Level.ERROR, LogFormatAdapter.normalizedMessage(format, arg));
}
@Override
public void error(String format, Object... args) {
delegate(Level.ERROR, LogFormatAdapter.normalizedMessage(format, args));
}
@Override
public void error(String string, Throwable e) {
delegate(Level.ERROR, string, e);
}
// Below are implementations of abstract methods from MarkerIgnoringBase which implements the SLF4J Logger interface.
@Override
public void warn(String format, Object arg1, Object arg2) {
delegate(Level.WARN, LogFormatAdapter.normalizedMessage(format, arg1, arg2));
}
@Override
public void warn(String msg, Throwable t) {
delegate(Level.WARN, msg, t);
}
@Override
public void trace(String format, Object arg1, Object arg2) {
delegate(Level.TRACE, LogFormatAdapter.normalizedMessage(format, arg1, arg2));
}
@Override
public void trace(String msg, Throwable t) {
delegate(Level.TRACE, msg, t);
}
@Override
public void debug(String format, Object arg1, Object arg2) {
delegate(Level.DEBUG, LogFormatAdapter.normalizedMessage(format, arg1, arg2));
}
@Override
public void debug(String msg, Throwable t) {
delegate(Level.DEBUG, msg, t);
}
@Override
public void info(String format, Object arg1, Object arg2) {
delegate(Level.INFO, LogFormatAdapter.normalizedMessage(format, arg1, arg2));
}
@Override
public void info(String msg, Throwable t) {
delegate(Level.INFO, msg, t);
}
@Override
public void error(String format, Object arg1, Object arg2) {
delegate(Level.ERROR, LogFormatAdapter.normalizedMessage(format, arg1, arg2));
}
// Below methods are delegating calls to the Maven Log instance.
private void delegate(Level logLineLevel, CharSequence charSequence) {
delegate(logLineLevel, charSequence, null);
}
private void delegate(Level logLineLevel, CharSequence charSequence, Throwable throwable) {
if (enabled) {
toMavenLog(logLineLevel, charSequence, throwable);
}
}
private void toMavenLog(Level logLineLevel, CharSequence charSequence, Throwable throwable) {
switch (logLineLevel) {
case TRACE:
// Maven Log doesn't support trace, delegating to log.debug(...)
case DEBUG:
if (charSequence == null) {
log.debug(PLUGIN_TAG, throwable);
} else if (throwable == null) {
log.debug(PLUGIN_TAG + charSequence);
} else {
log.debug(PLUGIN_TAG + charSequence, throwable);
}
break;
case INFO:
if (charSequence == null) {
log.info(PLUGIN_TAG, throwable);
} else if (throwable == null) {
log.info(PLUGIN_TAG + charSequence);
} else {
log.info(PLUGIN_TAG + charSequence, throwable);
}
break;
case WARN:
if (charSequence == null) {
log.warn(PLUGIN_TAG, throwable);
} else if (throwable == null) {
log.warn(PLUGIN_TAG + charSequence);
} else {
log.warn(PLUGIN_TAG + charSequence, throwable);
}
break;
case ERROR:
if (charSequence == null) {
log.error(PLUGIN_TAG, throwable);
} else if (throwable == null) {
log.error(PLUGIN_TAG + charSequence);
} else {
log.error(PLUGIN_TAG + charSequence, throwable);
}
break;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy