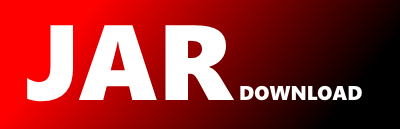
io.searchbox.core.Bulk Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jest-common Show documentation
Show all versions of jest-common Show documentation
ElasticSearch Java REST client - shared library package
package io.searchbox.core;
import com.google.common.reflect.TypeToken;
import com.google.gson.Gson;
import com.google.gson.JsonObject;
import io.searchbox.action.AbstractAction;
import io.searchbox.action.BulkableAction;
import io.searchbox.action.GenericResultAbstractAction;
import io.searchbox.params.Parameters;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.*;
/**
* The bulk API makes it possible to perform many index/delete operations in a
* single API call. This can greatly increase the indexing speed.
*
*
* Make sure that your source data (provided in Action instances) does NOT
* have unescaped line-breaks (e.g.: "\n"
or "\r\n"
)
* as doing so will break up the elasticsearch's bulk api format and bulk operation
* will fail.
*
* @author Dogukan Sonmez
* @author cihat keser
*/
public class Bulk extends AbstractAction {
final static Logger log = LoggerFactory.getLogger(Bulk.class);
protected Collection bulkableActions;
protected Bulk(Builder builder) {
super(builder);
indexName = builder.defaultIndex;
typeName = builder.defaultType;
bulkableActions = builder.actions;
setURI(buildURI());
}
private Object getJson(Gson gson, Object source) {
if (source instanceof String) {
return source;
} else {
return gson.toJson(source);
}
}
@Override
public String getRestMethodName() {
return "POST";
}
@Override
public String getData(Gson gson) {
/*
{ "index" : { "_index" : "test", "_type" : "type1", "_id" : "1" } }
{ "field1" : "value1" }
{ "delete" : { "_index" : "test", "_type" : "type1", "_id" : "2" } }
*/
StringBuilder sb = new StringBuilder();
for (BulkableAction action : bulkableActions) {
// write out the action-meta-data line
// e.g.: { "index" : { "_index" : "test", "_type" : "type1", "_id" : "1" } }
Map> opMap = new LinkedHashMap>(1);
Map opDetails = new LinkedHashMap(3);
if (StringUtils.isNotBlank(action.getId())) {
opDetails.put("_id", action.getId());
}
if (StringUtils.isNotBlank(action.getIndex())) {
opDetails.put("_index", action.getIndex());
}
if (StringUtils.isNotBlank(action.getType())) {
opDetails.put("_type", action.getType());
}
for (String parameter : Parameters.ACCEPTED_IN_BULK) {
try {
Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy