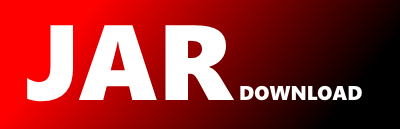
io.sentry.util.CollectionUtils Maven / Gradle / Ivy
package io.sentry.util;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import org.jetbrains.annotations.ApiStatus;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
/** Util class for Collections */
@ApiStatus.Internal
public final class CollectionUtils {
private CollectionUtils() {}
/**
* Returns an Iterator size
*
* @param data the Iterable
* @return iterator size
*/
public static int size(final @NotNull Iterable> data) {
if (data instanceof Collection) {
return ((Collection>) data).size();
}
int counter = 0;
for (Object ignored : data) {
counter++;
}
return counter;
}
/**
* Creates a new {@link ConcurrentHashMap} as a shallow copy of map given by parameter. Also makes
* sure no null keys or values are put into the resulting {@link ConcurrentHashMap}.
*
* @param map the map to copy
* @param the type of map keys
* @param the type of map values
* @return the shallow copy of map
*/
public static @Nullable Map newConcurrentHashMap(
@Nullable Map map) {
if (map != null) {
Map concurrentMap = new ConcurrentHashMap<>();
for (Map.Entry entry : map.entrySet()) {
if (entry.getKey() != null && entry.getValue() != null) {
concurrentMap.put(entry.getKey(), entry.getValue());
}
}
return concurrentMap;
} else {
return null;
}
}
/**
* Creates a new {@link HashMap} as a shallow copy of map given by parameter.
*
* @param map the map to copy
* @param the type of map keys
* @param the type of map values
* @return a new {@link HashMap} or {@code null} if parameter is {@code null}
*/
public static @Nullable Map newHashMap(@Nullable Map map) {
if (map != null) {
return new HashMap<>(map);
} else {
return null;
}
}
/**
* Creates a new {@link ArrayList} as a shallow copy of list given by parameter.
*
* @param list the list to copy
* @param the type of list entries
* @return a new {@link ArrayList} or {@code null} if parameter is {@code null}
*/
public static @Nullable List newArrayList(@Nullable List list) {
if (list != null) {
return new ArrayList<>(list);
} else {
return null;
}
}
/**
* Returns a new map which entries match a predicate specified by a parameter.
*
* @param map - the map to filter
* @param predicate - the predicate
* @param - map entry key type
* @param - map entry value type
* @return a new map
*/
public static @NotNull Map filterMapEntries(
final @NotNull Map map, final @NotNull Predicate> predicate) {
final Map filteredMap = new HashMap<>();
for (final Map.Entry entry : map.entrySet()) {
if (predicate.test(entry)) {
filteredMap.put(entry.getKey(), entry.getValue());
}
}
return filteredMap;
}
/**
* Returns a new list with the results of the function applied to all elements of the original
* list.
*
* @param list - the list to apply the function to
* @param f - the function
* @param - original list element type
* @param - returned list element type
* @return a new list
*/
public static @NotNull List map(
final @NotNull List list, final @NotNull Mapper f) {
List mappedList = new ArrayList<>(list.size());
for (T t : list) {
mappedList.add(f.map(t));
}
return mappedList;
}
/**
* Returns a new list which entries match a predicate specified by a parameter.
*
* @param predicate - the predicate
* @return a new list
*/
public static @NotNull List filterListEntries(
final @NotNull List list, final @NotNull Predicate predicate) {
final List filteredList = new ArrayList<>(list.size());
for (final T entry : list) {
if (predicate.test(entry)) {
filteredList.add(entry);
}
}
return filteredList;
}
/**
* Returns true if the element is present in the array, false otherwise.
*
* @param array - the array
* @param element - the element
* @return true if the element is present in the array, false otherwise.
*/
public static boolean contains(final @NotNull T[] array, final @NotNull T element) {
for (final T t : array) {
if (element.equals(t)) {
return true;
}
}
return false;
}
/**
* A simplified copy of Java 8 Predicate.
*
* @param the type
*/
public interface Predicate {
boolean test(T t);
}
/**
* A simple function to map an object into another.
*
* @param the original type
* @param the returned type
*/
public interface Mapper {
R map(T t);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy