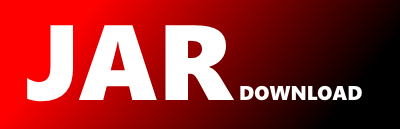
io.serverlessworkflow.api.schedule.Schedule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of serverlessworkflow-api Show documentation
Show all versions of serverlessworkflow-api Show documentation
Java SDK for Serverless Workflow Specification
package io.serverlessworkflow.api.schedule;
import java.io.Serializable;
import java.util.HashMap;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonValue;
/**
* Start state schedule definition
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"interval",
"cron",
"directInvoke",
"timezone"
})
public class Schedule implements Serializable
{
/**
* Time interval (ISO 8601 format) describing when the workflow starting state is active
*
*/
@JsonProperty("interval")
@JsonPropertyDescription("Time interval (ISO 8601 format) describing when the workflow starting state is active")
private String interval;
/**
* Repeating interval (cron expression) describing when the workflow starting state should be triggered
*
*/
@JsonProperty("cron")
@JsonPropertyDescription("Repeating interval (cron expression) describing when the workflow starting state should be triggered")
private String cron;
/**
* Define if workflow instances can be created outside of the defined interval/cron
*
*/
@JsonProperty("directInvoke")
@JsonPropertyDescription("Define if workflow instances can be created outside of the defined interval/cron")
private Schedule.DirectInvoke directInvoke;
/**
* Timezone name used to evaluate the cron expression. Not used for interval as timezone can be specified there directly. If not specified, should default to local machine timezone.
*
*/
@JsonProperty("timezone")
@JsonPropertyDescription("Timezone name used to evaluate the cron expression. Not used for interval as timezone can be specified there directly. If not specified, should default to local machine timezone.")
private String timezone;
private final static long serialVersionUID = -4388453232374848054L;
/**
* Time interval (ISO 8601 format) describing when the workflow starting state is active
*
*/
@JsonProperty("interval")
public String getInterval() {
return interval;
}
/**
* Time interval (ISO 8601 format) describing when the workflow starting state is active
*
*/
@JsonProperty("interval")
public void setInterval(String interval) {
this.interval = interval;
}
public Schedule withInterval(String interval) {
this.interval = interval;
return this;
}
/**
* Repeating interval (cron expression) describing when the workflow starting state should be triggered
*
*/
@JsonProperty("cron")
public String getCron() {
return cron;
}
/**
* Repeating interval (cron expression) describing when the workflow starting state should be triggered
*
*/
@JsonProperty("cron")
public void setCron(String cron) {
this.cron = cron;
}
public Schedule withCron(String cron) {
this.cron = cron;
return this;
}
/**
* Define if workflow instances can be created outside of the defined interval/cron
*
*/
@JsonProperty("directInvoke")
public Schedule.DirectInvoke getDirectInvoke() {
return directInvoke;
}
/**
* Define if workflow instances can be created outside of the defined interval/cron
*
*/
@JsonProperty("directInvoke")
public void setDirectInvoke(Schedule.DirectInvoke directInvoke) {
this.directInvoke = directInvoke;
}
public Schedule withDirectInvoke(Schedule.DirectInvoke directInvoke) {
this.directInvoke = directInvoke;
return this;
}
/**
* Timezone name used to evaluate the cron expression. Not used for interval as timezone can be specified there directly. If not specified, should default to local machine timezone.
*
*/
@JsonProperty("timezone")
public String getTimezone() {
return timezone;
}
/**
* Timezone name used to evaluate the cron expression. Not used for interval as timezone can be specified there directly. If not specified, should default to local machine timezone.
*
*/
@JsonProperty("timezone")
public void setTimezone(String timezone) {
this.timezone = timezone;
}
public Schedule withTimezone(String timezone) {
this.timezone = timezone;
return this;
}
public enum DirectInvoke {
ALLOW("allow"),
DENY("deny");
private final String value;
private final static Map CONSTANTS = new HashMap();
static {
for (Schedule.DirectInvoke c: values()) {
CONSTANTS.put(c.value, c);
}
}
private DirectInvoke(String value) {
this.value = value;
}
@Override
public String toString() {
return this.value;
}
@JsonValue
public String value() {
return this.value;
}
@JsonCreator
public static Schedule.DirectInvoke fromValue(String value) {
Schedule.DirectInvoke constant = CONSTANTS.get(value);
if (constant == null) {
throw new IllegalArgumentException(value);
} else {
return constant;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy