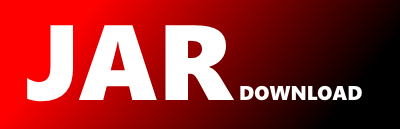
io.serverlessworkflow.api.states.CallbackState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of serverlessworkflow-api Show documentation
Show all versions of serverlessworkflow-api Show documentation
Java SDK for Serverless Workflow Specification
package io.serverlessworkflow.api.states;
import java.io.Serializable;
import java.util.List;
import java.util.Map;
import javax.validation.Valid;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import io.serverlessworkflow.api.actions.Action;
import io.serverlessworkflow.api.end.End;
import io.serverlessworkflow.api.error.Error;
import io.serverlessworkflow.api.filters.EventDataFilter;
import io.serverlessworkflow.api.filters.StateDataFilter;
import io.serverlessworkflow.api.interfaces.State;
import io.serverlessworkflow.api.start.Start;
import io.serverlessworkflow.api.transitions.Transition;
/**
* This state is used to wait for events from event sources and then transitioning to a next state
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"action",
"eventRef",
"timeout",
"eventDataFilter"
})
public class CallbackState
extends DefaultState
implements Serializable, State
{
/**
* Action Definition
*
*/
@JsonProperty("action")
@JsonPropertyDescription("Action Definition")
@Valid
private Action action;
/**
* References an unique callback event name in the defined workflow events
*
*/
@JsonProperty("eventRef")
@JsonPropertyDescription("References an unique callback event name in the defined workflow events")
private java.lang.String eventRef;
/**
* Time period to wait for incoming events (ISO 8601 format)
*
*/
@JsonProperty("timeout")
@JsonPropertyDescription("Time period to wait for incoming events (ISO 8601 format)")
private java.lang.String timeout;
@JsonProperty("eventDataFilter")
@Valid
private EventDataFilter eventDataFilter;
private final static long serialVersionUID = 3299874634212528933L;
/**
* No args constructor for use in serialization
*
*/
public CallbackState() {
}
/**
*
* @param name
* @param type
*/
public CallbackState(java.lang.String name, DefaultState.Type type) {
super(name, type);
}
/**
* Action Definition
*
*/
@JsonProperty("action")
public Action getAction() {
return action;
}
/**
* Action Definition
*
*/
@JsonProperty("action")
public void setAction(Action action) {
this.action = action;
}
public CallbackState withAction(Action action) {
this.action = action;
return this;
}
/**
* References an unique callback event name in the defined workflow events
*
*/
@JsonProperty("eventRef")
public java.lang.String getEventRef() {
return eventRef;
}
/**
* References an unique callback event name in the defined workflow events
*
*/
@JsonProperty("eventRef")
public void setEventRef(java.lang.String eventRef) {
this.eventRef = eventRef;
}
public CallbackState withEventRef(java.lang.String eventRef) {
this.eventRef = eventRef;
return this;
}
/**
* Time period to wait for incoming events (ISO 8601 format)
*
*/
@JsonProperty("timeout")
public java.lang.String getTimeout() {
return timeout;
}
/**
* Time period to wait for incoming events (ISO 8601 format)
*
*/
@JsonProperty("timeout")
public void setTimeout(java.lang.String timeout) {
this.timeout = timeout;
}
public CallbackState withTimeout(java.lang.String timeout) {
this.timeout = timeout;
return this;
}
@JsonProperty("eventDataFilter")
public EventDataFilter getEventDataFilter() {
return eventDataFilter;
}
@JsonProperty("eventDataFilter")
public void setEventDataFilter(EventDataFilter eventDataFilter) {
this.eventDataFilter = eventDataFilter;
}
public CallbackState withEventDataFilter(EventDataFilter eventDataFilter) {
this.eventDataFilter = eventDataFilter;
return this;
}
@Override
public CallbackState withId(java.lang.String id) {
super.withId(id);
return this;
}
@Override
public CallbackState withName(java.lang.String name) {
super.withName(name);
return this;
}
@Override
public CallbackState withType(DefaultState.Type type) {
super.withType(type);
return this;
}
@Override
public CallbackState withStart(Start start) {
super.withStart(start);
return this;
}
@Override
public CallbackState withEnd(End end) {
super.withEnd(end);
return this;
}
@Override
public CallbackState withStateDataFilter(StateDataFilter stateDataFilter) {
super.withStateDataFilter(stateDataFilter);
return this;
}
@Override
public CallbackState withMetadata(Map metadata) {
super.withMetadata(metadata);
return this;
}
@Override
public CallbackState withTransition(Transition transition) {
super.withTransition(transition);
return this;
}
@Override
public CallbackState withDataInputSchema(java.lang.String dataInputSchema) {
super.withDataInputSchema(dataInputSchema);
return this;
}
@Override
public CallbackState withDataOutputSchema(java.lang.String dataOutputSchema) {
super.withDataOutputSchema(dataOutputSchema);
return this;
}
@Override
public CallbackState withOnErrors(List onErrors) {
super.withOnErrors(onErrors);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy