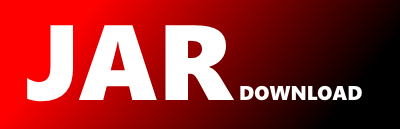
io.serverlessworkflow.api.auth.OauthDefinition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of serverlessworkflow-api Show documentation
Show all versions of serverlessworkflow-api Show documentation
Java SDK for Serverless Workflow Specification
package io.serverlessworkflow.api.auth;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.validation.Valid;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonValue;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"authority",
"grantType",
"clientId",
"clientSecret",
"scopes",
"username",
"password",
"audiences",
"subjectToken",
"requestedSubject",
"requestedIssuer",
"metadata"
})
public class OauthDefinition implements Serializable
{
/**
* String or a workflow expression. Contains the authority information
*
*/
@JsonProperty("authority")
@JsonPropertyDescription("String or a workflow expression. Contains the authority information")
@Size(min = 1)
private java.lang.String authority;
/**
* Defines the grant type
* (Required)
*
*/
@JsonProperty("grantType")
@JsonPropertyDescription("Defines the grant type")
@NotNull
private OauthDefinition.GrantType grantType;
/**
* String or a workflow expression. Contains the client identifier
* (Required)
*
*/
@JsonProperty("clientId")
@JsonPropertyDescription("String or a workflow expression. Contains the client identifier")
@Size(min = 1)
@NotNull
private java.lang.String clientId;
/**
* Workflow secret or a workflow expression. Contains the client secret
*
*/
@JsonProperty("clientSecret")
@JsonPropertyDescription("Workflow secret or a workflow expression. Contains the client secret")
@Size(min = 1)
private java.lang.String clientSecret;
/**
* Array containing strings or workflow expressions. Contains the OAuth2 scopes
*
*/
@JsonProperty("scopes")
@JsonPropertyDescription("Array containing strings or workflow expressions. Contains the OAuth2 scopes")
@Size(min = 1)
@Valid
private List scopes = new ArrayList();
/**
* String or a workflow expression. Contains the user name. Used only if grantType is 'resourceOwner'
*
*/
@JsonProperty("username")
@JsonPropertyDescription("String or a workflow expression. Contains the user name. Used only if grantType is 'resourceOwner'")
@Size(min = 1)
private java.lang.String username;
/**
* String or a workflow expression. Contains the user password. Used only if grantType is 'resourceOwner'
*
*/
@JsonProperty("password")
@JsonPropertyDescription("String or a workflow expression. Contains the user password. Used only if grantType is 'resourceOwner'")
@Size(min = 1)
private java.lang.String password;
/**
* Array containing strings or workflow expressions. Contains the OAuth2 audiences
*
*/
@JsonProperty("audiences")
@JsonPropertyDescription("Array containing strings or workflow expressions. Contains the OAuth2 audiences")
@Size(min = 1)
@Valid
private List audiences = new ArrayList();
/**
* String or a workflow expression. Contains the subject token
*
*/
@JsonProperty("subjectToken")
@JsonPropertyDescription("String or a workflow expression. Contains the subject token")
@Size(min = 1)
private java.lang.String subjectToken;
/**
* String or a workflow expression. Contains the requested subject
*
*/
@JsonProperty("requestedSubject")
@JsonPropertyDescription("String or a workflow expression. Contains the requested subject")
@Size(min = 1)
private java.lang.String requestedSubject;
/**
* String or a workflow expression. Contains the requested issuer
*
*/
@JsonProperty("requestedIssuer")
@JsonPropertyDescription("String or a workflow expression. Contains the requested issuer")
@Size(min = 1)
private java.lang.String requestedIssuer;
/**
* Metadata
*
*/
@JsonProperty("metadata")
@JsonPropertyDescription("Metadata")
@Valid
private Map metadata;
private final static long serialVersionUID = -1012409488046582753L;
/**
* No args constructor for use in serialization
*
*/
public OauthDefinition() {
}
/**
*
* @param clientId
* @param grantType
*/
public OauthDefinition(OauthDefinition.GrantType grantType, java.lang.String clientId) {
super();
this.grantType = grantType;
this.clientId = clientId;
}
/**
* String or a workflow expression. Contains the authority information
*
*/
@JsonProperty("authority")
public java.lang.String getAuthority() {
return authority;
}
/**
* String or a workflow expression. Contains the authority information
*
*/
@JsonProperty("authority")
public void setAuthority(java.lang.String authority) {
this.authority = authority;
}
public OauthDefinition withAuthority(java.lang.String authority) {
this.authority = authority;
return this;
}
/**
* Defines the grant type
* (Required)
*
*/
@JsonProperty("grantType")
public OauthDefinition.GrantType getGrantType() {
return grantType;
}
/**
* Defines the grant type
* (Required)
*
*/
@JsonProperty("grantType")
public void setGrantType(OauthDefinition.GrantType grantType) {
this.grantType = grantType;
}
public OauthDefinition withGrantType(OauthDefinition.GrantType grantType) {
this.grantType = grantType;
return this;
}
/**
* String or a workflow expression. Contains the client identifier
* (Required)
*
*/
@JsonProperty("clientId")
public java.lang.String getClientId() {
return clientId;
}
/**
* String or a workflow expression. Contains the client identifier
* (Required)
*
*/
@JsonProperty("clientId")
public void setClientId(java.lang.String clientId) {
this.clientId = clientId;
}
public OauthDefinition withClientId(java.lang.String clientId) {
this.clientId = clientId;
return this;
}
/**
* Workflow secret or a workflow expression. Contains the client secret
*
*/
@JsonProperty("clientSecret")
public java.lang.String getClientSecret() {
return clientSecret;
}
/**
* Workflow secret or a workflow expression. Contains the client secret
*
*/
@JsonProperty("clientSecret")
public void setClientSecret(java.lang.String clientSecret) {
this.clientSecret = clientSecret;
}
public OauthDefinition withClientSecret(java.lang.String clientSecret) {
this.clientSecret = clientSecret;
return this;
}
/**
* Array containing strings or workflow expressions. Contains the OAuth2 scopes
*
*/
@JsonProperty("scopes")
public List getScopes() {
return scopes;
}
/**
* Array containing strings or workflow expressions. Contains the OAuth2 scopes
*
*/
@JsonProperty("scopes")
public void setScopes(List scopes) {
this.scopes = scopes;
}
public OauthDefinition withScopes(List scopes) {
this.scopes = scopes;
return this;
}
/**
* String or a workflow expression. Contains the user name. Used only if grantType is 'resourceOwner'
*
*/
@JsonProperty("username")
public java.lang.String getUsername() {
return username;
}
/**
* String or a workflow expression. Contains the user name. Used only if grantType is 'resourceOwner'
*
*/
@JsonProperty("username")
public void setUsername(java.lang.String username) {
this.username = username;
}
public OauthDefinition withUsername(java.lang.String username) {
this.username = username;
return this;
}
/**
* String or a workflow expression. Contains the user password. Used only if grantType is 'resourceOwner'
*
*/
@JsonProperty("password")
public java.lang.String getPassword() {
return password;
}
/**
* String or a workflow expression. Contains the user password. Used only if grantType is 'resourceOwner'
*
*/
@JsonProperty("password")
public void setPassword(java.lang.String password) {
this.password = password;
}
public OauthDefinition withPassword(java.lang.String password) {
this.password = password;
return this;
}
/**
* Array containing strings or workflow expressions. Contains the OAuth2 audiences
*
*/
@JsonProperty("audiences")
public List getAudiences() {
return audiences;
}
/**
* Array containing strings or workflow expressions. Contains the OAuth2 audiences
*
*/
@JsonProperty("audiences")
public void setAudiences(List audiences) {
this.audiences = audiences;
}
public OauthDefinition withAudiences(List audiences) {
this.audiences = audiences;
return this;
}
/**
* String or a workflow expression. Contains the subject token
*
*/
@JsonProperty("subjectToken")
public java.lang.String getSubjectToken() {
return subjectToken;
}
/**
* String or a workflow expression. Contains the subject token
*
*/
@JsonProperty("subjectToken")
public void setSubjectToken(java.lang.String subjectToken) {
this.subjectToken = subjectToken;
}
public OauthDefinition withSubjectToken(java.lang.String subjectToken) {
this.subjectToken = subjectToken;
return this;
}
/**
* String or a workflow expression. Contains the requested subject
*
*/
@JsonProperty("requestedSubject")
public java.lang.String getRequestedSubject() {
return requestedSubject;
}
/**
* String or a workflow expression. Contains the requested subject
*
*/
@JsonProperty("requestedSubject")
public void setRequestedSubject(java.lang.String requestedSubject) {
this.requestedSubject = requestedSubject;
}
public OauthDefinition withRequestedSubject(java.lang.String requestedSubject) {
this.requestedSubject = requestedSubject;
return this;
}
/**
* String or a workflow expression. Contains the requested issuer
*
*/
@JsonProperty("requestedIssuer")
public java.lang.String getRequestedIssuer() {
return requestedIssuer;
}
/**
* String or a workflow expression. Contains the requested issuer
*
*/
@JsonProperty("requestedIssuer")
public void setRequestedIssuer(java.lang.String requestedIssuer) {
this.requestedIssuer = requestedIssuer;
}
public OauthDefinition withRequestedIssuer(java.lang.String requestedIssuer) {
this.requestedIssuer = requestedIssuer;
return this;
}
/**
* Metadata
*
*/
@JsonProperty("metadata")
public Map getMetadata() {
return metadata;
}
/**
* Metadata
*
*/
@JsonProperty("metadata")
public void setMetadata(Map metadata) {
this.metadata = metadata;
}
public OauthDefinition withMetadata(Map metadata) {
this.metadata = metadata;
return this;
}
public enum GrantType {
PASSWORD("password"),
CLIENT_CREDENTIALS("clientCredentials"),
TOKEN_EXCHANGE("tokenExchange");
private final java.lang.String value;
private final static Map CONSTANTS = new HashMap();
static {
for (OauthDefinition.GrantType c: values()) {
CONSTANTS.put(c.value, c);
}
}
private GrantType(java.lang.String value) {
this.value = value;
}
@Override
public java.lang.String toString() {
return this.value;
}
@JsonValue
public java.lang.String value() {
return this.value;
}
@JsonCreator
public static OauthDefinition.GrantType fromValue(java.lang.String value) {
OauthDefinition.GrantType constant = CONSTANTS.get(value);
if (constant == null) {
throw new IllegalArgumentException(value);
} else {
return constant;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy