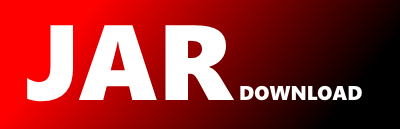
io.serverlessworkflow.api.functions.FunctionRef Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of serverlessworkflow-api Show documentation
Show all versions of serverlessworkflow-api Show documentation
Java SDK for Serverless Workflow Specification
package io.serverlessworkflow.api.functions;
import java.io.Serializable;
import java.util.HashMap;
import java.util.Map;
import javax.validation.Valid;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.databind.JsonNode;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"refName",
"arguments",
"selectionSet",
"invoke"
})
public class FunctionRef implements Serializable
{
/**
* Name of the referenced function
* (Required)
*
*/
@JsonProperty("refName")
@JsonPropertyDescription("Name of the referenced function")
@Size(min = 1)
@NotNull
private String refName;
/**
* Function arguments
*
*/
@JsonProperty("arguments")
@JsonPropertyDescription("Function arguments")
@Valid
private JsonNode arguments;
/**
* Only used if function type is 'graphql'. A string containing a valid GraphQL selection set
*
*/
@JsonProperty("selectionSet")
@JsonPropertyDescription("Only used if function type is 'graphql'. A string containing a valid GraphQL selection set")
private String selectionSet;
/**
* Specifies if the function should be invoked sync or async. Default is sync.
*
*/
@JsonProperty("invoke")
@JsonPropertyDescription("Specifies if the function should be invoked sync or async. Default is sync.")
private FunctionRef.Invoke invoke = FunctionRef.Invoke.fromValue("sync");
private final static long serialVersionUID = -194299573266930319L;
/**
* No args constructor for use in serialization
*
*/
public FunctionRef() {
}
/**
*
* @param refName
*/
public FunctionRef(String refName) {
super();
this.refName = refName;
}
/**
* Name of the referenced function
* (Required)
*
*/
@JsonProperty("refName")
public String getRefName() {
return refName;
}
/**
* Name of the referenced function
* (Required)
*
*/
@JsonProperty("refName")
public void setRefName(String refName) {
this.refName = refName;
}
public FunctionRef withRefName(String refName) {
this.refName = refName;
return this;
}
/**
* Function arguments
*
*/
@JsonProperty("arguments")
public JsonNode getArguments() {
return arguments;
}
/**
* Function arguments
*
*/
@JsonProperty("arguments")
public void setArguments(JsonNode arguments) {
this.arguments = arguments;
}
public FunctionRef withArguments(JsonNode arguments) {
this.arguments = arguments;
return this;
}
/**
* Only used if function type is 'graphql'. A string containing a valid GraphQL selection set
*
*/
@JsonProperty("selectionSet")
public String getSelectionSet() {
return selectionSet;
}
/**
* Only used if function type is 'graphql'. A string containing a valid GraphQL selection set
*
*/
@JsonProperty("selectionSet")
public void setSelectionSet(String selectionSet) {
this.selectionSet = selectionSet;
}
public FunctionRef withSelectionSet(String selectionSet) {
this.selectionSet = selectionSet;
return this;
}
/**
* Specifies if the function should be invoked sync or async. Default is sync.
*
*/
@JsonProperty("invoke")
public FunctionRef.Invoke getInvoke() {
return invoke;
}
/**
* Specifies if the function should be invoked sync or async. Default is sync.
*
*/
@JsonProperty("invoke")
public void setInvoke(FunctionRef.Invoke invoke) {
this.invoke = invoke;
}
public FunctionRef withInvoke(FunctionRef.Invoke invoke) {
this.invoke = invoke;
return this;
}
public enum Invoke {
SYNC("sync"),
ASYNC("async");
private final String value;
private final static Map CONSTANTS = new HashMap();
static {
for (FunctionRef.Invoke c: values()) {
CONSTANTS.put(c.value, c);
}
}
private Invoke(String value) {
this.value = value;
}
@Override
public String toString() {
return this.value;
}
@JsonValue
public String value() {
return this.value;
}
@JsonCreator
public static FunctionRef.Invoke fromValue(String value) {
FunctionRef.Invoke constant = CONSTANTS.get(value);
if (constant == null) {
throw new IllegalArgumentException(value);
} else {
return constant;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy