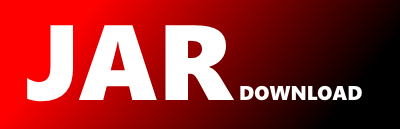
io.serverlessworkflow.api.functions.SubFlowRef Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of serverlessworkflow-api Show documentation
Show all versions of serverlessworkflow-api Show documentation
Java SDK for Serverless Workflow Specification
package io.serverlessworkflow.api.functions;
import java.io.Serializable;
import java.util.HashMap;
import java.util.Map;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonValue;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"workflowId",
"version",
"onParentComplete",
"invoke"
})
public class SubFlowRef implements Serializable
{
/**
* Unique id of the sub-workflow to be invoked
* (Required)
*
*/
@JsonProperty("workflowId")
@JsonPropertyDescription("Unique id of the sub-workflow to be invoked")
@NotNull
private String workflowId;
/**
* Version of the sub-workflow to be invoked
*
*/
@JsonProperty("version")
@JsonPropertyDescription("Version of the sub-workflow to be invoked")
@Size(min = 1)
private String version;
/**
* If invoke is 'async', specifies how subflow execution should behave when parent workflow completes. Default is 'terminate'
*
*/
@JsonProperty("onParentComplete")
@JsonPropertyDescription("If invoke is 'async', specifies how subflow execution should behave when parent workflow completes. Default is 'terminate'")
private SubFlowRef.OnParentComplete onParentComplete = SubFlowRef.OnParentComplete.fromValue("terminate");
/**
* Specifies if the function should be invoked sync or async. Default is sync.
*
*/
@JsonProperty("invoke")
@JsonPropertyDescription("Specifies if the function should be invoked sync or async. Default is sync.")
private SubFlowRef.Invoke invoke = SubFlowRef.Invoke.fromValue("sync");
private final static long serialVersionUID = 924910219419591889L;
/**
* No args constructor for use in serialization
*
*/
public SubFlowRef() {
}
/**
*
* @param workflowId
*/
public SubFlowRef(String workflowId) {
super();
this.workflowId = workflowId;
}
/**
* Unique id of the sub-workflow to be invoked
* (Required)
*
*/
@JsonProperty("workflowId")
public String getWorkflowId() {
return workflowId;
}
/**
* Unique id of the sub-workflow to be invoked
* (Required)
*
*/
@JsonProperty("workflowId")
public void setWorkflowId(String workflowId) {
this.workflowId = workflowId;
}
public SubFlowRef withWorkflowId(String workflowId) {
this.workflowId = workflowId;
return this;
}
/**
* Version of the sub-workflow to be invoked
*
*/
@JsonProperty("version")
public String getVersion() {
return version;
}
/**
* Version of the sub-workflow to be invoked
*
*/
@JsonProperty("version")
public void setVersion(String version) {
this.version = version;
}
public SubFlowRef withVersion(String version) {
this.version = version;
return this;
}
/**
* If invoke is 'async', specifies how subflow execution should behave when parent workflow completes. Default is 'terminate'
*
*/
@JsonProperty("onParentComplete")
public SubFlowRef.OnParentComplete getOnParentComplete() {
return onParentComplete;
}
/**
* If invoke is 'async', specifies how subflow execution should behave when parent workflow completes. Default is 'terminate'
*
*/
@JsonProperty("onParentComplete")
public void setOnParentComplete(SubFlowRef.OnParentComplete onParentComplete) {
this.onParentComplete = onParentComplete;
}
public SubFlowRef withOnParentComplete(SubFlowRef.OnParentComplete onParentComplete) {
this.onParentComplete = onParentComplete;
return this;
}
/**
* Specifies if the function should be invoked sync or async. Default is sync.
*
*/
@JsonProperty("invoke")
public SubFlowRef.Invoke getInvoke() {
return invoke;
}
/**
* Specifies if the function should be invoked sync or async. Default is sync.
*
*/
@JsonProperty("invoke")
public void setInvoke(SubFlowRef.Invoke invoke) {
this.invoke = invoke;
}
public SubFlowRef withInvoke(SubFlowRef.Invoke invoke) {
this.invoke = invoke;
return this;
}
public enum Invoke {
SYNC("sync"),
ASYNC("async");
private final String value;
private final static Map CONSTANTS = new HashMap();
static {
for (SubFlowRef.Invoke c: values()) {
CONSTANTS.put(c.value, c);
}
}
private Invoke(String value) {
this.value = value;
}
@Override
public String toString() {
return this.value;
}
@JsonValue
public String value() {
return this.value;
}
@JsonCreator
public static SubFlowRef.Invoke fromValue(String value) {
SubFlowRef.Invoke constant = CONSTANTS.get(value);
if (constant == null) {
throw new IllegalArgumentException(value);
} else {
return constant;
}
}
}
public enum OnParentComplete {
CONTINUE("continue"),
TERMINATE("terminate");
private final String value;
private final static Map CONSTANTS = new HashMap();
static {
for (SubFlowRef.OnParentComplete c: values()) {
CONSTANTS.put(c.value, c);
}
}
private OnParentComplete(String value) {
this.value = value;
}
@Override
public String toString() {
return this.value;
}
@JsonValue
public String value() {
return this.value;
}
@JsonCreator
public static SubFlowRef.OnParentComplete fromValue(String value) {
SubFlowRef.OnParentComplete constant = CONSTANTS.get(value);
if (constant == null) {
throw new IllegalArgumentException(value);
} else {
return constant;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy